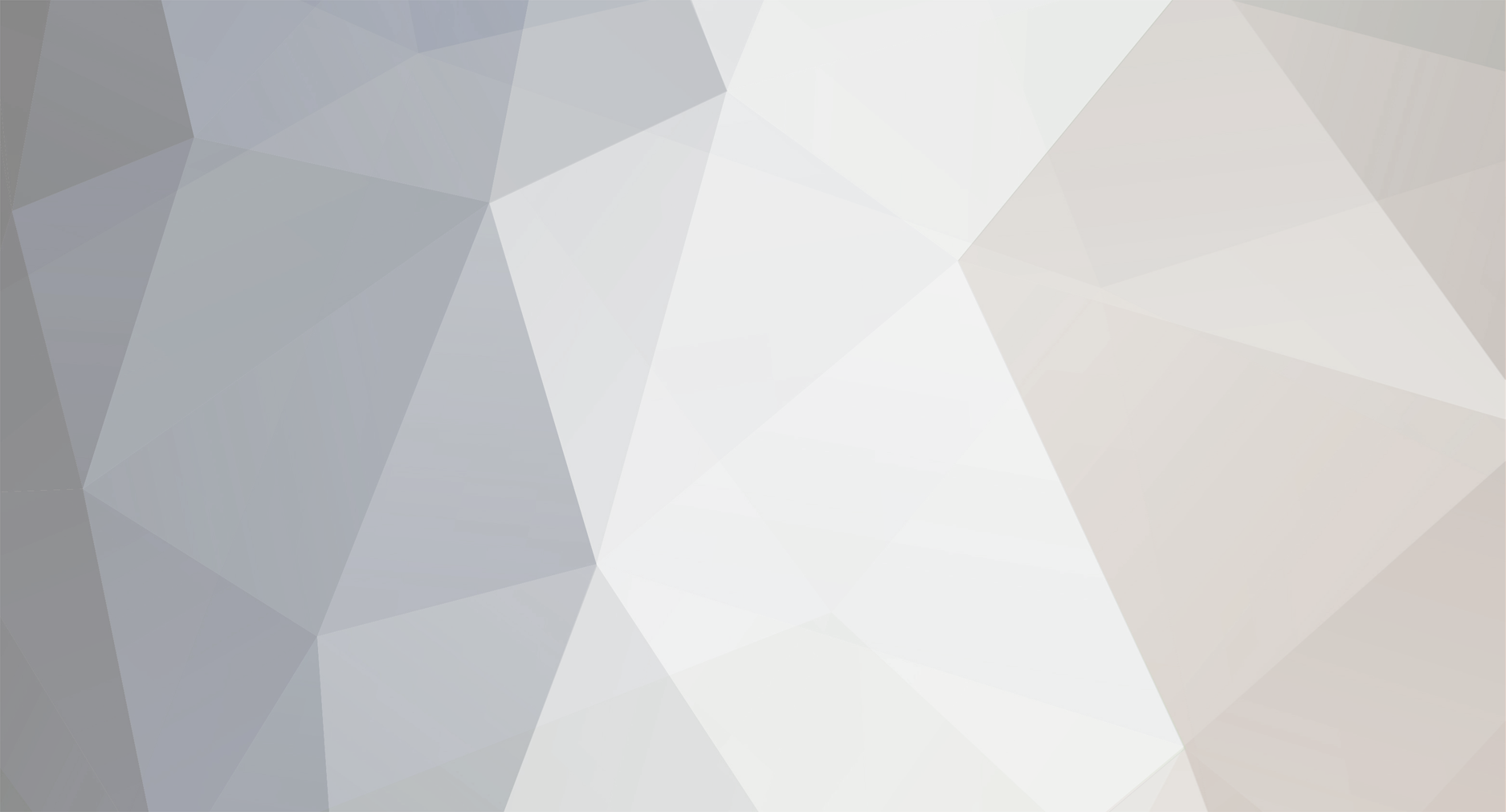
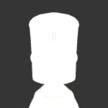
EricccMa
Members-
Posts
3 -
Joined
-
Last visited
Reputation
1 NeutralRecent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
-
Thank you for reviewing my code. I try to change my code as you suggest. Unfortunately, throttle still doesn't work. These days, I refactor my code and here is my code. KPanel.ino #include <KerbalSimpit.h> #include <Arduino.h> #include <PayloadStructs.h> #include <Wire.h> #include <SPI.h> #include <U8g2lib.h> #include "Util.h" #define RC_PIN 2 U8G2_SSD1306_128X64_NONAME_1_HW_I2C lcd(U8G2_R0, /* reset=*/ U8X8_PIN_NONE); //init u8g2 driver // How often to send echo packets (in ms) unsigned int sendInterval = 100; void initHandler() { lcd.firstPage(); do { lcd.setCursor(0,20); lcd.print("Connecting"); }while(lcd.nextPage()); } void setup() { lcd.begin(); lcd.setFont(u8g2_font_ncenB14_tr); Serial.begin(115200); initSimpit(Serial,RC_PIN); initHandler(); startSimpit(); } void loop() { static unsigned long lastLoop = millis(); lcd.firstPage(); do { if(receiveFlag) { lcd.setCursor(0,20); if(mannualMode) { lcd.print("Manual"); }else { lcd.print("Auto"); } lcd.setCursor(0,40); lcd.print("ALT:"); lcd.print(altitude.surface); }else { lcd.setCursor(0,40); lcd.print("Wait Data"); } }while(lcd.nextPage()); unsigned long now = millis(); unsigned long delta = now - lastLoop; if(delta>=sendInterval) { sendRC(); } checkRecv(); } Utils.h #ifndef UTIL_H #define UTIL_H #include <Arduino.h> #include <HardwareSerial.h> #include <KerbalSimpit.h> #include <PayloadStructs.h> extern KerbalSimpit* mySimpit;//KerbalSimpit extern bool receiveFlag;//true if is in flight extern int RC[10]; extern int RC_Parsed[4]; extern altitudeMessage altitude; extern int rcPin; extern unsigned long lastRecvTime; extern bool mannualMode; //current mode: true:mannual false:auto void initSimpit(Stream& in,int rc); void startSimpit(); void checkRecv(); void sendRC(); void parseRC(); void InterruptService(); void messageHandler(byte messageType, byte msg[], byte msgSize); #endif Utils.cpp #include "Util.h" KerbalSimpit* mySimpit;//KerbalSimpit bool receiveFlag = false;//true if is in flight int RC[10]={0}; int RC_Parsed[4]={0}; int rcPin; altitudeMessage altitude; unsigned long lastRecvTime=0; bool mannualMode = true; void initSimpit(Stream& in,int rc) { mySimpit = new KerbalSimpit(in); rcPin = rc; attachInterrupt(digitalPinToInterrupt(rcPin),InterruptService,CHANGE); } void startSimpit() { // This loop continually attempts to handshake with the plugin. // It will keep retrying until it gets a successful handshake. while (!mySimpit->init()) { delay(100); } lastRecvTime = millis(); mySimpit->registerChannel(ALTITUDE_MESSAGE); mySimpit->inboundHandler(messageHandler); } void checkRecv() { mySimpit->update(); unsigned long delta = millis() - lastRecvTime; if(delta>1000) { receiveFlag = false; }else { receiveFlag = true; } } void