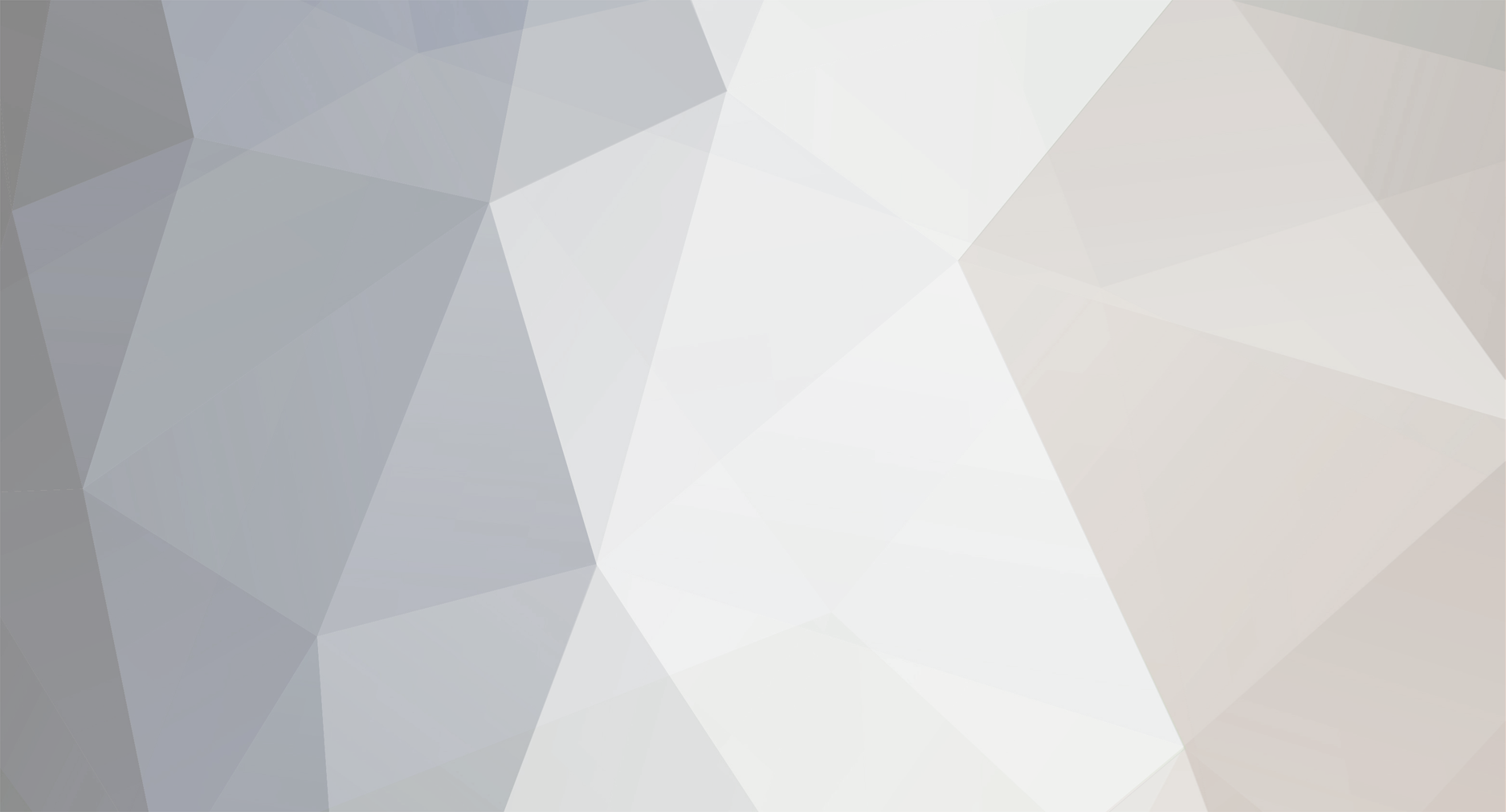
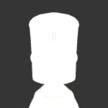
Dunbaratu
Members-
Posts
3,857 -
Joined
-
Last visited
Content Type
Profiles
Forums
Developer Articles
KSP2 Release Notes
Everything posted by Dunbaratu
-
[0.90] Magic Smoke Industries Infernal Robotics - 0.19.3
Dunbaratu replied to sirkut's topic in KSP1 Mod Releases
I hope people don't mind if I post this here. It's really about the kOS Scriptable Autopilot mod mostly, BUT it's about progress on making kOS interface with other mods, and the showcase mod it's interfacing with is Infernal Robotics: (I posted this a few days ago but it was in the wrong thread by mistake.) -
Okay thanks for the replies. I'll start looking into how to make the change. One of the reasons it's ugly is that my logic currently is this to decide if the laser hits something: FIRST, try Physics.RayCast(). If that finds a hit on a terrain polygon or a ship part, then quit right there and return that, we're done. SECOND, if Physics.RayCast() failed to find a hit, there might still be a hit far away because planetary terrain that's far from the camera isn't really fully rendered into polygons. It's sort of "holographic" in the sense that RayCasts pass through it. Therefore when Physics.RayCast() finds no hit, fall back on a numeric iterative solver algorithm that takes sample points along the ray to see when the ray first goes below the pqsController's reported terrain altitude for that spot, and where it does, iteratively narrow down with tighter and tighter sample points until the exact spot where it went underground is found. As you can see that fallback algorithm in the second part is WAAAY too expensive to be doing inside FixedUpdate(). But the decision as to whether or not to do it depends on the result of the raycast in FixedUpdate. Since the ratio of number of FixedUpdate()'s to Update()'s is not 1:1, it may get slightly messy. Do I have a guarantee that Update() and FixedUpdate() share the same thread, meaning that my Update() method can't be interrupted partway through by my FixedUpdate() method, or visa versa? If so, that would make it easier because then I can just remember the state of the most recent RayCast from FixedUpdate() and just look at it in Update().
-
