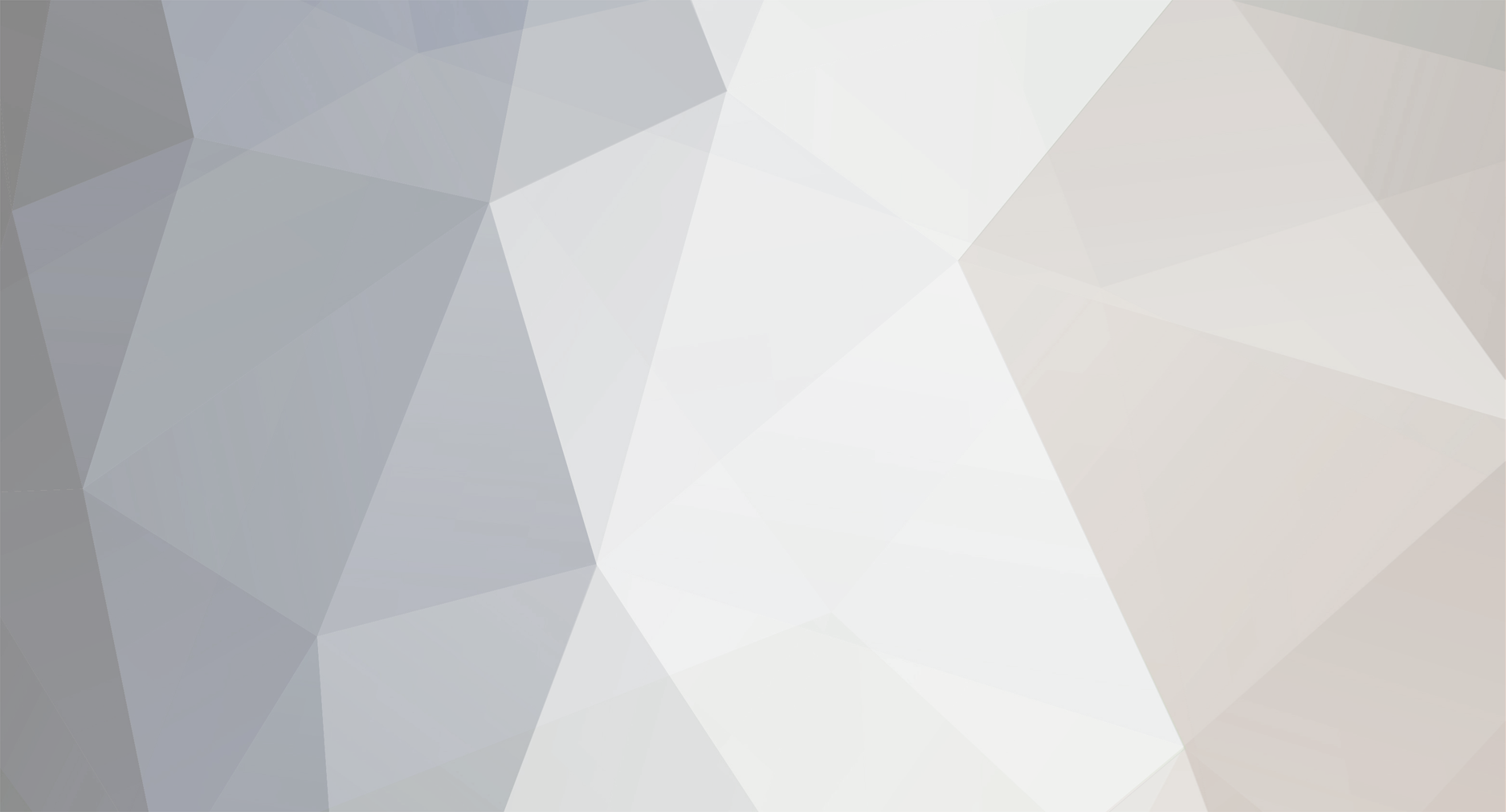
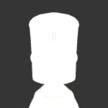
Idranel
Members-
Posts
8 -
Joined
-
Last visited
Content Type
Profiles
Forums
Developer Articles
KSP2 Release Notes
Bug Reports
Everything posted by Idranel
-
[1.3] kOS Scriptable Autopilot System v1.1.3.0
Idranel replied to erendrake's topic in KSP1 Mod Releases
Yes this is assumed here. Picking two vectors spanning such a plane more or less arbitrarily works well enough if things are mostly flat. For anything hilly (the question remains whether or not KSP terrain actually gets bumpy enough) you'll probably want a higher resolution. But admittedly it might not be worth going through the trouble considering how slow it'll be in comparison. Fortunately in native code this stuff is fast enough to be useful. -
[1.3] kOS Scriptable Autopilot System v1.1.3.0
Idranel replied to erendrake's topic in KSP1 Mod Releases
This method works for arbitrary point cloud neighborhoods without prior knowledge of their structure. Its commonly used to compute normals in 3D laser scan data. If you're limited to three points then the vector cross product makes more sense. -
[1.3] kOS Scriptable Autopilot System v1.1.3.0
Idranel replied to erendrake's topic in KSP1 Mod Releases
Have you ever wished for a method of measuring slopes within line of sight without flying over the spot or derriving samples of ground altitude measurements ? Fortunately you can do just that using the LaserDist mod, kOS and some math. Mount a number of laser distance sensors (using IR for rotation if desired) in such a way that you have one central laser beam and a number of other beams in a cone or pyramid like fashion. Optional: Designate one (origin-)part of your vessel to be used as the origin of your local coordinate system. Using part:FACING:INVERSE you can transform the orientation of the sensors into something sane for your vessel's frame of reference. Collect distance measurements and calculate the vector from origin to where the beam hit the ground. (Problem: its unclear/undocumented where exactly the beams originate from in relation to the pose of the sensor part) Compute Covariance Matrix Z (3x3) as follows: Let p0 be the point where the central beam hits and pj ; j > 0 the other points. Z := 1/(number of sensors) * SUMi [( pi - p0) * (pi - p0)T] Invert Z using the general formula for 3x3 matrix inversion ( see