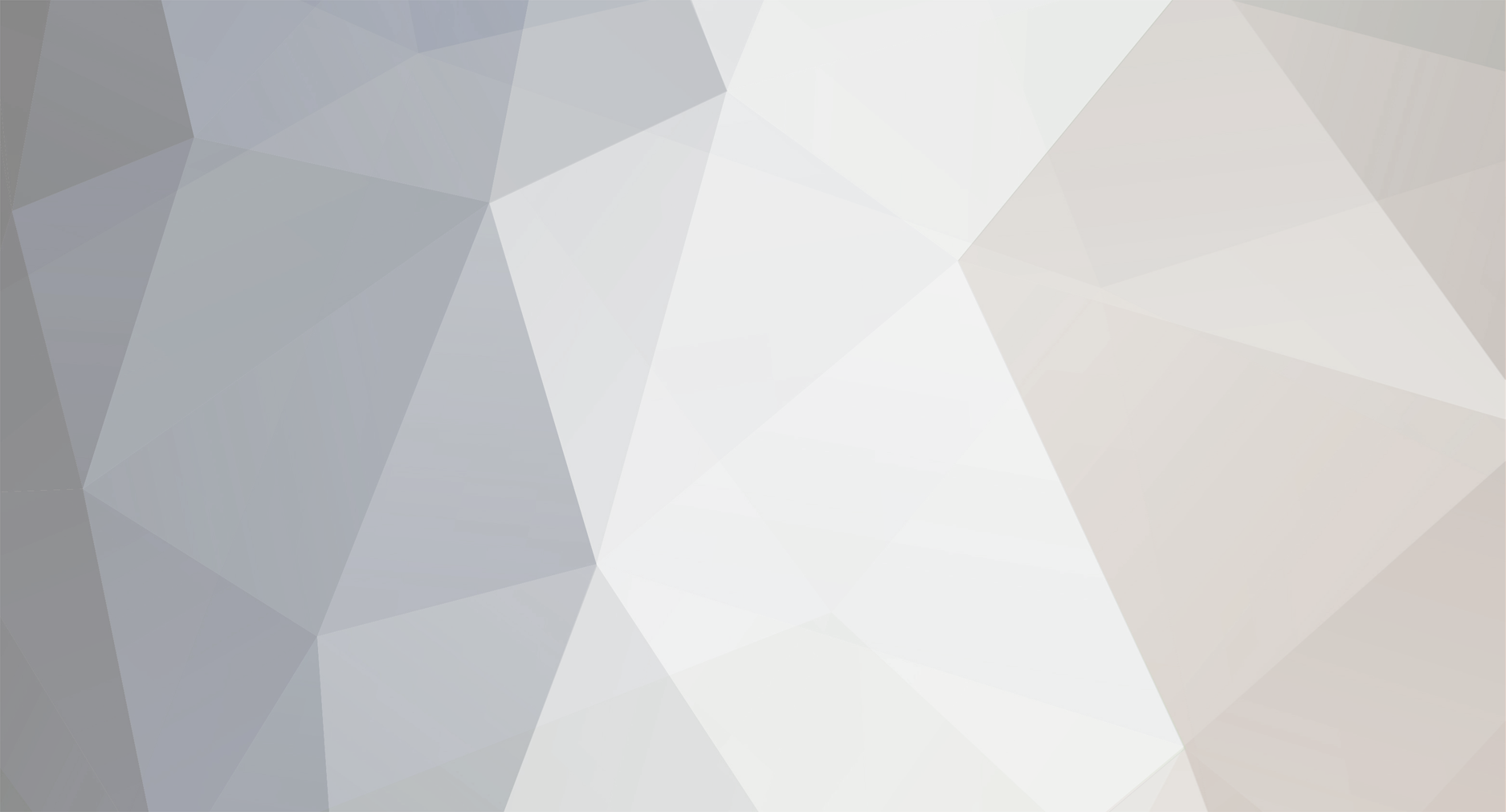
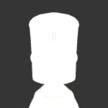
JellyCubes
-
Posts
141 -
Joined
-
Last visited
Content Type
Profiles
Forums
Developer Articles
KSP2 Release Notes
Bug Reports
Posts posted by JellyCubes
-
-
No words can describe how amazing this is.