sendRC() { parseRC(); rotationMessage rotationMsg; rotationMsg.mask=1|2|4; if(RC[4]>1500)//Manual { mannualMode = true; rotationMsg.roll=RC_Parsed[0]; rotationMsg.pitch=RC_Parsed[1]; rotationMsg.yaw=RC_Parsed[3]; }else { mannualMode = false; rotationMsg.roll=0; rotationMsg.pitch=0; rotationMsg.yaw=0; } mySimpit->send(ROTATION_MESSAGE,rotationMsg); mySimpit->send(THROTTLE_MESSAGE,(unsigned char*)(&RC_Parsed[2]),2); } void InterruptService() { static unsigned long int lastTime=0; static int cursor=0; if (digitalRead(2) == LOW) { unsigned long int now=micros(); unsigned long int thisTime=now-lastTime; if(thisTime>3000) { cursor=0; }else { RC[cursor++]=thisTime; } lastTime=now; } } void parseRC() { for(int i=0;i<4;i++) { RC_Parsed[i]=map(RC[i],1000,2000,-31000,31000); } RC_Parsed[2] +=31000; } void messageHandler(byte messageType, byte msg[], byte msgSize) { static bool ledStatus = false; digitalWrite(13,ledStatus); ledStatus = !ledStatus; if(messageType == ALTITUDE_MESSAGE) { altitude=parseAltitude(msg); } lastRecvTime = millis(); } I can make sure it sends throttle data, but it still doesn't work. Can you help me find the solution? Hope for replying soon. Thanks
-
Of course Ok. It's just a simple code modified from example. Here is the code. /* KerbalSimpitHelloWorld A very barebones Hello World sketch, that doesn't require any extra hardware. It periodically sends EchoRequest packets to the game, and uses the EchoReply packets to switch the on-board LED on and off. Note that the game only activates the echo handler during the flight scene. Great for confirming basic connectivity. Peter Hardy <[email protected]> */ #include "KerbalSimpit.h" #include <Arduino.h> #include <LiquidCrystal.h> #include <PayloadStructs.h> // Declare a KerbalSimpit object that will // communicate using the "Serial" device. KerbalSimpit mySimpit(Serial); // This boolean tracks the desired LED state. bool state = false; const int rs = 12, en = 11, d4 = 5, d5 = 4, d6 = 3, d7 = 6; LiquidCrystal lcd(rs, en, d4, d5, d6, d7); altitudeMessage altitude; // A timestamp of the last time we sent an echo packet. unsigned long lastSent = 0; // How often to send echo packets (in ms) unsigned int sendInterval = 100; int firstTimeFlag=1; int receivedFlag=0; void messageHandler(byte messageType, byte msg[], byte msgSize) { // We are only interested in echo response packets. if (messageType == ECHO_RESP_MESSAGE) { // The message payload will be either "low" or "high". // We use the strcmp function to check what the string payload // is, and set the LED status accordingly. if (msg[0]=='l') { digitalWrite(LED_BUILTIN, LOW); } else { digitalWrite(LED_BUILTIN, HIGH); } } if(messageType == ALTITUDE_MESSAGE) { altitude=parseAltitude(msg); if(firstTimeFlag) { lcd.clear(); lcd.print("R-ALT"); firstTimeFlag=0; } lcd.setCursor(0,1); lcd.print(altitude.surface); receivedFlag=1; } if(messageType==SCENE_CHANGE_MESSAGE) { firstTimeFlag=1; receivedFlag=0; altitude.surface=0; } } int