https://ardoris.wordpress.com/2008/07/18/general-formula-for-the-inverse-of-a-3x3-matrix/ or http://mathworld.wolfram.com/MatrixInverse.html ) Compute the eigenvector v corresponding to the most significant eigenvalue l of Z-1 using the power method. This is the eigenvector corresponding to the least signficant eigenvalue of Z. initialize v with something other than 0 iterate vk+1 = (Z-1 * vk) / ||(Z-1 * vk)|| until value hasn't changed much [*]This vector can either point towards the sky or the ground. If that matters then check which case it is and flip it around as needed. [*]You can now compare the normal vector with vector from central point p0 to the center of the body (direction of gravity at that point) you're orbiting. The resulting eigenvector is the one corresponding to the least signficant eigenvalue of Z. Z describes how the points are distributed around p0 and its Eigenvectors describe a new orthogonal base where each vector is chosen so that the variance (of the points) along its direction is maximized (but each additional vector is orthogonal to the previous ones). ( See http://en.wikipedia.org/wiki/Principal_component_analysis too). The vector orthogonal to two (orthogonal) vectors that maximize the variance is an approximation of the normal in the center point provided that the other points are reasonably close together and provide enough resolution when sampling the surface. So far I've implemented all necessary matrix operations (on lists) but given the non existance of variable scopes and return values actually reusing code is a complete nightmare with KOS. It always turns into frustrating spaghetti. Given that the type system is weak sauce too its almost tempting to try mod a Python interpreter into KSP instead. -
[1.3] kOS Scriptable Autopilot System v1.1.3.0
Idranel replied to erendrake's topic in KSP1 Mod Releases
Here is a little feature request: One of my SSTO designs (using FAR) requires variable flap settings based on air speed measured in mach numbers. It would be nice to have a function returning the speed of sound for a vessel's location. -
GPL licenses are highly annoying as they require derivative works also to be licensed under GPL. Especially version 3 can be a pain in the butt. But what exactly derivative means when one just links to a gpl'ed library can be pretty fuzzy and unclear. Sometimes I wish game developers would force modders into using BSD/MIT licenses simply to avoid drama and headaches.
