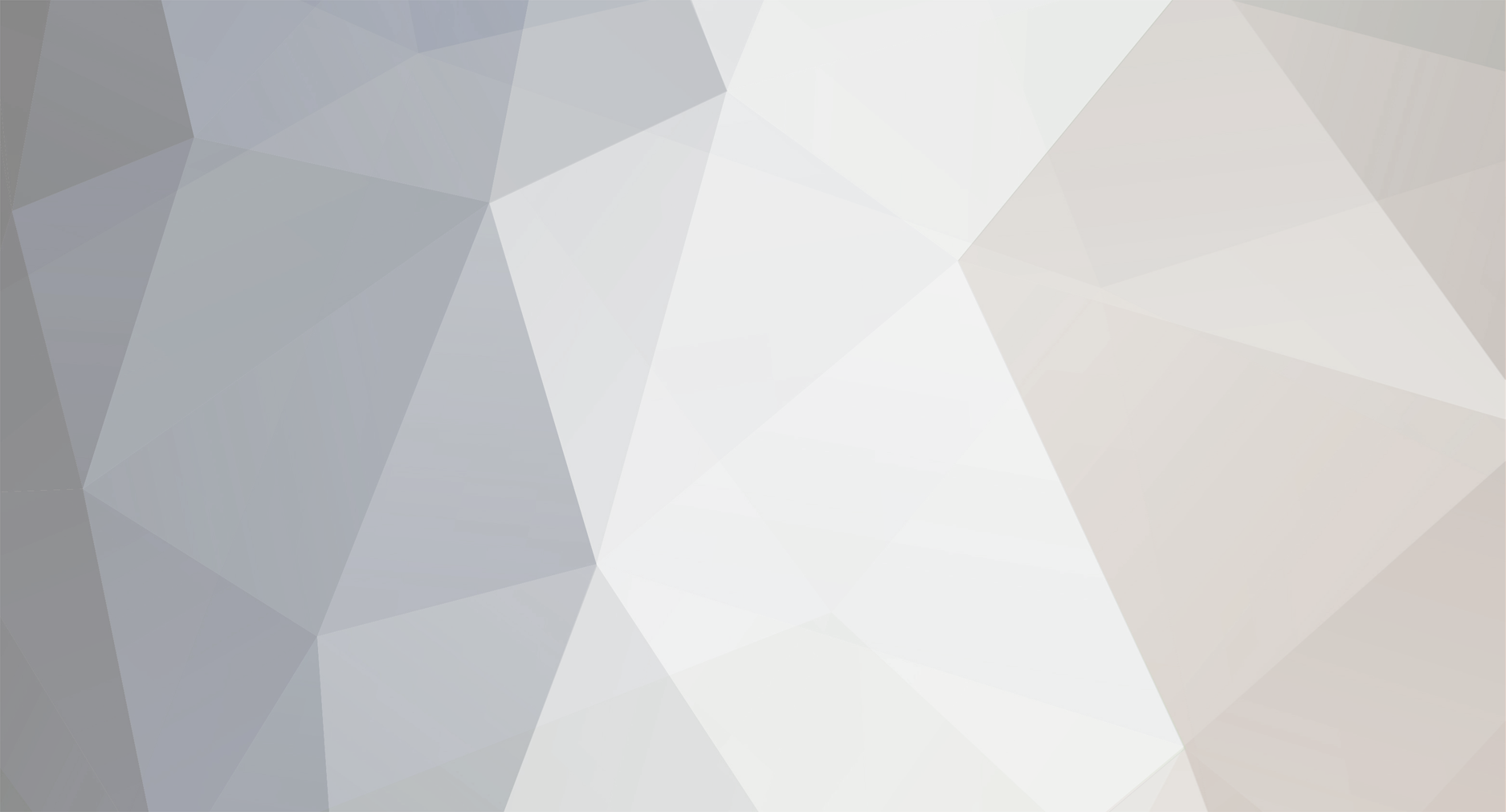
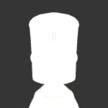
DMagic
Members-
Posts
4,180 -
Joined
-
Last visited
Content Type
Profiles
Forums
Developer Articles
KSP2 Release Notes
Everything posted by DMagic
-
An early build is available for download and testing now, get it on GitHub. The core functionality appears to be working, but there are several features missing, in addition to the list of planned features in the first post. Blizzy78's toolbar is not currently supported, there is no ability to re-size the window, or any of the UI content. There is no indication for completed contract parameters. Strategy modifier amounts are not shown. There is no support for the VAB/SPH. Let me know if anyone runs into bugs trying this out.
