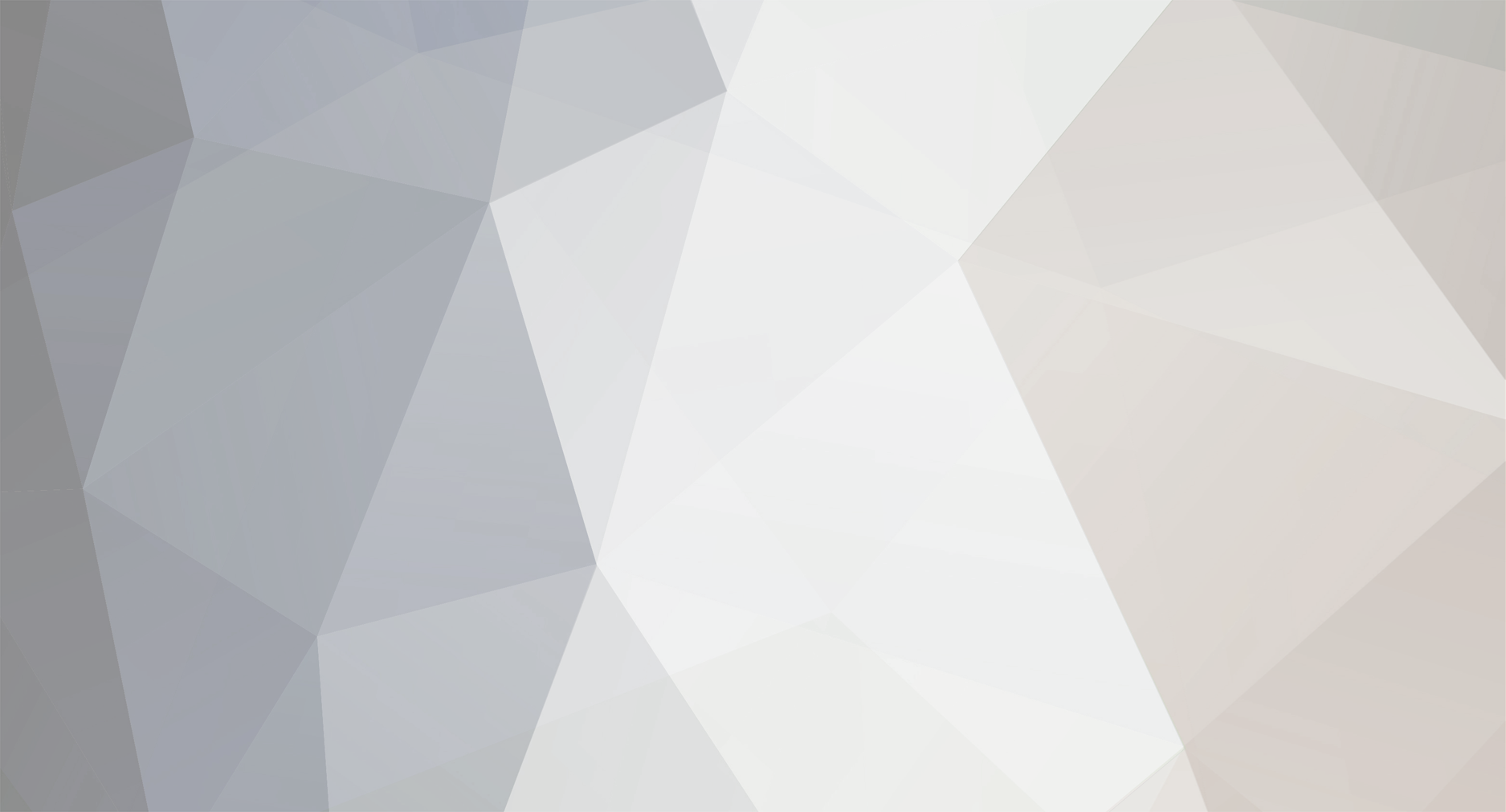
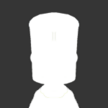
jamespicone
Members-
Posts
107 -
Joined
-
Last visited
Content Type
Profiles
Forums
Developer Articles
KSP2 Release Notes
Everything posted by jamespicone
-
EDIT: Now has a SpaceDock page: http://spacedock.info/mod/288/BackgroundProcessing. The SpaceDock page is the best source of information on what the mod currently does and how to use it. I'm working on a little mod/library to make it easier to write PartModules that do something when a vessel is not being actively controlled by the player - say, because they've left it landed or orbiting and gone back to the space center, or undocked it, whatever. For an example of mods that currently do something like this, SCANSat's scanners can continue mapping a planet even when the vessel with the scanners on them is in the background. I'm also intending to fake resource handling for certain resources while vessels are backgrounded - ElectricCharge in particular. Essentially I'm replicating some of the functionality of ASMI's RealTimeModules with a more convenient license (MIT). The source is here, but keep in mind that this is early days and the mod doesn't do much of use for players yet, and is only just usable by mod authors for backgrounding. I'm posting here because it has, at least, gotten to the point where as a mod author you could theoretically use it and it would be theoretically useful. It works like this: It creates a MonoBehaviour at startup, BackgroundProcessing.Addon, with a RegisterHandler method, and provides an interface, IBackgroundHandler. PartModules that want background processing should, in their OnAwake method, create a new, static object implementing IBackgroundHandler (that is, there is one IBackgroundHandler per PartModule /type/), and should then call BackgroundProcessing.Addon.Instance.RegisterHandler(). Once again, that's all in OnAwake - don't register handlers after that. Then, during play, if the background processing library picks up a backgrounded vessel, it calls IBackgroundHandler.FixedBackgroundUpdate() on the appropriate handler for every partmodule in the vessel, passing in a reference to the vessel (which may or may not be all there - be very careful with it. It won't be null though), and a part flightID which will allow the handler to determine exactly which partmodule it was that needs handling. It's extremely simple. The next step is to implement some handling for electric charge in the background. EDIT: The method mod authors should use to interface with this library/mod has changed. Right now, on startup, the mod checks all PartModules in the system to see whether they have certain methods. If they do, those methods are called at the appropriate times. If you want your PartModule to perform any kind of processing when the Vessel it is on is out of load range, define: class MyPartModule : PartModule { public static void FixedBackgroundUpdate(Vessel vessel, uint partFlightID); } it'll get called at fixed intervals with the