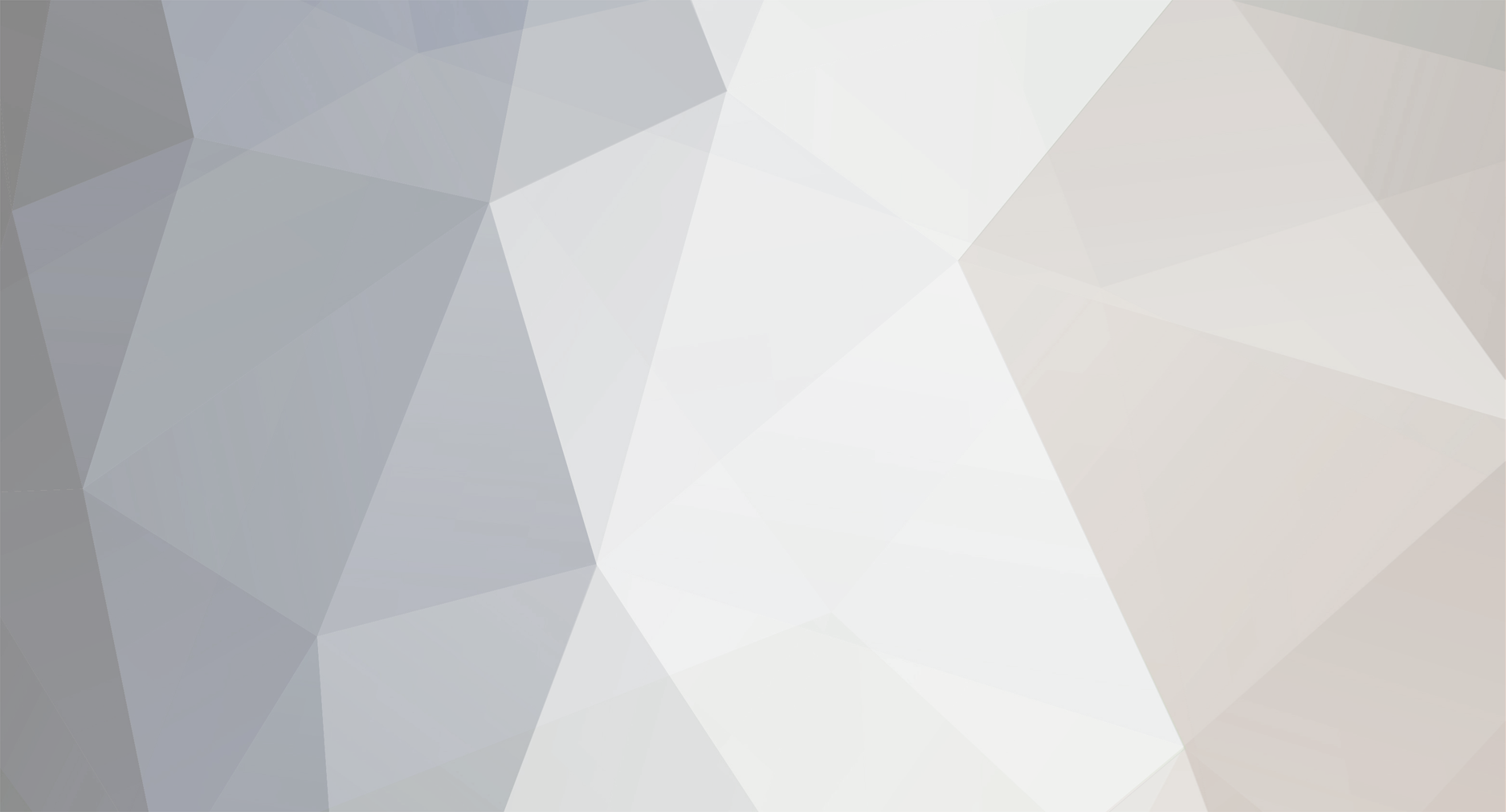
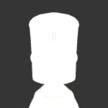
rejooh
Members-
Posts
7 -
Joined
-
Last visited
Content Type
Profiles
Forums
Developer Articles
KSP2 Release Notes
Bug Reports
Everything posted by rejooh
-
Flight Scene Render Texture
rejooh replied to rejooh's topic in KSP1 C# Plugin Development Help and Support
Thank you a lot! Works as expected. -
Hi, I'm currently trying to implement some more realistic science. Particularly SSTV (Slow-Scan Television), as it was used in the early US Space Program. To do so I need to render the Screen to a RenderTexture to create the SSTV audio signal. Concerning the rendering to the RenderTexture I found this topic and looking at the Romfarer source helped a lot. But I'm stuck with one problem. Every aspect of the flight scene renders well except the procedural quad sphere terrain. It's just plain white as can be seen in the following Texture2D's saved as .png. Thats my source: public class SSTVCamera { private static string[] GameCameras = { "Camera ScaledSpace", "Camera 01", "Camera 00" }; private static int ScaledSpaceCameraIndex = 0; private static int FarCameraIndex = 1; private static int NearCameraIndex = 2; private GameObject[] gameObjects; private Camera[] cameras; private RenderTexture renderTexture; public SSTVCamera(int width, int height, int depth, RenderTextureFormat format) { renderTexture = new RenderTexture(width, height, depth, format); renderTexture.Create(); LoadCameras(60, 557059); } public Texture2D RenderToTexture(Vector3 position, Vector3 aim, Quaternion rotation, bool planetary) { RenderTexture currentRenderTexture = RenderTexture.active; RenderTexture.active = renderTexture; if(planetary) { cameras[ScaledSpaceCameraIndex].transform.position = position; cameras[ScaledSpaceCameraIndex].transform.forward = aim; cameras[ScaledSpaceCameraIndex].transform.localRotation = rotation; cameras[ScaledSpaceCameraIndex].Render(); } else { cameras[NearCameraIndex].transform.position = position; cameras[NearCameraIndex].transform.forward = aim; cameras[NearCameraIndex].transform.localRotation = rotation; cameras[FarCameraIndex].transform.position = position; cameras[FarCameraIndex].transform.forward = aim; cameras[FarCameraIndex].transform.localRotation = rotation; cameras[ScaledSpaceCameraIndex].transform.rotation = cameras[NearCameraIndex].transform.rotation; cameras[ScaledSpaceCameraIndex].Render(); cameras[FarCameraIndex].Render(); cameras[NearCameraIndex].Render(); } Texture2D texture = new Texture2D(renderTexture.width, renderTexture.height); texture.ReadPixels(new Rect(0, 0, renderTexture.width, renderTexture.height), 0, 0); RenderTexture.active = currentRenderTexture; return texture; } private void LoadCameras(float fov, int cullingMask) { gameObjects = new GameObject[GameCameras.Length]; cameras = new Camera[GameCameras.Length]; for(int i = 0; i < GameCameras.Length; i++) { LoadCamera(GameCameras[i], ref gameObjects[i], ref cameras[i]); } cameras[ScaledSpaceCameraIndex].fov = fov; cameras[FarCameraIndex].fov = fov; cameras[NearCameraIndex].fov = fov; cameras[FarCameraIndex].cullingMask = cullingMask; cameras[NearCameraIndex].cullingMask = cullingMask; } private void LoadCamera(string name, ref GameObject gameObject, ref Camera camera) { gameObject = new GameObject(); gameObject.name = name + " " + gameObject.GetInstanceID(); camera = gameObject.AddComponent<Camera>(); camera.CopyFrom(FindCamera(name)); camera.targetTexture = renderTexture; camera.enabled = false; } private Camera FindCamera(string name) { foreach(Camera camera in Camera.allCameras) { if(camera.name == name) return camera; } return null; } private void DestroyCameras() { foreach(GameObject gameObject in gameObjects) { GameObject.Destroy(gameObject); } } } } Any help is greatly appreciated.