[move]<3 <3 <3 <3[/move]
A question: a conventional object will explode if the ground comes within 0.2 m of the node_collider origin. Do the same thing happen to the cart wheels?
-
So, is this the final version???
Currently spending most of my time messing around with the plugin system so changing it isn\'t high on my priority list.
Sorry if I sound like a idiot, but what would the instructions be for a Munar orbit at 5KM going over the poles? I\'m not good at the whole 'Eccentricity' thing.....
Semi-major axis = 205000
Eccentricity = 0
Inclination = 90 or 270
-
It\'s possible to collide with them but the main problem is that the collider is limited to 256 faces and it\'s the collider that determines what you can collide with.
The asteroid has 1000 faces so the collider only approximates the shape of the asteroid. The collider might be above the visible surface in one area and below the visible surface in another area.
-
I had around 40 units of fuel left at the end.
3.5 tanks is probably reaching the limit for Mun landings. 3 tanks might be possible everything would have to be done perfectly. May be a flight computer module could do it.
-
Put the program in the KSP save file and run it.
Without any kind of in-depth tracking, it\'s very difficult to intercept an asteroid in Kerbol orbit. Landing isn\'t really possible either since the asteroid surface and the collider don\'t match up.
-
Landed on the Mun and returned safely* with 3.5 tanks. *Landed on a hill so constant control imput is required to prevent toppling over and save-scummed once because time warp did not unwarp quickly enough.
No landing legs, no RCS, no SAS and no parachute.
-
So if you try this when you have 2 ships with the same name, will they just translate up to the new orbit height, without changing their position on the orbit, or will they both occupy the same point, and therefore destroy each other, or C, none of the above? I dont know, i havent tried it (I have a mac, and want to wait a little before i try it out)..
All the ships are moved to the chosen orbit. It does not change the position along the orbit.
-
I could compile a mac version for you.
This would be nice.
New script in the works; an asteroid field generator.
Lulz.
-
[ quote author=Gojira link=topic=7844.msg115619#msg115619 date=1330701392]
Mac version please?
ignore that
but really, will there be a mac version?
Sorry, but I neither own a Mac or know how to cross-compile.
-
[list type=decimal]
[li]Put the Instant Orbit program in the /saves/default folder in Kerbal Space Program.[/li]
[li]Launch a ship in KSP and immediately return to the Space Center (don\'t end the flight). The ship should still be on the launchpad. Check the Flight Tracking building to confirm.[/li]
[li]If KSP is still open, return to the main Space Center scene and then open the Instant Orbit program.[/li]
[li]Enter the name of the ship that was just launched and then follow the prompts.[/li]
Step 2 is only required if you want to put something directly into orbit. Ships that are already in orbit and have their orbits changed.
All ships with the same name will have their orbits changed.
-
Added a railgun version that works with 0.14. Ships now experience recoil when firing. The recoil force may force rip the railgun off your ship.
Ideally, I would like to create a railgun that spawns railgun shells so you don\'t have to manually add them in the VAB and make ammunition a consumable like fuel. A user controlled, turreted railgun version would probably also be possible or maybe a railgun that automatically detects other ships and fires on them.
-
The semi-major axis is the sum of the apoapse radius and periapse radius (NOT altitude) divided by two. For a circular orbit, the semi-major axis is equal to the orbital radius.
Kerbin radius = 600 000 m
Mun radius = 200 000 m
Kerbol radius = 65 400 000 m
Geostationary Kerbin orbit semi-major axis = 3 468 400 m
-
So instead of just orbiting we can modify those orbits?
The semi-major axis, eccentricity and inclination can currently be changed for any ship. Longitude of ascending node, and craft position along the orbit can\'t be changed.
Only test this with 0.14 x2 but I presume it would work for the other experimental builds.
-
A very simple program that can put ships into orbit and change the orbits of any existing ships in orbit. You can put a ship into low Kerbin orbit, geosynchronous orbit, low Mun orbit, Kerbol orbit, anything.
Put the file into the same folder as the persistent save files. Enter the name of the ship and change the orbital parameters. Program will change the orbital parameters of all ships with the same name. Program can be used while in the Space Center so you don\'t have to close KSP.
Backup your save just in case of any errors.
