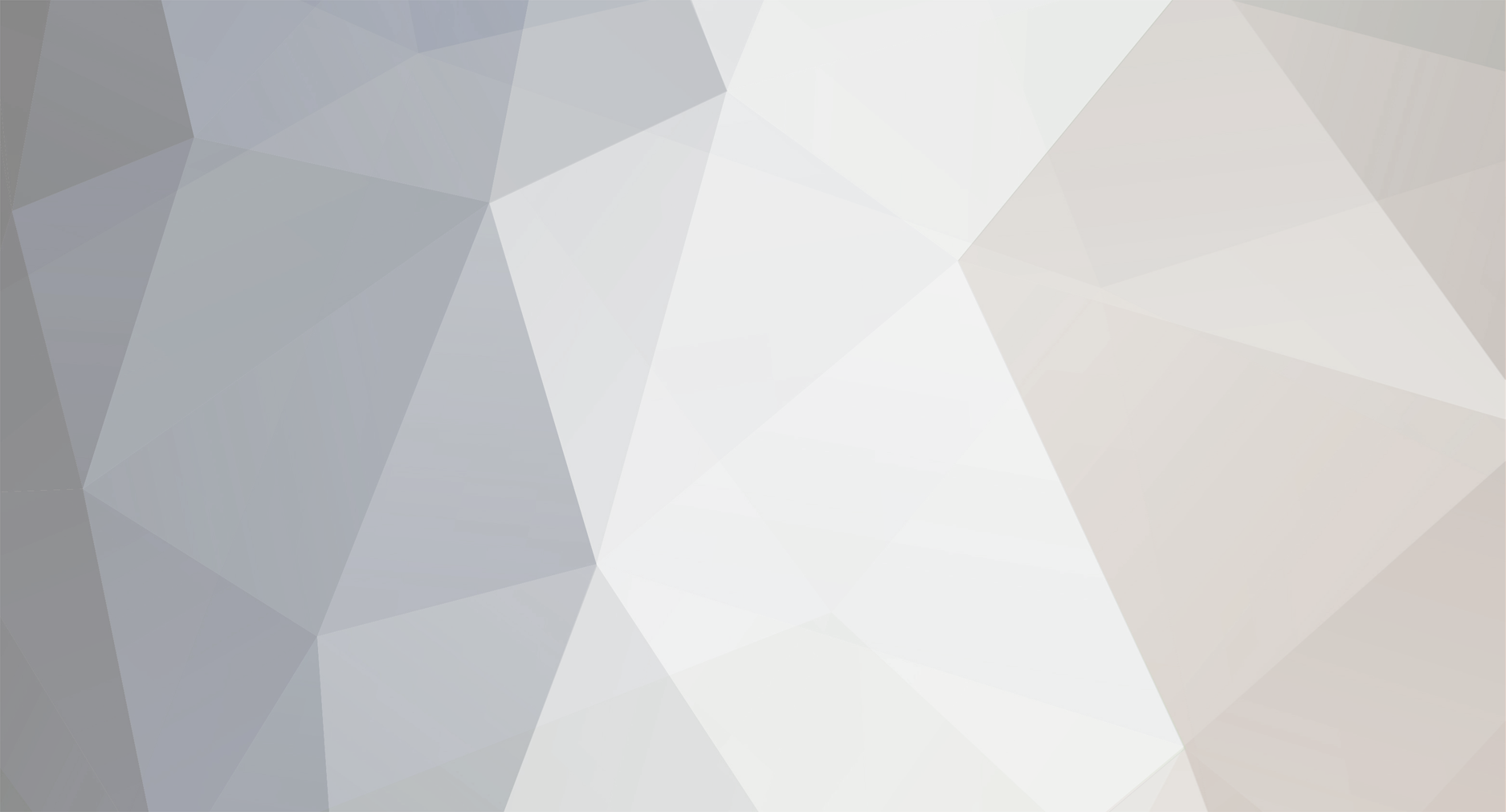
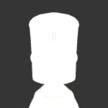
kellven
Members-
Posts
293 -
Joined
-
Last visited
Content Type
Profiles
Forums
Developer Articles
KSP2 Release Notes
Everything posted by kellven
-
My workaround for custom type persistence: 1. Add a [KSPField] string to your PartModule, and override OnAwake and OnLoad like this: public class MyModule : PartModule { [KSPField] public string MyPersistantData; public MyDataStructure MDS0 = new MyDataStructure(); public override void OnAwake() { if (MDS0 == null) MDS0 = new MyDataStructure(); DeSerialize(); //read all the data stored in MyPersistantData back into the relevant places. base.OnAwake(); } public override void OnLoad(ConfigNode node) { foreach (ConfigNode subnode in node.nodes) { switch (subnode.name) { case 'DuelingInts': { MDS0.Load(subnode); break; } } } Serialize(); //store all necessary data into MyPersistantData base.OnLoad(node); } public void Serialize() { MyPersistantData = string.Empty; if (MDS0 != null) MyPersistantData += MDS0.ToString(); //can store more data here, just use a delimiter like in the class } public void DeSerialize() { if (string.IsNullOrEmpty(MyPersistantData)) return;//fail if nothing was saved //can split the string into substrings here like in the class if you\'re packing multiple objects into it MDS0.Read(MyPersistantData); //this returns a bool for handling multiple object packing } } 2. Setup your class you need persistent data storage for like so: //need this for culture normalization, crazy Euros use all kinds of funny stuff using System.Globalization; // public class MyDataStructure { public int Data0; public int Data1; //These two just keep things consistent private static CultureInfo cult = CultureInfo.InvariantCulture; private static NumberStyles style = NumberStyles.Float | NumberStyles.AllowThousands; // private static string[] Delimiter = new string[] { '*' }; private static int NumEntries = 2; /* Just an example of validating data private bool ValidateMe(int i) { if (i < 17 && i > 3) return true; return false; } */ //as this class does not use the IConfigNode interface or loading mechanism, this method can be named anything public void Load(ConfigNode node) { if (node == null) return; //used tryparse here as that does not throw exceptions int.TryParse(node.GetValue('inta'), out Data0); int.TryParse(node.GetValue('intb'), out Data1); } public