-
[KSP 1.8.1] SCANsat [v19.1] -- Dev version [February 20, 2020]
DMagic replied to DMagic's topic in KSP1 Mod Development
I guess that's what I get for trying to maintain strict version control over this sort of thing. I forgot to update the hard-coded version number used at startup to check if a valid SCANsat version is installed... I'll see about fixing that, and maybe adding in the tracking station orbits and a stand-alone landing target system that can be used instead of the MechJeb system. -
[KSP 1.8.1] SCANsat [v19.1] -- Dev version [February 20, 2020]
DMagic replied to DMagic's topic in KSP1 Mod Development
It's added to the list. Nothing changed from the previous version for MechJeb targeting. Is this a sandbox game? If not there might be something wrong with the way I'm checking to see if the Landing Guidance module has been unlocked. Does it work with a new vessel, or after saving and loading? -
[KSP 1.8.1] SCANsat [v19.1] -- Dev version [February 20, 2020]
DMagic replied to DMagic's topic in KSP1 Mod Development
What version of MechJeb are you using? It's entirely possible that this won't work with the dev builds. -
While trying to find good colors for my new CapCom mod I realized I was almost randomly picking things from the huge XKCDColors list. There are 954 colors in there and only the names to go by when trying to choose which one. So I made this simple in-game UI to show each color, print its name, show a few variations, and print the RGB values. You can drag the window and scroll through the available colors, there is no way to close it. You can change which scene it shows up in by changing the KSPAddon startup value in the code shown below. Download the compiled plugin from DropBox. Unzip and put the file anywhere in the GameData folder. For the code below all that you need to do is setup a project with references to KSP's Assembly-CSharp and UnityEngine. /* License: Public Domain * * By DMagic - 2015 */ using System; using System.Collections.Generic; using System.Reflection; using UnityEngine; namespace XKCDColorViewer { [KSPAddon(KSPAddon.Startup.MainMenu, true)] class XKCDColorPrintout : MonoBehaviour { Rect windowRect = new Rect(50, 50, 600, 600); Vector2 scroll = new Vector2(); GUIStyle title, fonts, fontStyle, rgbValue; Dictionary<string, Color> xColors = new Dictionary<string, Color>(); List<KeyValuePair<string, Color>> sortedColors = new List<KeyValuePair<string, Color>>(); bool loaded; private void Start() { assignColors(); } private void OnGUI() { if (!loaded) { title = new GUIStyle(GUI.skin.label); title.fontSize = 14; title.alignment = TextAnchor.MiddleLeft; title.wordWrap = false; fonts = new GUIStyle(title); fonts.alignment = TextAnchor.MiddleCenter; Texture2D blackBackground = new Texture2D(1, 1); blackBackground.SetPixel(1, 1, Color.black); blackBackground.Apply(); fonts.normal.background = blackBackground; fonts.fontSize = 16; fontStyle = new GUIStyle(title); fontStyle.fontStyle = FontStyle.Bold; fontStyle.alignment = TextAnchor.MiddleCenter; Texture2D whiteBackground = new Texture2D(1, 1); whiteBackground.SetPixel(1, 1, Color.white); whiteBackground.Apply(); fontStyle.normal.background = whiteBackground; rgbValue = new GUIStyle(title); rgbValue.fontSize = 12; loaded = true; } windowRect = GUI.Window("xkcdColors".GetHashCode(), windowRect, drawWindow, new GUIContent("XKCD Colors")); } private void drawWindow(int id) { Rect r = new Rect(2, 20, 595, 570); Rect rView = new Rect(0, 0, 575, 30540); scroll = GUI.BeginScrollView(r, scroll, rView); Rect label = new Rect(4, 2, 140, 28); int i = 0; foreach (var c in sortedColors) { i++; if (label.yMin >= (scroll.y - 20) && label.yMax <= (scroll.y + windowRect.height - 30)) { title.normal.textColor = c.Value; fonts.normal.textColor = c.Value; fontStyle.normal.textColor = c.Value; rgbValue.normal.textColor = c.Value; string s = c.Key; GUI.Label(label, i + ": " + s + ": ", title); label.x += 140; label.width = 110; GUI.Label(label, "Font Size 16 ", fonts); label.x += 120; label.width = 115; GUI.Label(label, "Font Style Bold", fontStyle); label.x += 125; label.width = 160; GUI.Label(label, string.Format("R: {0:N3}, G: {1:N3}, B: {2:N3}", c.Value.r, c.Value.g, c.Value., rgbValue); } label.y += 32; label.x = 4; label.width = 140; } GUI.EndScrollView(); GUI.DragWindow(); } private void assignColors() { Type xkcd = typeof(XKCDColors); var properties = xkcd.GetProperties(BindingFlags.Static | BindingFlags.Public); foreach (var prop in properties) { try { Color c = (Color)prop.GetValue(null, null); string s = prop.Name; if (xColors.ContainsKey(s)) continue; xColors.Add(s, c); } catch { print("Failed to assign a color value"); continue; } } foreach (var p in xColors) sortedColors.Add(p); sortedColors.Sort((a, => a.Key.CompareTo(b.Key)); } } }
- 1 reply
-
- 1
-
-
[KSP 1.8.1] SCANsat [v19.1] -- Dev version [February 20, 2020]
DMagic replied to DMagic's topic in KSP1 Mod Development
That should be doable in the tracking station. Doing so in the Space Center raises the problem of not having any simple way to select a vessel to show, and displaying multiple orbits is not really feasible. -
