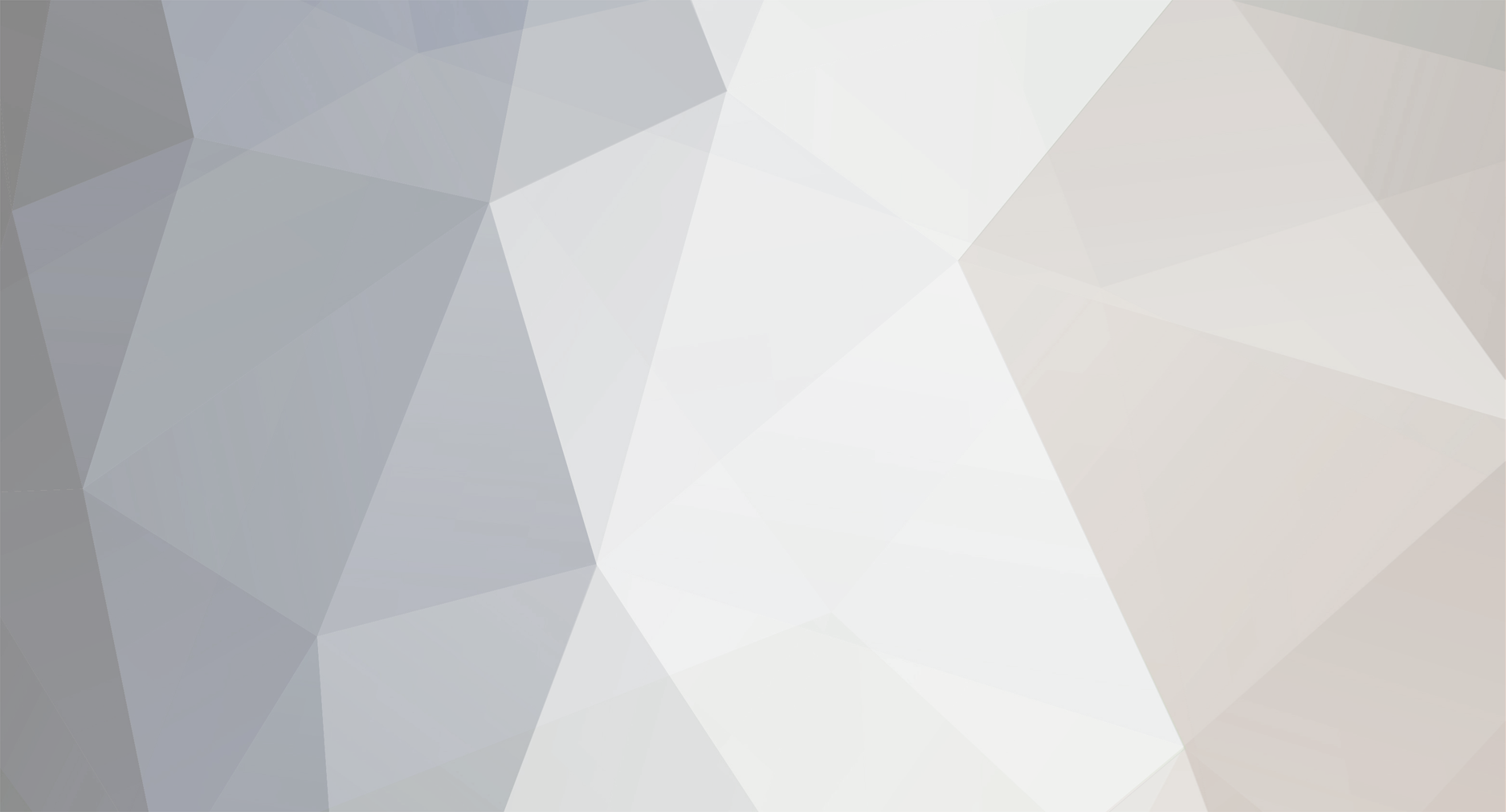
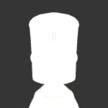
taio
Members-
Posts
38 -
Joined
-
Last visited
Content Type
Profiles
Forums
Developer Articles
KSP2 Release Notes
Everything posted by taio
-
LOL Squad… Ran into something else I thought I’d share. Some of the cfgs are in a bizarre encoding. Wrote this Python script to investigate (after removing all mod files): import chardet import fnmatch import os from collections import Counter path = 'C:/Program Files (x86)/Steam/SteamApps/common/Kerbal Space Program/GameData/' result = {} c = Counter() for root, dirnames, filenames in os.walk(path): for filename in fnmatch.filter(filenames, '*.cfg'): filepath = os.path.join(root, filename) x = chardet.detect(open(filepath, "rb").read()) result[filepath] = (x['encoding'], x['confidence']) c[result[filepath]] += 1 print(c) # frequency table bad = [] # find all non-ascii characters by file, row, and column for (k, v) in result.items(): if v != ('ascii', 1.0): bad.append(k) print(k[76:], v) # strip beginning of path for file in bad: print(file[76:]) for col, line in enumerate(open(file, encoding='utf-8')): for row, ch in enumerate(line): if ord(ch) >= 128: print('\t[{0}:{1}] {2} ({3:4x})'.format(row, col, ch, ord(ch))) It gives this output: Counter({('ascii', 1.0): 266, ('UTF-8-SIG', 1.0): 6, ('ISO-8859-2', 0.8515969760557984): 1}) Squad\Parts\Utility\ladderTelescopic\ladderTelescopic.cfg ('UTF-8-SIG', 1.0) Squad\Parts\Utility\ladderRadial\ladderRadial.cfg ('UTF-8-SIG', 1.0) Squad\Parts\Command\advancedSasModuleLarge\advSasModuleLarge.cfg ('ISO-8859-2', 0.8515969760557984) Squad\Parts\Utility\ladderTelescopicBay\ladderTelescopicBay.cfg ('UTF-8-SIG', 1.0) Squad\Parts\Science\GooExperiment\gooExperiment.cfg ('UTF-8-SIG', 1.0) Squad\Resources\ScienceDefs.cfg ('UTF-8-SIG', 1.0) Squad\Parts\Utility\commsAntennaDTS-M1\commsAntennaDTS-M1.cfg ('UTF-8-SIG', 1.0) Squad\Parts\Utility\ladderTelescopic\ladderTelescopic.cfg [0:0]  (feff) Squad\Parts\Utility\ladderRadial\ladderRadial.cfg [0:0]  (feff) Squad\Parts\Command\advancedSasModuleLarge\advSasModuleLarge.cfg [261:41] ™ (2122) Squad\Parts\Utility\ladderTelescopicBay\ladderTelescopicBay.cfg [0:0]  (feff) Squad\Parts\Science\GooExperiment\gooExperiment.cfg [0:0]  (feff) [19:16] ™ (2122) Squad\Resources\ScienceDefs.cfg [0:0]  (feff) [20:237] ™ (2122) Squad\Parts\Utility\commsAntennaDTS-M1\commsAntennaDTS-M1.cfg [0:0]  (feff) The number after the encoding is a certainty that ranges from 0 to 1. All the files are ASCII, except that six start with the byte‑order marker U+FEFF. In other words, they are encoded in utf-8-sig, which is idiotic. The detection code thinks one additional file is in Latin-2, but it’s wrong (well, it’s right, but it’s also UTF-8); the only non-ASCII character besides U+FEFF in these files is the trademark symbol, which is perfectly fine UTF-8. TL;DR: When you open one of the six utf-8-sig files, you will probably need to strip the first character, or your parsing code will probably reject it. I just added the line str = strip(str, '\ufeff') just after reading the file into a string, which covers all cases. Once you do that, the rest of the file/string is UTF-8.
-
I’ve been writing my own in Julia for another project, so here’s another format issue you’ll run into: apparently, leaving off a “}†at the end of the document (i.e. leaving the last top‑level node unclosed) is tolerated. And a bunch of the stock cfgs do this. Sigh…
-
Found a logic bug. The parser assumes cfgs have a strict format that (for example) MechJeb’s MechJeb2_AR202/part.cfg does not conform to. That file has four top‑level nodes, where your parser assumes one. Also, where your parser assumes braces are always on their own lines, that file has several child nodes on one line, e.g.: MechJebModuleCustomWindowEditor { unlockTechs = flightControl } There are other mod parts that don’t have braces on their own lines, though I haven’t noticed any that had > 1 top‑level node.