bool Read(string s) { if (string.IsNullOrEmpty(s)) return false;//Failure string[] strarr = s.Split(Delimiter, System.StringSplitOptions.RemoveEmptyEntries);//Holds array of string encoded values if (strarr.Length != NumEntries) return false;//Failure, incorrect number of serialized elements, or corruption int[] intvals = new int[NumEntries];//will hold array of decoded values bool valid = true; for (int i = 0; i < NumEntries; i++) { valid &= int.TryParse(strarr[i], style, cult, out intvals[i]);//Try to decode values, and keep a running failure check //valid &= ValidateMe(intvals[i]); //Example of another check to make sure the value is valid. } if (valid) //if all the data decoded and validated right, fill it in { Data0 = intvals[0]; Data1 = intvals[1]; } return valid; } public override string ToString() { string s = string.Empty; s += Data0.ToString(cult); s += Delimiter; s += Data1.ToString(cult); return s; } } 3. Setup part.cfg like so: // Kerbal Space Program - Part Config // // // --- general parameters --- name = KISConnect module = Strut author = Kellven // --- asset parameters --- mesh = ConnectV2.dae scale = 1 texture = ConnectV2.png specPower = 0.1 rimFalloff = 3 alphaCutoff = 0 // --- node definitions --- node_attach = 0.0, 0.0, -0.10, 0.0, 0.0, 1.0 // --- editor parameters --- cost = 1000 category = 1 subcategory = 0 title = KIS Connect v2 manufacturer = Kellvonic Imperial Spaceyard description = Stick just one of these on your ship, and it will automagically make the parts connect better. Adding more than one will not increase connection betterness. // attachment rules: stack, srfAttach, allowStack, allowSrfAttach, allowCollision attachRules = 0,1,0,1,1 // --- standard part parameters --- mass = 0.000001 dragModelType = default maximum_drag = 0 minimum_drag = 0 angularDrag = 0 crashTolerance = 100 breakingForce = 1000 breakingTorque = 1000 maxTemp = 2900 fullExplosionPotential = 0.0 emptyExplosionPotential = 0.0 MODULE { name = MyModule DuelingInts { inta = 1 intb = 2 } } Just used my own part as an example. It\'s a strut, shows up in strut section, and does nothing but read two ints from part.cfg into a data structure, but it should persist that structure through the various scenes. There might be a couple of errors in here still, I did a quick rewrite of my code into a general purpose example, but the general workaround idea does work in game.