WIP: CapCom V0.3 Download on KerbalStuff Download on GitHub Version 0.3 is available for download now. Updated for KSP 1.0. I also realized that Backspace is the default action group key for the Abort sequence , so I changed the cancel/decline key to delete. Most of the features I have planned have been implemented. These include: Keyboard shortcuts available Up/Down arrows used to move through current contract list Left/Right arrows used to switch to different contract lists Enter to accept an offered contract Delete to decline or cancel a contract [*] Contract sorting options available Difficulty Funds, Science, or Reputation rewards Agency [*] Vertical window re-sizing is available using the re-size handle in the lower-right [*] Window position and size is persistent [*] Contract parameter status is indicated by a status icon [*] Agency info can be displayed by clicking on the agency flag [*] Strategy modifiers are shown in the rewards section [*] All settings persistent across all scenes and save files External config file saved in the GameData/CapCom folder Option to hide the nonsense mission briefing Option to hide mission notes by default; expand with the blue note icon Option to show warnings when declining or canceling a contract Option to use Blizzy78's toolbar Reassign all keyboard shortcuts Planned features include: Numerous UI improvements Correctly show strategy modifiers when no base reward is present Option to use KSP-style UI skin Original post below: One of my pet peeves in KSP is how so many scene changes are required during the course of playing. Since every scene change requires saving and loading everything in your save file, which can get really big when you start piling on addons, this can be very slow. Buildings like the R&D center, the astronaut complex, or the strategy building are OK, since you don't normally need to interact with these during a mission. But the Mission Control building is something you might need to get to while in flight, or even in the tracking station. I've created a working backbone for a Mission Control UI that can be accessed from the Space Center, Tracking Station, or while in flight. It tracks all offered, active, and complete contracts, and adds any newly offered contracts to its list. The basic idea is to create something similar to the Mission Control center (along with a few improvements, like sorting, hiding those nonsense briefings, etc...) that you can access anywhere. I'm not sure if it will work properly in the VAB/SPH, since vessels and Celestial Bodies aren't loaded in that scene. Source for the project can be viewed on GitHub. The project is released under the MIT license.
-
[1.8.x+] Waypoint Manager [v2.8.1] [2019-10-22]
DMagic replied to nightingale's topic in KSP1 Mod Releases
With a few tweaks SCANsat should be able to show custom waypoints just fine. Right now things might get a messy because the waypoint indicators are all indistinguishable unless you mouse over them (the standard waypoint icons and their associated experiment icons are too big), but I'm thinking that I could change the dot in the middle of the SCANsat waypoint icon to share its color with the FinePrint waypoint icon, that should help a little. -
[1.8.x] DMagic Orbital Science: New Science Parts [v1.4.3] [11/2/2019]
DMagic replied to DMagic's topic in KSP1 Mod Releases
All magnetic survey contracts will revert to default mission settings. The eccentricity requirement will be set to 0.2, inclination to 20, and the duration to 100 days (Kerbin days I think, it should handle the conversion to Earth days properly since it's defined by the number of seconds, but I haven't tested it). The science collection objectives won't be affected, if you've already completed them they will stay that way. All of the orbital objectives will also reset to being incomplete, you'll have to visit each vessel to restart the timer for each contract (if you have multiple vessels completing a survey just visit each one once). So unless you have a contract with a time of less than 100 days before it expires you should be fine. And if you have surveys that have been accepted, but you haven't started yet, they'll basically be switched to easy mode. -
This is one of those things that's been on the list for a long time, but I'm in no hurry to get to it. The KSP UI isn't quite as compact as the Unity UI, so everything would have to be adjusted again. Contracts Window + uses a lot of manual positioning of UI objects, changing to the KSP skin would likely require re-positioning of all of those objects (twice, since changing the UI scale also requires adjusting the position of basically everything) to make sure they stay compact and aligned correctly. The window is designed to stay open all of the time and not take up more space than necessary, so I make it as compact as possible and try not to draw UI objects unnecessarily (ie. not drawing rewards when the window isn't expanded). This means I can't use Unity's automatic layout system and have to position most objects manually, meaning specifying exactly which pixel each object is positioned at. Doing that four times is not something I look forward to.