Unity's Physics.RayCast() doesn't seem very reliable when it comes to aiming the ray at ship parts. It works fine for ground terrain or KSC buildings, but when aimed at ship parts it works intermitently. Note, this is NOT (I don't think) a problem with the layers because it hits the SAME part for one or two Update()'s, then in the next update it passes right through it, then in the next Update() it hits it again, then a few updates later it starts passing through it again, and so on. The problem seems more pronounced when the ship is in motion (I have a laser on the ship aiming at another part of the same ship to measure the distance between them as an Infernal Robotics part moves them farther apart, and when the ship takes off the erroneous "penetrating" raycasts start happening more frequently than when it was on the ground.) I've tried googling about it and it seems to be that people have trouble with Raycast hitting moving parts and it has something to do with the exact timing of when you call Physics.RayCast(). I find people claiming it's better if you put Physics.RayCast() calls in FixedUpdate() instead of Update(), but that seems like a drastic measure as FixedUpdate is a precious resource I should avoid using, and the rest of my logic about what to do with the raycast is too "expensive" for FixedUpdate() and needs to stay in Update() so it's going to make the programming more complex if I putthe raycasts in FixedUpdate(). Does anyone have good experience with Physics.Raycast() hitting ship parts and know whether or not this claim is really true (that you need to put the raycasts in FixedUpdate() for them to work right?) That's a major undertaking to make that change for my mod and it would be horrible if I went through the work only to find its a red herring. Unity's own docs for RayCast never mention this, but given the state of Unity's documentation, the fact that it never mentions it is hardly conclusive.
-
[1.3] kOS Scriptable Autopilot System v1.1.3.0
Dunbaratu replied to erendrake's topic in KSP1 Mod Releases
Just a bit of teaser to tide people over while we keep plugging away at kOS v0.15 : The two mods being used in the example are LaserDist and Infernal Robotics, but the principle is that it generically works on any mod provided you spend some time at the terminal printing out various exploratory fields to find out what the mod calls things: -
Okay so I actually wrote a script to try using this and I ran into trouble because Unity's Physics.RayCast doesn't work right apparently if you try to call it from Update() to detect an object in motion. (Apparently the object gets moved asynchronously and the collider for it is still at the previous frame's position). The effect is that when trying to detect an object in motion, LaserDist will sometimes pass right through it. I never noticed before because all my testing was on stationary objects. I'll still have to look into this and see if I can reproduce it more reliably. It's intermittent.