-
[Plugin] [Part] [0.17] KIS Connect V7a [2Oct12] [MIT]
kellven replied to kellven's topic in KSP1 Mod Releases
Sorry this is taking so long, but I\'m fairly certain the new plugin system is broken with non-basic type persistence, which I actually have to workaround to get a fix for this to work. Here\'s what I *think* the problem was, at least the one I saw. 1. The new model system ends up with tighter collision boxes, and the collision handling itself was set to be 'harder'. 2. In the 0.14x version, the joints being 'tightened' didn\'t have noticeable effect on this because the rest of the system was so loose to begin with. Which is why wobblyness caused such severe problems. 3. With the new 'tightened' collision, added to the 'tightening' of the joints I introduced, what we have now is an 'echoing cascade of near perfectly elastic collisions'. In human speech, we went from dropping a quarter on a pile of laundry to dropping a quarter on an army bunk in basic training. When the joints are tightened by this plugin, it becomes dropping a quarter on a sheet of tool steel. 4. This shows up as the rocket dancing merrily about the launchpad when it\'s dropped to ground after reassembly from the VAB transition. In big rockets, might just disassemble instead. 5. Why North? I have absolutely no idea, but I think it\'s just physX\'s underwear peeking out. Probably related to the rotation you get whenever you stick too many radial engines on a large mass ship in vacume. The collision echo wouldn\'t just happen on the launchpad, it would also happen inflight, as the new colliders are nudging each other constantly, I think one of the devs said they were having issues with that in 0.15 normally. Most likely, the net vibrations just end up resolving in a shift north for some unknown algorithmic reason. 6. What do you intend to do about it, and why is it taking so blasted long? Re-setup the joint replacer to add a little give in the form of dampening(Like sticking some bubblewrap between the parts so they don\'t bang into each other so hard). It\'s taking forever because I can load the softjointlimit data into the new plugin architecture, but they new system refuses to save that data once it gets it, as it\'s not one of the simple predefined types. I finally have a mostly working custom serializer, but I think I must have screwed up the logic in one of the validators. -
I still don\'t understand how the data loaded into an IConfigNode class is supposed to persist through scenes once it gets loaded from part.cfg, but I have a basic order of operations for the PartModule in action, so I\'ll share that. ->part is created(added to scene, or created from part.cfg) 1. PartModule constructor called 2. PartModule OnAwake called 3. : IConfigNode class constructors for [KSPField] fields in PartModule class 4a. : IConfigNode class Load() called '' if this is the part.cfg reading scene <-This is not done elsewhere 4b. : IConfigNode class Save() called '' this seems to happen when launching from VAB, and starting flightscene. It appears to be tied to the flightstate saving. It is not called after reading part.cfg. 5a. PartModule OnLoad() called '' <-This is done when reading part.cfg, nowhere else 5b. PartModule OnSave() called '' <-This is done when saving the flightstate, after the [KSPField] Save() calls 6. PartModule OnStart called <-This is not done when reading part.cfg, but is done in VAB/Flightscene. Flightscene happens just before PQS (KerbinOcean) UpdateInit=3.098877s (1252 quads) PQS (OceanPQS) UpdateInit=0.001693726s (44 quads) 7. OnUpdate etc called once the part is activated. This could be problematic for parts that should update when inactive.