RC1[10]={1506,1506,1506,1506,1506,1506,1400}; int16_t RC[4]={0}; void parseRC() { for(int i=0;i<4;i++) { RC[i]=map(RC1[i],1100,1900,-97,97); RC[i]=map(RC[i],-97,97,-31000,31000); } } void InterruptService() { static unsigned long int lastTime=0; static int cursor=0; if (digitalRead(2) == LOW) { unsigned long int now=micros(); unsigned long int thisTime=now-lastTime; if(thisTime>3000) { cursor=0; }else { RC1[cursor++]=thisTime; } lastTime=now; } } void setup() { // Open the serial connection. pinMode(2,INPUT); attachInterrupt(0,InterruptService,CHANGE); Serial.begin(115200); lcd.begin(16, 2); // Print a message to the LCD. lcd.print("Connecting..."); // Set up the built in LED, and turn it on. pinMode(LED_BUILTIN, OUTPUT); digitalWrite(LED_BUILTIN, HIGH); // This loop continually attempts to handshake with the plugin. // It will keep retrying until it gets a successful handshake. while (!mySimpit.init()) { delay(100); } // Turn off the built-in LED to indicate handshaking is complete. digitalWrite(LED_BUILTIN, LOW); mySimpit.registerChannel(ALTITUDE_MESSAGE); mySimpit.registerChannel(SCENE_CHANGE_MESSAGE); lcd.clear(); lcd.print(" #Connected#"); lcd.setCursor(0,1); lcd.print("Waiting Data..."); // Sets our callback function. The KerbalSimpit library will // call this function every time a packet is received. mySimpit.inboundHandler(messageHandler); } void loop() { unsigned long now = millis(); // If we've waited long enough since the last message, send a new one. if (now - lastSent >= sendInterval) { if(receivedFlag) { parseRC(); rotationMessage rotationMsg; rotationMsg.mask=1|2|4; if(RC1[4]>1500)//Manual { rotationMsg.roll=RC[0]; rotationMsg.pitch=RC[1]; rotationMsg.yaw=RC[3]; lcd.setCursor(11,0); lcd.write("MAN "); }else { rotationMsg.roll=0; rotationMsg.pitch=0; rotationMsg.yaw=0; lcd.setCursor(11,0); lcd.write("AUTO"); } mySimpit.send(ROTATION_MESSAGE,rotationMsg); RC[2]+=3000; RC[2]=RC[2]<0?0:RC[2]; RC[2]=map(RC[2],0,6000,0,1000); mySimpit.send(THROTTLE_MESSAGE,(unsigned char*)(&RC[2]),2); }else { lcd.setCursor(0,0); lcd.print(" #Connected# "); lcd.setCursor(0,1); lcd.print("Waiting Data..."); } } // Call the library update() function to check for new messages. mySimpit.update(); } here mySimpit.send(ROTATION_MESSAGE,rotationMsg); RC[2]+=3000; RC[2]=RC[2]<0?0:RC[2]; RC[2]=map(RC[2],0,6000,0,1000); mySimpit.send(THROTTLE_MESSAGE,(unsigned char*)(&RC[2]),2); }else Everything works well except the throttle. I just test on KSP1.3.1. About KSP 1.4, I haven't test because have no radio controller this time.
-
Excuse me,I try sending the THROTTLE_MESSAGE(19),but it doesn't work. At the same time ,rotation message works well. I used the func send(19,data,2) . My KSP Version is 1.3.1.1891. And I used a arduino nano and a crystal Liquid to decode the PPM signal and send the control message by serial and show attitude on screen. Everything worked well except the throttle. Is there any solutions?