-
[1.8.x+] Waypoint Manager [v2.8.1] [2019-10-22]
DMagic replied to nightingale's topic in KSP1 Mod Releases
You can't get the exact coordinates of the waypoints out of SCANsat, but you can mouse over them and get pretty close, along with the name, more so if you zoom in on them. I tested them with Anomaly Surveyor waypoints and they worked fine, but I'm not sure if they will work with custom waypoints. What do you do with the Contract reference for custom waypoints? Is it left null? If that's the case they won't show up at all in SCANsat. Otherwise, anything attached to an active contract will show. MechJeb landing guidance integration is actually very easy (assuming you don't mind using a small shim plugin, if you do mind that's another case...), as it's simple to plug in to the current MechJeb core and add a landing target; most of the complexity in SCANsat comes from the other way around, watching for a new landing target assigned by MechJeb. -
[KSP 1.8.1] SCANsat [v19.1] -- Dev version [February 20, 2020]
DMagic replied to DMagic's topic in KSP1 Mod Development
A minor fix is up on GitHub. Slope and biome color selections are now persistent, these will complain in the debug log and reset to defaults upon the first load. The zoom map properly resets when opening again from the big map. -
[KSP 1.8.1] SCANsat [v19.1] -- Dev version [February 20, 2020]
DMagic replied to DMagic's topic in KSP1 Mod Development
I see what the problem is. I'll hold off a bit to see if anything else comes up, but it's an easy fix; it's affecting biome colors too. -
[KSP 1.8.1] SCANsat [v19.1] -- Dev version [February 20, 2020]
DMagic replied to DMagic's topic in KSP1 Mod Development
That's what I want. I intentionally leave some of this stuff a little vague and don't have the detailed descriptions and tutorials that the release thread has because I want to know what needs explaining (and because the gifs take forever to make...). -
[KSP 1.8.1] SCANsat [v19.1] -- Dev version [February 20, 2020]
DMagic replied to DMagic's topic in KSP1 Mod Development
The zoom map does not automatically sync with the big map. You can zoom in/out, or re-center it without it updating to the big map's current settings. I figured there were a lot of situations where you might want to have a different map type, resource overlay, etc... in the zoom map. Click the zoom scale indicator in the center of the top menu of the zoom map to re-sync everything (there's a tooltip for it, if you've turned them off). You can also right-click on the big map somewhere to open the map again; there is a bug in that though, it won't reset the resources. The slope colors should persistent through anything that involves saving, they work basically the same as biome colors. As for the transparent colors, Unity doesn't understand non-square textures, at least as far as I know, so when you click somewhere outside of the wheel, but within the texture you'll get transparent colors. I might put some kind of gray background just to make it clear what's going on there. -
[KSP 1.8.1] SCANsat [v19.1] -- Dev version [February 20, 2020]
DMagic replied to DMagic's topic in KSP1 Mod Development
Trajectories seems to have a nice, friendly API for this sort of thing, so I can probably add this without much trouble. What I want to do first is add landing selection independent of MechJeb. So you could select a landing site on the zoom map and have it show on the map and on the actual planet in map view (and maybe in the flight scene too); I'll probably just borrow MechJeb's method for doing that and add some options to the settings menu to toggle MechJeb/Trajectories integration if they are available. Different colors could probably be used to differentiate between landing targets associated with different addons. -
[KSP 1.8.1] SCANsat [v19.1] -- Dev version [February 20, 2020]
DMagic replied to DMagic's topic in KSP1 Mod Development
New version is up, get it on GitHub. It contains several minor bug fixes, configurable slope map colors, and MechJeb landing guidance interaction. Check the first post for a full change log. -
[KSP 1.8.1] SCANsat [v19.1] -- Dev version [February 20, 2020]
DMagic replied to DMagic's topic in KSP1 Mod Development
Keeping the window on top? As far as I know that's a Unity GUI problem, that kind of thing happens all of the time when you have different windows on top of each other. I've never really looked into it though. -
[KSP 1.8.1] SCANsat [v19.1] -- Dev version [February 20, 2020]
DMagic replied to DMagic's topic in KSP1 Mod Development