-
[Plugin] [Part] [0.17] KIS Connect V7a [2Oct12] [MIT]
kellven replied to kellven's topic in KSP1 Mod Releases
Persistence of non-default types is still completely fubar for me, so cfg settable soft joint limits are out. The workflow and even overridden method invocation are quickly unraveling with this module. In human speak, don\'t be expecting a new version today, the new plugin system is about as sturdy as the joints this thing is supposed to replace. -
[Plugin] [Part] [0.17] KIS Connect V7a [2Oct12] [MIT]
kellven replied to kellven's topic in KSP1 Mod Releases
I finally saw the problem myself. Yay me! I can think of a couple explanations, but it doesn\'t seem to be directly related to the sat stuff in any way. I didn\'t actually launch the ship, as it was dancing a pretty pirouette around the pad without any help from me, but I think I understand what\'s going on, maybe... Running the plugin in the new PartModule system I think should fix or eliminate one possible cause. Setting up the spring node like I said I was going to should eliminate another. -
[Plugin] [Part] [0.17] KIS Connect V7a [2Oct12] [MIT]
kellven replied to kellven's topic in KSP1 Mod Releases
noneyabidnis & Zhent, were you both by chance using Satellite Relay Network? I noticed the GUI from the screenshot. I have a vague notion that the course shift might possibly be caused by my destroying the stack nodes when replacing them. I\'m thinking that might cause some parts to get sent a message that makes them think they were 'forcibly disconnected' from the main ship. I\'ll rewrite the plugin now with the new PartModule system, and try to replace the stack joints before I destroy the old ones, and see if I can replicate the course 'correction' north with the sat plugin running. I noticed when analyzing the new plugin system that I can catch when the joints need to be replaced much easier under it. Unfortunately, that looks to be the only easy part of the system. -
Module and Resource example
kellven replied to kellven's topic in KSP1 C# Plugin Development Help and Support
I believe those Module classes are just the examples from Mu\'s thread. StackIcon.partModule is very interesting, have to take a look when I get through fixing whatever is fubar with my joint fixer plugin. I\'m thinking it might possibly be an extension to the normal stack icons, as they\'re generally tied to the Part, not the PartModule. I had to cheat to get my fuel tank part to show a fuel tank icon. -
Module and Resource example
kellven replied to kellven's topic in KSP1 C# Plugin Development Help and Support
If you want it to show up as in the 'pick a pod' list, you need to set it as: // --- general parameters --- name = advancedmk1pod module = CommandPod author = Maraz | Mrbrownce AFAIK, there are no 'modularized' versions of the CommandPod, ASAS, or MechJeb as of yet, so no you can\'t do that so far without writing your own versions of them. The problem is that they haven\'t been updated to the new PartModule system yet, the classes CommandPod, AdvSASModule, MuMechJeb are still setup as being their own parts, not to work as assignable PartModule(s). Once we get versions of them that are set up as PartModules, that should work like you had it. -
[Plugin] [Part] [0.17] KIS Connect V7a [2Oct12] [MIT]
kellven replied to kellven's topic in KSP1 Mod Releases
Might have something to do with the radial soyuz style engines, I\'ll have to build a similar one, and try it out. -
You might very well be right, but there is Part.AddModule(), which I was assuming is available at runtime to plugins, which would necessitate the list of fields being dynamic. If it\'s not going to work properly, that\'s another problem in itself. I think the fields list is actually a 'public BaseFieldList Fields { get; }', I tried looking in BaseFieldList, but couldn\'t make much sense of it, too much hidden code.
-
I haven\'t gotten around to messing with the GUI stuff yet, but I think we ran into the same underlying issue. The [KSPField] attribute requires one of the listed types, or a class that implements the IConfigNode interface. What you\'re specifically asking for is to pass a List<T> to whatever it is that handles the GUI. I am also 'losing' my list through nonpersistance, as [KSPField] doesn\'t handle List<T> objects. What I think will work, and am going to try is to wrap my list inside a class that implements IConfigNode, and the Load/Save members to resolve the strings back into a list. If that works like I think it should, I can then apply [KSPField] to an object of my wrapper class type, and it\'ll persist properly. What you will probably need to do is add more stuff to the wrapper class to let whatever the GUI code is resolve it to whatever it needs to. As far as actually adding [KSPField] stuff at runtime, I found something here that may not work due to it\'s specific limitations, and here that may work using reflection.emit to assemble and manufacture a custom class at runtime. In general though, because [KSPField] is an attribute, it\'s in no way dynamic at runtime, unless a reflection.emit class assembler and factory class scheme work out. But that is really, really more involved than modding a game should ever become.