relevant vessel and the partFlightID of the part that owns the module that triggered the background call (so you'll get multiple calls per vessel if there are multiple PartModules with backgrounding on the same vessel). Think of it as FixedUpdate for when you don't exist. If your PartModule should produce or consume a resource in the background, or if your mod wants a particular resource handled in the background, you can do that too. Override these methods: class MyPartModule : PartModule { public static List<string> GetInterestingResources(); public static int GetBackgroundResourceCount(); public static void GetBackgroundResource(int i, out string resourceName, out float rate); } GetInterestingResources() should return a List<string> containing the resource name of every resource your PartModule thinks should be handled in the background. ElectricCharge is an interesting resource by default, no others are. For example, a hydrogen ramscoop PartModule that sucks up interstellar hydrogen from a future-tech mod that has a Hydrogen resource might return {"Hydrogen"}. GetBackgroundResourceCount() and GetBackgroundResource() form a pair used to indicate that your PartModule produces or consumes a particular resource in the background consistently - either your PartModule is a constant drain or some kind of generator. GetBackgroundResourceCount() should return the number of different resources your PartModule produces in the background, and GetBackgroundResource() should return the resource name and the rate (in units per second) that that resource is produced for the i-th resource your PartModule produces. For example, the hydrogen ramscoop PartModule I just mentioned would look like this: public static int GetBackgroundResourceCount() {return 1;} public static int GetBackgroundResource(int i, out string resourceName, out float rate) {resourceName = "Hydrogen"; rate = "0.001f";} I'm currently working on providing some methods backgrounded PartModules can use as an analog to RequestResource, so that the ramscoop above could be speed-dependent, for example. Also bugfixes, improving some code, making solar panels behave properly, cleaner timewarp handling, etc. etc.
-
I'm trying to detect a Vessel being backgrounded from a PartModule on the Vessel and pass the Vessel ID on somewhere else. When I say 'backgrounded', I mean when the player leaves a vessel in flight and goes to the space center. From what I can tell PartModule.OnDestroy() gets called when recovering or backgrounding a Vessel, but it's also called all the goddamn time (like twice when starting a flight before there's even a ship on the launchpad), and vessel is sometimes null when it's called - not sure if it's reliable when it is or isn't null by then. OnInactive() doesn't seem to be called for backgrounding. I haven't tried listening for events yet - OnVesselDestroy() seems like the obvious one, but also looks like it'll get a bunch of spurious calls as well. OnVesselLoaded() would be perfect were it not the wrong way around. Thoughts?