-
Update. v0.5 Faster Numeric approximater, laser varying animation, kOS usage doc ------------------------------------------------------------------------- Fixed: * CPUHOG slider had no effect. Now it does. New: * Sped up numeric approximation algorithm by using body.pqsController.radiusMin and body.pqsController.radisuMax to set upper and lower bounds on the possible distances at which a terrain hit can occur. * [Added kOS usage doc](https://github.com/Dunbaratu/LaserDist/blob/master/doc/Using_in_kos.md) (Note, usage in kOS doesn't work yet. This is just the description of how it will be used when it's ready. * Added simple laser animation effect that makes the beam picture fluctuate and flicker a bit. I now figure it's good enough that I'd move this from the work in progress forum to the releases forum, but I'm just waiting for the kOS support before I do that so the two are ready together.
-
[1.3] kOS Scriptable Autopilot System v1.1.3.0
Dunbaratu replied to erendrake's topic in KSP1 Mod Releases
Marianoapp had added some code to the project that looks like it's designed to allow one SCS unit to tell another SCS unit to run a command on it, but it's not really tested or done. If you want to see it, look at src/Interprocessor. I'd prefer it if instead of actually directly having control to run something on the other SCS unit, there was just a messaging system in place to send/get messages between CPUs and let the CPUs decide what to do about the messages. -
KSP doesn't load the terrain polygons into Unity at distances longer than about 10km or so. Raycasts only work when close up. I want this to also work from orbit too. The only way I've found to make it work from far away from the ground is making a numeric approximation algorithm that takes sample points along the ray and queries the pqsController to find the altitude at those locations, using a dissection algorithm to find where the ray first becomes underground, then recursively checking where in THAT slice the sub-slice is where it first goes underground, then check the sub-sub-sub slices, and so on until the error is less than the epsilon I've chosen (which is 2 meters, as this is only used from very long distances where that's acceptable). KSP does have a raycast method in the pqsController but all it does is a sphere intersection with the ray and the body's default zero-altitude radius, ignoring the actual terrain. The first thing my code does is attempt the direct raycast hoping the polygons are actually loaded and it's a nearby hit. It's only when that fails but the ray does intersect the body's radiusMax sphere that I then drop down into the more expensive numeric approximation algorithm.
-
The relevant thing not being said here, and it's important as its the only thing I was ever confused about, is the fact that not only is the child PQS used for oceans, but that this system is used exclusively for oceans and never for generating flat land. The same type of child PQS data structure could have easily been used to make flat bottom land, so it's not an obvious assumption to make that it is never done that way. Although mentioning that the oceans are also a separate PQS of their own does give rise to an alternate algorithm I can use that's more robust than assuming this system will only ever be used for oceans from now and forever. Instead of trying to track whether the planet has oceans, I just ask for all the PQS objects attached to it, and look for the intersect with whichever one of them is nearest, not caring whether it's an ocean or not. That way the code might continue working if in a future release SQUAD decides to change the assumptions and start using the child PQS system for solid ground.
-
Then why would anyone think that telling me oceans can't be solid constituted an answer? Someone who thinks I thought oceans can be solid didn't understand me. No, Greys said that an assumption I wasn't even making was wrong. The actual assumption I was making is that both oceans and flat bottom land are modeled using the same smooth sphere superimposition method, not that oceans themselves are solid. It only became clear eventually further down in the thread that in addition to the strawman of my assumption referred to in Grey's post being wrong, that the assumption I actually made was wrong too. The claim that it was clear from Grey's post alone is wrong. It's simpler than you described, which is why it's confusing. Talking about the red herrings of whether oceans can be solid deflected the topic from the actual point where my assumption was wrong, which was about whether or not solid land uses the same sphere intersection system oceans use. "The system that superimposes a sphere above the negative altitude terrain is only used by oceans and oceans alone. It was not used to build flat land like you see on Minmus. Minmus's pqsController actually returns zero for real for the terrain height in those locations". <- That's the simple answer that addresses the point where I actually had the wrong assumption, and it's not quite how it was being expressed. Telling me that "my" assumption that oceans can be solid is wrong is addressing a different assumption than the one I was making. I never thought that the flat land on Minmus was actually an ocean. Anyway, thanks for clearing it up.