-
kOS Mapping Software After I overcame some problems, my mapping program finally does the job it's supposed to do. The program basically just converts latitude / longitude coordinates to screen coordinates. It afterwards checks whether altitude - alt:radar is higher than 1000m or 1km and prints "*" to the screen if that's the case. Here are two screenshots. One I've taken shortly after the program started, one taken after 3 hours of 4x physical time warp. For now, it is only possibly to map celestial-bodys that don't have an atmosphere because the orbit needs to be really low for the radio altitude to work. I used a circual 7,5 by 7,5km orbit around minmus. After looking into the code, you might quickly realize that converting floating point numbers into integers for the print at(x,y) function was another major problem. The function doesn't convert the numbers on it's own so I had to the job manually, which makes the program really slow, but it seems to be the only way for now. I also tried using faster time warps to increase the mapping speed, but the radar altitude doesn't seem to work using it either. I'm going to finish the map of minmus for now. Have fun with the program! set longscale to 48 / 360. set latscale to 31 / 180. clearscreen. print "--------------------------------------------------" at (0, 1). print "--------------------------------------------------" at (0, 34). print "-180 0 180" at (0, 35). until 1 = 2 { set height to altitude - alt:radar. set screenx to 24 + (longitude * longscale). set screeny to 17 - (latitude * latscale). print "MAPPING " + body + " - CURRENT ELEVATION: " + height at(0, 0). if height > 1000 { if screenx < 50 { set rscreenx to 50. } if screenx < 49 { set rscreenx to 49. } if screenx < 48 { set rscreenx to 48. } if screenx < 47 { set rscreenx to 47. } if screenx < 46 { set rscreenx to 46. } if screenx < 45 { set rscreenx to 45. } if screenx < 44 { set rscreenx to 44. } if screenx < 43 { set rscreenx to 43. } if screenx < 42 { set rscreenx to 42. } if screenx < 41 { set rscreenx to 41. } if screenx < 40 { set rscreenx to 40. } if screenx < 39 { set rscreenx to 39. } if screenx < 38 { set rscreenx to 38. } if screenx < 37 { set rscreenx to 37. } if screenx < 36 { set rscreenx to 36. } if screenx < 35 { set rscreenx to 35. } if screenx < 34 { set rscreenx to 34. } if screenx < 33 { set rscreenx to 33. } if screenx < 32 { set rscreenx to 32. } if screenx < 31 { set rscreenx to 31. } if screenx < 30 { set rscreenx to 30. } if screenx < 29 { set rscreenx to 29. } if screenx < 28 { set rscreenx to 28. } if screenx < 27 { set rscreenx to 27. } if screenx < 26 { set rscreenx to 26. } if screenx < 25 { set rscreenx to 25. } if screenx < 24 { set rscreenx to 24. } if screenx < 23 { set rscreenx to 23. } if screenx < 22 { set rscreenx to 22. } if screenx < 21 { set rscreenx to 21. } if screenx < 20 { set rscreenx to 20. } if screenx < 19 { set rscreenx