-
How does Science work?
jamespicone replied to jamespicone's topic in KSP1 C# Plugin Development Help and Support
Figured it out, I have to call ResearchAndDevelopment.GetSubjectByID() during recovery processing to get the ScienceSubject to do stuff to. -
How does Science work?
jamespicone replied to jamespicone's topic in KSP1 C# Plugin Development Help and Support
I've done some more work on this and asked around a lot in the #kspmodding channel on EsperNet, the central class here is ResearchAndDevelopment. In particular, ResearchAndDevelopment.GetExperimentSubject, GetExperiment, GetScienceValue, and SubmitScienceData. I've now got my mod at the point where it can generate pseudo-ScienceData and when the vessel is recovered it gets collected. Buuuuuttttt.... the Research and Development archives don't update properly, science doesn't update properly, and in general it's not quite functioning right. Code is here: https://code.google.com/p/ssaad/source/browse/ . I'm doing the following to update science during vessel recovery: public void handleRecoveryProcessing(ProtoVessel pv, MissionRecoveryDialog d, float f) { foreach (JMPScienceSubject s in scienceData.Keys) { float sci = ResearchAndDevelopment.GetScienceValue(scienceData[s], s.subject); ResearchAndDevelopment.Instance.SubmitScienceData(scienceData[s], s.subject); d.AddDataWidget(new MissionRecoveryDialog.ScienceSubjectWidget(s.subject, scienceData[s], sci)); } scienceData.Clear(); capacityUsed = 0; part.RequestResource("DataStorage", -capacity); GameEvents.onVesselRecoveryProcessing.Remove(handleRecoveryProcessing); } The MissionRecoveryDialog is displaying 0.0 for science earned (which I can fix by adding to MissionRecoveryDialog.scienceEarned, but that doesn't seem like the right way to do things), and while the subjects are showing up in the research and development archives, they're displaying as having 0 science done on them and the bar is completely empty. I also get full value of any additional missions hitting the same science subject. Anyone have any ideas why that's happening and how I can fix it? I've tried playing around with the fields of ScienceSubject during recovery processing and that doesn't seem to be helping any. -
What's the best way to get what biome a vessel is in for a science context? I'm currently doing this: string biome = vessel.landedAt ?? ""; if (biome == string.Empty) { biome = ScienceUtil.GetExperimentBiome(vessel.mainBody, vessel.latitude, vessel.longitude); } based on some stuff I've seen in a few other mods. Seems to work fine for getting the biome from the biome map, but getting the special KSP-centre biomes using vessel.landedAt isn't doing what I expect - it's 'Launchpad' for the first few frames, and then it changes to 'KSC_LaunchPad_platform'. Actual ModuleScienceExperiments give 'Launchpad' for the same location, no matter when they're fired. Is there a better solution than figuring out what all the possible values are and mapping them to the appropriate science-biome-name?
-
Hi all. I've got some ideas for a mod to change the way science data is collected/stored/converted into science points (briefly, instead of experiments that run once and store data, you have sensors that collect data continuously, comms antennas send it back continuously, and it asymptotically approaches max science for that biome/situation/data type situation), but I'm running into a bit of a block in that the various science classes aren't terribly well-documented, at least as far as I can see. Does anyone know what the 'science lifecycle' is? My current understanding is something like this: - Vessels have a ModuleScienceExperiment and optionally a ModuleDataTransmitter and some ModuleScienceContainers. (Do EVAs have a ModuleScienceExperiment? Crew capsules definitely do.) - At some point in flight, DeployExperiment() or DeployAction(KSPActionParam) get called. That triggers any relevant animation and creates a ScienceData. ScienceData is created as a number of mits, with an id that points into the science subject table and provides the data-per-mit information. Also stores the reduction for transmission and the boosted-by-lab state per-sciencedata. The ModuleScienceExperiment keeps that ScienceData in an internal array. Relevant: ScienceUtil is used to get experimental biome/situation. What does GetTransmitterScore do? - If comms: ModuleDataTransmitter::StartTransmission() or TransmitData() get called, do something to increment science at home, and call ModuleScienceExperiment::DumpData() - If collected by Kerbal: ModuleScienceExperiment::CollectDataExternalEvent() gets called, does something? - If moved into ModuleScienceContainer: Don't know. Presumably AddData() gets called on the modulesciencecontainer or something? Which object is responsible for this? When is this used? Presumably EVA Kerbals returning to a command pod or the like trigger this. - If recovered: ScienceData in modules is transformed into science points somehow. Essentially I'm interested in how the conversion to science points happens and how I can do it myself - essentially, implementing MyModuleScienceExperiment that does things completely differently. I'm not sure there's a clean way to implement what I'm thinking of doing on top of the current mechanics - maybe have a ModuleScienceExperiment that every FixedUpdate() makes ScienceData at very small data sizes and then SendEvent(StartTransmitting()), but that's pretty ugly. I'm not sure I can safely 'merge' ScienceData objects. Any thoughts?