#include <fstream>
#include <string>
#include <stdio.h>
using namespace std;
int main()
{
ifstream saveFile('persistent.sfs');
ofstream writeFile('intermediate.sfs', ios::out | ios::binary);
string lineWhole;
int shipNumber = 0;
string shipName;
int shipOrbit;
string shipSemiMajorAxis;
string shipEccentricity;
string shipInclination;
cout << 'Enter name of ship:\n';
getline(cin,shipName);
cout << '\nEnter orbiting body:\n 0 - Kerbol\n 1 - Kerbin\n 2 - Mun\n';
cin >> shipOrbit;
cout << '\nEnter semi-major axis (in metres):\n';
cin >> shipSemiMajorAxis;
cout << '\nEnter eccentricity:\n';
cin >> shipEccentricity;
cout << '\nEnter inclination (in degrees):\n';
cin >> shipInclination;
while(getline(saveFile,lineWhole)){;
if(lineWhole.find(shipName)==8){
shipNumber = 1;
writeFile << lineWhole << '\n';
}
else if(shipNumber==1 && lineWhole.substr(1,5)=='sit ='){
writeFile << '\tsit = ORBITING' << '\n';
}
else if(shipNumber==1 && lineWhole.substr(1,8)=='landed ='){
writeFile << '\tlanded = False' << '\n';
}
else if(shipNumber==1 && lineWhole.substr(1,10)=='landedAt ='){
writeFile << '\tlandedAt = ' << '\n';
}
else if(shipNumber==1 && lineWhole.substr(2,5)=='SMA ='){
writeFile << '\t\tSMA = ' << shipSemiMajorAxis << '\n';
}
else if(shipNumber==1 && lineWhole.substr(2,5)=='ECC ='){
writeFile << '\t\tECC = ' << shipEccentricity << '\n';
}
else if(shipNumber==1 && lineWhole.substr(2,5)=='INC ='){
writeFile << '\t\tINC = ' << shipInclination << '\n';
}
else if(shipNumber==1 && lineWhole.substr(2,5)=='REF ='){
writeFile << '\t\tREF = ' << shipOrbit << '\n';
shipNumber = 0;
}
else{
writeFile << lineWhole << '\n';
}
}
saveFile.close();
writeFile.close();
int deleteResult = remove('persistent.sfs');
int renameResult = rename('intermediate.sfs','persistent.sfs');
if(renameResult!=0){
perror('Rename error');
}
if(deleteResult!=0){
perror('Delete error');
}
return 0;
}#include <iostream>
Code if you want to compile it yourself. Code is probably crappy since it\'s the first program I\'ve made with C++.
Instant Orbit / Orbit Modifier V0.1
A debris field generator that puts debris into orbit. It is limited to debris with only one part. Need to enter every orbital parameter, which may be a good or bad thing depending on your point of view.
Space is so vast that unless you have tens of thousands of parts in the same orbit, you\'re unlikely to hit anything when travelling through a debris field. As a reference, our own asteroid belt only is only around 4% of the mass of the moon.
#include <fstream>
#include <string>
#include <cstdlib>
#include <ctime>
#include <stdio.h>
#include <iomanip>
using namespace std;
double randomNumberFunction();
int main()
{
srand(time(0));
ifstream openFile('persistent.sfs');
if(openFile.is_open()){
openFile.close();
}
else{
openFile.close();
ofstream writeFile('persistent.sfs', ios::out | ios::binary | ios::app);
writeFile << '// KSP Flight State\n\nversion = 0.14.0\nUT = 0\nactiveVessel = 0\n';
writeFile.close();
}
int shipuid = time(0);
string shipName;
cout << 'Enter name of debris:\n(Name of the debris displayed in the game.)' << endl;
getline(cin,shipName);
string shipID;
cout << '\nEnter debris ID name:\n(Name of the part. The name in the config file.)' << endl;
getline(cin,shipID);
double shipMass;
cout << '\nEnter debris mass:' << endl;
cin >> shipMass;
int shipAmount;
cout << '\nAmount of debris to add:' << endl;
cin >> shipAmount;
double shipSMA;
cout << '\nEnter semi-major axis:' << endl;
cin >> shipSMA;
double shipSMAVar;
cout << '\nEnter semi-major axis variance:\n(Will add or subtract a random value up to this number to the semi-major axis.)' << endl;
cin >> shipSMAVar;
double shipECC;
cout << '\nEnter eccentricity:' << endl;
cin >> shipECC;
double shipECCVar;
cout << '\nEnter eccentricity variance:\n(Will add or subtract a random value up to this number to the eccentricity.)' << endl;
cin >> shipECCVar;
double shipINC;