I've fixed some of the issues from the previous build for the upcoming version. Waypoint data shouldn't get lost when changing scenes while the map is still open, I've included an Active Texture Management config to prevent it from altering SCANsat's icon textures, and I've fixed a few other minor things. I've also added a color selector for the useless slope maps. These maps use two sets of colors, one for "low" slope values and another for "high" values; I'll probably label the color selection window a bit better. A much more exciting addition is the ability to display and control MechJeb landing guidance targets. Any targets selected using the MechJeb interface will show up on the map of that planet. MechJeb targets are handled together with FinePrint waypoints, so they are both toggled on/off the same way. You can also select a landing target through SCANsat by clicking on a location in the new zoom window. Target selection is activated by clicking the MechJeb target icon in the top bar of the zoom window. If you are in map mode you'll see the new target displayed on the planet surface, the same way you do while selecting targets through MechJeb. There are still a few kinks to work out, but mostly this works very simply. Everything is handled through a separate, small plugin, since MechJeb is too complicated to handle through reflection. The MechJeb target icon will only show up on the zoom map when you have MechJeb installed, your current vessel has a MechJeb module (it doesn't have to be the actual MechJeb part, any part with the MechJeb part module added will count), and if the current map target planet matches your vessel's current planet. -
[KSP 1.8.1] SCANsat [v19.1] -- Dev version [February 20, 2020]
DMagic replied to DMagic's topic in KSP1 Mod Development
Not likely. It's probably ATM, I'm not really sure how the configs for that work, but I think they may have to be set to not touch the textures in the SCANsat/Icons folder, the same goes for any DDS converter, since Unity needs to be able to directly access that color wheel texture. I'll try to find a way around that, since it's really annoying to have to rely on another addon to not screw up mine. Edit: Yep it's an ATM problem. It looks like there are two things that need to be fixed. The current SCANsat ATM config looks like this: ACTIVE_TEXTURE_MANAGER_CONFIG { folder = SCANsat enabled = true OVERRIDES { SCANsat/Icons/* { compress = true mipmaps = false scale = 1 max_size = 0 } } } It needs to look like this: ACTIVE_TEXTURE_MANAGER_CONFIG { folder = SCANsat enabled = true OVERRIDES { SCANsat/Icons/.* { compress = true mipmaps = false scale = 1 max_size = 0 make_not_readable = false } } } It is very important to note the missing . in the folder location, without it ATM is just ignoring the override settings. The make_not_readable flag is needed to keep the color wheel icon available to the CPU so it can grab the pixel color when you click on it. That seems to work for both basic and aggressive ATM. -
[KSP 1.8.1] SCANsat [v19.1] -- Dev version [February 20, 2020]
DMagic replied to DMagic's topic in KSP1 Mod Development
That's likely to be either some kind of read-access issue or some texture fiddling plugin screwing around with textures that it shouldn't. -
[1.8.x] DMagic Orbital Science: New Science Parts [v1.4.3] [11/2/2019]
DMagic replied to DMagic's topic in KSP1 Mod Releases
I also tried to make the distinction between the two much clearer. In the latest version they all say "systems check" or something like that, and the actual science collection button says "collect data" or something along those lines. Think of it as a very small scale movement. All of these parts are very configurable. If you don't want the animation toggle to display you can just turn it off the in the part's config file. A handful of lines control the visibility of these options. None of these affect the actual data collection button. showStartEvent = false //Set this to false to prevent the start animation from showing showEndEvent = false //This is automatically turned off for the rover parts and anything else that uses one-way animations showToggleEvent = true //This is the line used by most of the probe parts that have extended and retracted settings showEditorEvents = true //This turns on or off the VAB/SPH options to play the animation -
[KSP 1.8.1] SCANsat [v19.1] -- Dev version [February 20, 2020]
DMagic replied to DMagic's topic in KSP1 Mod Development
Okay, a new release is up on GitHub. It fixes the broken sandbox/science mode and adds waypoints to IVA maps. -
[KSP 1.8.1] SCANsat [v19.1] -- Dev version [February 20, 2020]
DMagic replied to DMagic's topic in KSP1 Mod Development
Is this a sandbox or science mode save? I guess eventually I'll stop assuming everyone is using career mode, since this is the second time I've been bitten by this in the past week... It's a simple, one-line fix. I'll update with this and the IVA waypoints in a minute.