-
Module and Resource example
kellven replied to kellven's topic in KSP1 C# Plugin Development Help and Support
Been working on this still. Wanted to let anyone who was dissecting this know that it looks like the engine is loading it\'s required propellants correctly when the part cfg file is parsed, but then somehow loses that data by the time the ship actually gets to the runway. So while the engine appeared to be working, it wasn\'t actually burning (or even checking) any of the resources it was supposed to. I also managed to make the stack icon update to a fueltank icon if it\'s left at mysterybox, and I think the only thing stopping the fuel bars showing now is that the fuel level is never actually adjusted by the engines. I\'ll re-upload it once I get the propellant persistence problem sorted out. -
GameInfo.gameScene not accurate.
kellven replied to jhultgre's topic in KSP1 C# Plugin Development Help and Support
I noticed the same thing a couple hours ago working on my modularized plugin example. Also showing up as 'Loading' when grabbed in flight. If you\'re using the new OnStart(), 'PartModule.StartState state' does show relevant you can use, but you need to be using the PartModule system. I ran into a ton of bugs like this, that weren\'t immediately apparent doing a straight port of my 0.14 code. That method, and the state will prove very useful I think, as scene switch detection was a serious problem with 0.14, and it seems to be called with the appropriate state each time the scene switches. -
[Plugin] [Part] [0.17] KIS Connect V7a [2Oct12] [MIT]
kellven replied to kellven's topic in KSP1 Mod Releases
Anything would be helpfull really, even just a screenshot. I\'m not even sure where the NP parts are anymore, but I\'ll take a look when I get a second. I\'m working out some kinks in the new code architecture regime that\'s supposed to come in with 0.16, once I get a couple problems worked out, I think I can do a much cleaner port to 0.15. I really would like to get this problem you\'re having fixed, there\'s just so many other changes that happened internally with 0.15, that it\'s not obvious where the issue lies. -
It seems that under the new regime, >model/obj_gimbal< is a string that gives me a working solution to my earlier problem: That\'s not what it looks like in my version, but there\'s potentially something there I can\'t figure, as I don\'t touch modeling 'art' stuff if I can avoid it. thrustVectorTransformName is supposed to be a string denoting 'something' that\'s got with part.FindModelTransform(thrustVectorTransformName) I assume this is done either to confuse us, or to take into account mirroring/rotated parts with the VAB changes. I want to know exactly what this string value is supposed to be set to, or what the relevant code object is so I can pull it thataway. I have a strong suspicion it\'s something defined in the model heirarchy, but I just don\'t understand such things. Once I get some kind of readout on the tank contents, I\'ll put up the whole thing in this forum section for anyone who wants a concrete example of how to get the new module and resource stuff working. Whatever gets returned from part.FindModelTransform('model/obj_gimbal') not only is not null, but it actually works in my code for making the thing fly straight. What\'s very interesting about this, is that I\'m using C7\'s structural fuselage with that code. When exactly did fuselages get a obj_gimbal? It IS null, I just commented out my debug spammer. Now I have to figure out a valid string to pass into part.FindModelTransform, as I\'m trying to test Mu\'s ModuleEngineRocketLiquid that\'s in the KSP assembly, and his version doesn\'t have a fail fallback setup like mine does. Anyone have a good string to pass into a C7 fuselage to get the main transform?
-
[0.17] Electrical Energy Plugin | v1.5 (Oct 12)
kellven replied to Kreuzung's topic in KSP1 Mod Releases
The plugin loader doesn\'t take into account referenced plugins atm. Result is I have to recompile my plugins every time MechJeb updates. You probably have the same problem. -
[Plugin] [Part] [0.17] KIS Connect V7a [2Oct12] [MIT]
kellven replied to kellven's topic in KSP1 Mod Releases
It was a royal pain to get this to work in 0.15, but the joints were not fixed in the update either. Unfortunately, I\'m completely unable to reproduce the turning problem. I\'m going to have to think about it for a while. There should be absolutely nothing in here that could affect course, it\'s just modifying/replacing the joints, and I get all the values used from the pre-existing joints themselves. The only thing I can think of that could possibly be wrong is that something has a very unusual joint setup that\'s not on my exclusion list yet. -
Not sure if this helps, but there\'s also a static FlightGlobals.Vessels which should hold a List<Vessel> that you can get anywhere. Might also be easier to grab FlightGlobals.Vessels[0].rootPart.transform.position, and compare those values, once you find which one is your vessel. Just mentioned it, as those are all from statics, so you can pull the values from anywhere.