cout << '\nEnter inclination:' << endl;
cin >> shipINC;
double shipINCVar;
cout << '\nEnter inclination variance:\n(Will add or subtract a random value up to this number to the inclination.)' << endl;
cin >> shipINCVar;
double shipLPE;
cout << '\nEnter longitude of periapsis (in degrees):' << endl;
cin >> shipLPE;
double shipLPEVar;
cout << '\nEnter longitude of periapasis variance:\n(Will add or subtract a random value up to this number to the longitude of periapsis.)' << endl;
cin >> shipLPEVar;
double shipLAN;
cout << '\nEnter longitude of ascending node (in degrees):' << endl;
cin >> shipLAN;
double shipLANVar;
cout << '\nEnter longitude of ascending node variance:\n(Will add or subtract a random value up to this number to the longitude of ascending node.)' << endl;
cin >> shipLANVar;
double shipMNAStart;
cout << '\nEnter start of mean anomaly where debris will spawn:\n(Mean anomaly refers to where the debris will spawn along the orbit. Enter a number between 0 and 6.2832.)\n(0 will spawn debris at periapsis, 3.1415 will spawn debris at apoapsis and 6.2832 will spawn debris back at periapsis.)' << endl;
cin >> shipMNAStart;
double shipMNAEnd;
cout << '\nEnter end of mean anomaly:' << endl;
cin >> shipMNAEnd;
double shipMNAVar;
cout << '\nEnter mean anomaly variance:\n(Will add or subtract a random value up to this number to the mean anomaly.)' << endl;
cin >> shipMNAVar;
string shipRef;
cout << '\nEnter orbiting body:\n 0 - Kerbol\n 1 - Kerbin\n 2 - Mun\n';
cin >> shipRef;
double shipMNAInc = (shipMNAEnd - shipMNAStart)/shipAmount;
ofstream writeFile('persistent.sfs', ios::out | ios::binary | ios::app);
for(int ii=0; ii<shipAmount; ii++){
writeFile << setiosflags(ios::fixed) << setprecision(8) << 'VESSEL {' << endl;
writeFile << '\tname = ' << shipName << endl << '\tsit = ORBITING\n\tlanded = False\n\tlandedAt = \n\tsplashed = False' << endl;
writeFile << '\tmet = 0\n\tlct = 0\n\troot = 0\n\tpos = 0,0,0\n\tlat = 0\n\tlon = 0\n\talt = 0' << endl;
writeFile << '\trot = ' << 2*randomNumberFunction()-1 << ',' << 2*randomNumberFunction()-1 << ',' << 2*randomNumberFunction()-1 << ',' << 2*randomNumberFunction()-1 << endl;
writeFile << '\tCoM = 0,0,0\n\tstg = 0\n\tORBIT {' << endl;
writeFile << '\t\tSMA = ' << shipSMA + 2*randomNumberFunction()*shipSMAVar - shipSMAVar << endl;
writeFile << '\t\tECC = ' << shipECC + 2*randomNumberFunction()*shipECCVar - shipECCVar << endl;
writeFile << '\t\tINC = ' << shipINC + 2*randomNumberFunction()*shipINCVar - shipINCVar << endl;
writeFile << '\t\tLPE = ' << shipLPE + 2*randomNumberFunction()*shipLPEVar - shipLPEVar << endl;
writeFile << '\t\tLAN = ' << shipLAN + 2*randomNumberFunction()*shipLANVar - shipLANVar << endl;
writeFile << '\t\tMNA = ' << shipMNAStart + ii * shipMNAInc + 2*randomNumberFunction()*shipMNAVar - shipMNAVar << endl;
writeFile << '\t\tEPH = 0' << endl;
writeFile << '\t\tREF = ' << shipRef << endl << '\t\tOBJ = 1\n\t}' << endl;
writeFile << '\tPART {\n\t\tname = ' << shipID << endl;
writeFile << '\t\tuid = ' << shipuid + ii << endl;
writeFile << '\t\tparent = 0\n\t\tposition = 0,0,0\n\t\trotation = 0,0,0,1\n\t\tistg = 0\n\t\tdstg = 0\n\t\tsqor = 0\n\t\tsidx = 0\n\t\tattm = 0\n\t\tcData = \n\t\tsrfN = None, -1\n\t\tattN = None, -1' << endl;
writeFile << '\t\tmass = ' << shipMass << endl;
writeFile << '\t\ttemp = 0\n\t\texpt = 0.5\n\t\tstate = 2\n\t\tconnected = False\n\t\tattached = True' << endl;
writeFile << '\t}\n}' << endl;
}
return 0;
}
double randomNumberFunction()
{
double randomNumber = ((double)rand())/(double)RAND_MAX;
return randomNumber;
}#include <iostream>
-
Instant orbits in 0.14 just got a whole lot easier now since orbital information is stored in the save file. Custom parts may be able to give an instant orbit, but since there\'s no documentation available right now, manual editing will have to suffice. No Battlestar Galactica style jump drives just yet.