-
1) Get your current altitude and subtract the altitude of where you want to start turning. If its negative go straight up instead. 2) Choose an altitude where you want to stop turning and subtract the altitude where you start, then divide the value from 1) by that number. Think of something clever for when you're above the turn end that doesnt cause bugs. 3) Vessel specific: Figure out some function that maps from [0,1] -> [90,0] for your pitch angle and use it on the value from 2) You could try a similiar function of altitude to define a target speed or thrust setting To prevent the angle of attack getting out of hand compute the angle between your prograde vector and the vector you want to lock onto. If that exceeds some safety limit then only change steering up to that point.
-
Here is a little script that extrapolates a planes vertical speed and tries to converge the altitude to some target altitude. Testing it on a B9 I8-L Bradbury it was able to keep it stable within 2.5 meters of the target (11500m). On heavier designs like the B9 D-175 Strugatsky it struggled to keep within a couple 100m as there is just too much momentum and not enough pitch authority at that altitude. Random question: What exactly is the semantic of locking variables ? When exactly are they updated ? set targetAlt to 11500. set targetHeading to 90. set vMinPitch to -10. set vMaxPitch to 15. set vPitchDownSpeed to 0.05. set vPitchUpSpeed to 0.05. set predictionTime to 120. set newPitch to 10. set vhPrev to 0. set vhDev1Prev to 0. set vhDev1 to 0. set vhDev2 to 0. set dampening to 0.95. CLEARSCREEN. until 0 { set vh to VERTICALSPEED. set vhDev1 to (VERTICALSPEED - vhPrev) * (1-dampening) + dampening * vhDev1. set vhDev2 to (vhDev1 - vhDev1Prev) * (1-dampening) + dampening * vhDev2. declare vPitchAdjustment. //set predictedAltitude to ALTITUDE + (vh + ((vhDev1 + vhDev2 * predictionTime / 2 ) * predictionTime / 2)) * predictionTime. set predictedAltitude to ALTITUDE + (vh + (vhDev1 * predictionTime / 2)) * predictionTime. set altitudeGap to targetAlt - predictedAltitude. set pitchSpeedModifier to altitudeGap / 500. if altitudeGap > 0.5 { set vPitchAdjustment to vPitchUpSpeed * pitchSpeedModifier. }. if altitudeGap < 0.5 { set vPitchAdjustment to vPitchDownSpeed * pitchSpeedModifier. }. set newPitch to newPitch + vPitchAdjustment. if newPitch < vMinPitch { set newPitch to vMinPitch. }. if newPitch > vMaxPitch { set newPitch to vMaxPitch. }. lock steering to heading targetHeading by newPitch. print " Surface Speed: " + SURFACESPEED at (0,1). print " Vertical Speed: " + vh at (0,2). print " delta Vertical Speed: " + vhDev1 at (0,3). print "delta^2 Vertical Speed: " + vhDev2 at (0,4). print " Prediction Window: " + predictionTime at (0,5). print " Altitude: " + ALTITUDE at (0,6). print " Predicted Altitude: " + predictedAltitude at (0,7). print " Pitch Change: " + vPitchAdjustment at (0,8). print " Pitch Target: " + newPitch at (0,9). print " Heading Target: " + targetHeading at (0,10). set vhDev1Prev to vhDev1. set vhPrev to vh. }. BTW: Some random things I wish for min/max functions or maybe even a clamping function. arrays (associative or vanilla) so that its easier to store flight data, sensor readings ect. While its possible to produce a graph with print .. at it would be nice to also have that data readily available for further computations.
-
Some random thoughts: The editor terminates with an error when the legth of a line exceeds the terminal window. The Font is difficult to read, stuff like < and { or ( should be easier to distinguish. Some useful math functionality like logarithms is missing. It would be nice to have else or even elseif cases for if statements. A while loop would be nice, so that its easier to choose between checking the conditions before or after each loop body execution. Apparantly some people use sub-programs and I assume that they share variables, but it would be really nice to have proper functions with parameters and return values. The documentation is so terrible that it overshadows what is accomplished with this mod. It seems to be impossible to determine basic stuff like the attitude of your craft, especially pitch. Various people asked for it three atleast three times in this thread without getting an answer and its not documented anywhere. While its a very nice mod I don't see it going anywhere until decent documentation is available.