-
Module and Resource example
kellven replied to kellven's topic in KSP1 C# Plugin Development Help and Support
I\'m actually using real Earth values in everything still. The conversion was just from the density of 6:1 LOX:LH2 to the density of 'fuel' in the default KSP rocket tanks. I was worried that the 'amount' values in my fuel tanks were so small(the net thrust per tank is equal assuming you\'re using this engine/tank, and LiquidEngine1/FuelTank), and the fuel consumption in the engines is also tiny. The real problem with conversions is that Kerbin g is almost identical to Earth g, which would suggest a 1:1 ratio, but the actual planet, and all the rocket parts are smaller than Earth counterparts. And the metrics used to measure non-distance stuff is also out of scale. In other words, using the kg:KG conversion factor should give you something more like the 500 'amount' of fuel in a tank, instead of 4.45 total in my tank. Also should bring the engine consumption from 0.0713/s closer to LiquidEngine\'s 8/s. The tiny values from direct conversion don\'t seem to cause noticeable problems yet, so I just left the KerboGram/KerboLiter in to make it clear what\'s an earth measurement, and what\'s a KSP measure. -
Trying to work through the mechanics of the new plugin system. I built something resembling Mu\'s example of a generic rocket module, and resource tank. The code to make the tank display like an actual FuelTank isn\'t working yet, but it does appear to be actually doing what it\'s supposed to. This is built as 2 'Part' Parts, one as a dual chamber LOX/LH2 tank, the other as a LOX/LH2 rocket, both are using C7\'s structural fuselage as a base. I\'m posting this here in case anyone is having problems just getting the new system setup into something that works, and want\'s a concrete example to examine. http://dl.dropbox.com/u/74137297/KISModuleDemoV0.zip There\'s also an OOO spreadsheet I made for calculating all the fiddly conversions. May or may not be horribly inaccurate. -24May12- Newer code with tons of debug.logs for your parsing pleasure. Think the fuel tank at least works as it, but can\'t test it without the engine running properly. http://dl.dropbox.com/u/74137297/KFuelTank.cs http://dl.dropbox.com/u/74137297/KPropulsionBase.cs -11Jun12- I accidentally fed the code after midnight, then spilled water on it, and it\'s grown into a horde of angry gremlins. This is in no way suitable for public consumption yet, but I did manage to get several more things working in game, and it\'s actually flyable atm. https://dl.dropbox.com/u/74137297/KISModuleDemoV2.zip
-
Ok, I got Mu\'s 'generic reaction engine' example set up and (mostly) working (with dual fuel use/storage also), but there\'s something that\'s bugging me still. [KSPField] public string thrustVectorTransformName; thrustTransform = part.FindModelTransform(thrustVectorTransformName); if (thrustTransform != null && part.Rigidbody != null) { part.Rigidbody.AddForceAtPosition(-thrustTransform.forward * currentThrust, thrustTransform.position); } That\'s not what it looks like in my version, but there\'s potentially something there I can\'t figure, as I don\'t touch modeling 'art' stuff if I can avoid it. thrustVectorTransformName is supposed to be a string denoting 'something' that\'s got with part.FindModelTransform(thrustVectorTransformName) I assume this is done either to confuse us, or to take into account mirroring/rotated parts with the VAB changes. I want to know exactly what this string value is supposed to be set to, or what the relevant code object is so I can pull it thataway. I have a strong suspicion it\'s something defined in the model heirarchy, but I just don\'t understand such things. Once I get some kind of readout on the tank contents, I\'ll put up the whole thing in this forum section for anyone who wants a concrete example of how to get the new module and resource stuff working.
-
[v0.17] Damned Aerospace Version 1.5.3 + Crewtank
kellven replied to DYJ's topic in KSP1 Mod Releases
Indeed, the 'the upside-down parts' aren\'t just upside down, they\'re backwards too. One thrusts forward, one backward. Flipping a puller prop, and sticking it on your rear, makes you go backward, not forward. I suppose that\'s Japan\'s version of airbrakes. -
If you want a new Model, start here. If you want a part with different characteristics, start here. If you want a part to run new code, go learn C#, then start here. Not sorted by level of difficulty.