-
Using ConfigNode outside KSP
taio replied to Ippo's topic in KSP1 C# Plugin Development Help and Support
This was just what I needed. Thanks! -
Mass-optimal engine type vs delta-V, payload, and min TWR
taio replied to tavert's topic in KSP1 Tools and Applications
Ah, I see. Thanks. -
Mass-optimal engine type vs delta-V, payload, and min TWR
taio replied to tavert's topic in KSP1 Tools and Applications
John FX, could I get a link to the code you’re using, and if convenient a brief description of the differences from tavert’s April code? I’d like to add some mod parts and generate some charts of my own. -
[1.0.2] NovaPunch 2.09. - May 6th - 1.0 Compatibility Update
taio replied to Tiberion's topic in KSP1 Mod Releases
Thanks for the quick replies. -
[1.0.2] NovaPunch 2.09. - May 6th - 1.0 Compatibility Update
taio replied to Tiberion's topic in KSP1 Mod Releases
The Radial Liquid Booster has no fuel. Is this working as designed, or a bug? Generally you don’t call it a booster when it has an engine but no internal fuel… right? -
I had the same issue on a Pentium T4500 (dual core, 2.3 GHz, 1 MB L2 cache), old integrated Intel graphics, 4 GB RAM, 1366x768 display. This post resolved my problems. It’s still slower when a planet is in view, but it’s just barely noticeable. It’s good enough that I leave planets in view. If you follow the link you’ll see his description of his own content is outdated, but IIRC I’m running the High V2 config.
-
How was I supposed to handle the 0.90 update with CKAN? Here’s how it went: Steam updates KSP to 0.90; I update CKAN to 1.3.0 and open it. I refresh repository & see that some mods are compatible, some aren’t, some need updates, and some have no updates. The incompatible ones that also have no updates have no option to uninstall. I update the mods I can update, then manually remove the incompatible mods, and reload CKAN. It tells me I still have the incompatible mods installed. (That is, as before, they appear when I filter by “Installed,†yet have a “-†in the “Installed†column, which is confusing.) I delete GameData/CKAN and all mods. Reload CKAN, install compatible mods, manually restore backed‑up mod settings. I assume this was not the intended procedure.
-
The intellectual carefulness in each aspect of this project is terrific. I have only glanced at bits of the code, but I did love the bit about Sputnik romanization. I have a vague suggestion about conveying information about fields like gravitational potential. If, in addition to a visual plot on some reference plane, it is helpful to give the player info about a single, selectable point in space, I think it is best selected by the player clicking once on that point (defining a line through space normal to the camera perspective), rotating the camera some amount, and clicking on it again (the second click being clamped to the line defined by the first click). Whenever I have seen a graphical control for selecting a point in three dimensions, it has been done by having the user select a point on a reference plane, and then offsetting it normal to that plane. This is needlessly awkward. The two clicks could also be used to select a plane along which to plot the potential, the plane given by the two intersecting lines. This might be more relevant than my first thought about only one point. Another good way to control the plane for plotting the potential is simply to select the one normal to the camera perspective and passing through the barycenter (or a craft, or a point chosen manually)  in real time as the camera rotates. This would greatly enable the player in building a mental picture of the potential field, especially if it could somehow be adapted to n bodies.
-
Exploring The System - A design tutorial campaign 0.90 Final
taio replied to Pecan's topic in KSP1 Tutorials
This is a great piece of work. As a beginner I have mostly followed it through. However, I have found some problems with your Orbital SP design in the latest version of KSP. The .craft file works as specified, but the stock parts are different, so that the same design cannot be easily reconstructed by a reader. At least these things are different: The old Mk2 Fuselage is now called the Mk2 Liquid Fuel Fuselage. Crucially, the part’s mass and fuel capacity have been modified. The old Mk2 Cockpit is now called the Mk1 Inline Cockpit. The old Small Control Surface is now called the Elevon 4. Various parts (at least the Delta Wing and Elevon 4) have changed models, so that your pictures aren’t 100% representative. Due mostly or entirely to the fuselage change, the total mass of the craft is 12.3 tons instead of the quoted 9.8 tons. I wasn’t able to get it much higher than 15 km on jets. Also, it was while trying to construct this craft that I first had a need to manually rotate parts in the editor with WASDQE, and I did not at first realize that this function was even available, so I had to do some confused searching. A brief mention about this would be great, especially when you have the cubic octagonal struts placed. (I believe you rotate them so they clip inside the fuel tank, but do not explain this technique.)