to 19. } if screenx < 18 { set rscreenx to 18. } if screenx < 17 { set rscreenx to 17. } if screenx < 16 { set rscreenx to 16. } if screenx < 15 { set rscreenx to 15. } if screenx < 14 { set rscreenx to 14. } if screenx < 13 { set rscreenx to 13. } if screenx < 12 { set rscreenx to 12. } if screenx < 11 { set rscreenx to 11. } if screenx < 10 { set rscreenx to 10. } if screenx < 9 { set rscreenx to 9. } if screenx < 8 { set rscreenx to 8. } if screenx < 7 { set rscreenx to 7. } if screenx < 6 { set rscreenx to 6. } if screenx < 5 { set rscreenx to 5. } if screenx < 4 { set rscreenx to 4. } if screenx < 3 { set rscreenx to 3. } if screenx < 2 { set rscreenx to 2. } if screenx < 1 { set rscreenx to 1. } if screenx < 0 { set rscreenx to 0. } if screeny < 35 { set rscreeny to 35. } if screeny < 34 { set rscreeny to 34. } if screeny < 33 { set rscreeny to 33. } if screeny < 32 { set rscreeny to 32. } if screeny < 31 { set rscreeny to 31. } if screeny < 30 { set rscreeny to 30. } if screeny < 29 { set rscreeny to 29. } if screeny < 28 { set rscreeny to 28. } if screeny < 27 { set rscreeny to 27. } if screeny < 26 { set rscreeny to 26. } if screeny < 25 { set rscreeny to 25. } if screeny < 24 { set rscreeny to 24. } if screeny < 23 { set rscreeny to 23. } if screeny < 22 { set rscreeny to 22. } if screeny < 21 { set rscreeny to 21. } if screeny < 20 { set rscreeny to 20. } if screeny < 19 { set rscreeny to 19. } if screeny < 18 { set rscreeny to 18. } if screeny < 17 { set rscreeny to 17. } if screeny < 16 { set rscreeny to 16. } if screeny < 15 { set rscreeny to 15. } if screeny < 14 { set rscreeny to 14. } if screeny < 13 { set rscreeny to 13. } if screeny < 12 { set rscreeny to 12. } if screeny < 11 { set rscreeny to 11. } if screeny < 10 { set rscreeny to 10. } if screeny < 9 { set rscreeny to 9. } if screeny < 8 { set rscreeny to 8. } if screeny < 7 { set rscreeny to 7. } if screeny < 6 { set rscreeny to 6. } if screeny < 5 { set rscreeny to 5. } if screeny < 4 { set rscreeny to 4. } if screeny < 3 { set rscreeny to 3. } if screeny < 2 { set rscreeny to 2. } if screeny < 1 { set rscreeny to 1. } if screeny < 0 { set rscreeny to 0. } print "*" at(rscreenx, rscreeny). } }
-
Hello everyone, I'm currently trying to map the surface of minmus to the console. By simply converting latitude / longitude coordinates to screen coordinates and subtracting alt:radar from altitude. Everything expect the print at function works fine. print at(x,y) doesn't seem to accept values that aren't integers. But the coordinates aren't. Now, I need to know whether there is any way of converting floating point numbers to integers or apply floating point values to the print at(x,y) function. Thanks for your help, rejooh.
-
- please delete -
-
I watched the video a few times but i am not able to find an explanation for the black stripes, I followed it step by step.
-
Good evening, I have a problem concerning texturization of my models with Unity3D. After saving the model and baking its texture with Cinema 4D I import model and texture with Unity and the former neat texture has suddenly black stripes, which become less showy when I resize the textures to higher resolutions. (For example 2048x2048 or 4096x4096). They also disappear when zooming in. Here is a picture, I hope you have an idea how to fix the problem: Thanks for your help, rejooh.