I recommend using an editor such as Notepad++ to edit save files.
Instant Orbits
Launch a ship, and while still on the launchpad return to the Space Centre. Open the persistance.sfs file and search for the name of the ship you just launched. You should have something like this:
VESSEL {
name = Instant Orbiter
sit = PRELAUNCH
landed = True
landedAt = LaunchPad
splashed = False
met = 0
lct = 4.17999990656972
root = 0
pos = 0,0,0
lat = -0.236453328375202
lon = 27.8244657605096
alt = 66.4334788515698
rot = 0.3673681,0.6042479,0.6059003,-0.3644312
CoM = 0.0008940728,-4.480832,-0.007221261
stg = 1
cPch = 0, FLOAT
cHdg = 0, FLOAT
cMod = 1, INT
ORBIT {
SMA = 300809.642266737
ECC = 0.994822866051143
INC = 0.236455925375334
LPE = 90.2300765984522
LAN = 117.664053966235
MNA = 3.14159348003525
EPH = 4.17999990656972
REF = 1
OBJ = 0
}Change the sit, landed and landedAt fields to:
sit = ORBITING
landed = false
landedAt =Then under the ORBIT section, change the parameters to whatever you want.
SMA = semi-major axis
ECC = eccentricity
INC = inclination
LPE = longitude of periapsis?
LAN = longitude of ascending node
MNA = mean anomaly?
EPH = epoch
REF = body the spacecraft is orbiting (0 for Kerbol, 1 for Kerbin, 2 for Mun)
OBJ = what kind of object spacecraft is (0 for a controllable ship, 1 for ship to be debris)
-
Needs moar debris.
-
Instant orbits are even easier in 0.14. You easily change altitude, eccentricity and inclination of orbits now.
Has the added bonus of your ship not exploding into a million pieces too.
-
You could always write an autopilot module in 0.14 that does the whole mission automatically.
-
How do people manage to do this in reality? It\'s impossible I tells ya.
-
Any chance that we could have mini railguns?
Maybe. I\'m really lazy though, so don\'t hold your breath for it.
Could you try to make the bullets have a collision only at their bottom? that way we could stack WAAAAY more stuff...
Use the smaller training rounds. Funny things happen it the bullet travels too fast and the node collider is too small.
-
Mind posting a pic or two of the ship... thats mind boggling and must have been a slideshow to get into orbit..
112 liquid fuel tanks (not including fuel tanks intended for orbit) and 36 engines. The thrust to weight ratio of the upper stages is quite low to save weight.
-
http://kerbalspaceprogram.com/forum/index.php?topic=5506.msg75818#msg75818
Yeah. If anyone successfully manages this with stock parts, then I\'m afraid we\'ll have to burn them.
'Thou shalt not suffer a witch to live.'
Video unrelated:
-
44 full fuel tanks into a 74x74 km orbit
Final score = 3130 points.
Radially attach the fuel tanks and you descend back to Kerbin using your ascent stage.
-
A spreadsheet to calculate various properties of rockets. Calculates delta-v, thrust to weight ratio, mass ratio and some other stuff. Now you can play KSP without actually playing KSP.
Use together with my delta-v map of the current solar system.
Only stock part data is included but other part data can be easily added. Can also be useful for balancing parts without having to continuously load and reload KSPs.
Disclaimer: Use of this spreadsheet may or may not take the fun out of KSP.
Fun with Save File Editing (Instant Orbits for 0.14)
in KSP1 Mod Development
Posted
INC should be 0 and not 180.