-
Get component? The only suggestion I saw mentioned CelestialBody.ocean, not get component. Neither would I, and I didn't. I didn't claim that Kerbin's superimposed sphere was a solid one, but that Minmus's was, under the assumption that Minmus had such a superimposed sphere. No, not really. That answer just told me that Minmus had no ocean, which I already knew and I never claimed it had an ocean, nor a "hard sea", as it was put. What I assumed was that the method of superimposing a smooth sphere with the bumpy pqsController terrain height was a technique that wasn't limited to oceans only. In other words, I was envisioning the cases breaking down this way: Does Body have a superimposed sea-level sphere? / \ / \ no yes (i.e Mun) | Case 1 | | Is it an ocean? / \ / \ / \ no yes (i.e. Minmus) (i.e. Kerbin) Case 2 Case 3 It sounds like what you're trying to say is that Case 2 doesn't exist. So telling me oceans aren't solid doesn't really tell me anything because by the time I'm calling it an "ocean" I'm already thinking Case 3 and I'm already thinking "well of course they're not solid. The kinds of spheres that are solid aren't going to be called "oceans". That's why the previous answers were not clear. They assumed I was thinking of it this way: Does Body have an ocean? / \ / \ no yes (i.e Mun) | Case 1 | | Is it liquid? / \ / \ / \ no yes (i.e. Minmus) (i.e. Kerbin) Case 2 Case 3 When that's not what I was thinking, nor how I was trying to describe it.
-
I didn't say it had an ocean. I said it has a mathematical "sea level" and it does. It's the altitude that is returned as "zero" in the altimeter, as opposed to the radar altitude to the actual terrain. The point is that on some worlds, when the pqsController tells you terrain exists that has negative altitude (the radius returned is less than the planet's default sphere radius, it's "sea level"), you actually can land on it, but on other words you cannot because there is an actual object to collide with a sea level that you hit first. I don't care whether that object is liquid and therefore can be called an "ocean" or not. I only care whether that object exists on the body in question in one form or another, be it liquid or not. On some worlds, while the pqsController will return terrain at negative altitude, you can't actually get to it because the world is made up of an intersection between the terrain altitude returned by pqsController AND a smooth sphere at altitude zero, and whichever of the two of them is higher is the effective lowest you can go. On other bodies, you actually can go land at a negative altitude when the pqsController tells you there is negative altitude terrain there, because the "sea level" altitude isn't populated with anything. I deliberately avoided calling the sea level object an "ocean" because for the purpose of this query I don't care whether it's made of liquid or not. I only care whether it's going to be there or not, interfering with trying to reach the negative terrain. As for Minmus, I was assuming it worked just like Kerbin - an intersection of a plain spherical object with a bumpy terrain object, but in minmus's case the plain spherical object was solid instead of liquid. That's why I left the door open for the possibility that the same intersection with a plain sphere that was used for oceans might be used for dry land too. I don't KNOW whether that's the case or not. All I want to know is "what's the reliable way to detect whether or not there will be that superimposed sphere in place or not?" If it turns out that this technique is only used when CelestialBody.ocean is true, and it's never used for dry land, then that's my answer. I didn't know whether or not that superimposing-a-sphere-with-the-terrain technique was reserved purely for oceans and nothing else. Is it?
-
[1.3] kOS Scriptable Autopilot System v1.1.3.0
Dunbaratu replied to erendrake's topic in KSP1 Mod Releases
I seem to recall from another mod I was making that when you model parts in the Unity editor you need to make them such that the rocket-up direction is the +Y axis, but that for steering purposes in game, KSP refers to rotations that presume the initial starting point is the +Z axis... It may be this difference that is the problem. Perhaps all we have to do is that for part transforms, make their default roation the +y rather than the +z and it will come out right... I'll have to see, but it will take a few days to get to diagnosing this further. -
Some bodies, like Kerbin and Eve and Laythe, have a sea level sphere that is real and made of liquid. Some bodies, like Minmus, have a sea level sphere that is real and made of solid ground. But other bodies, like the Mun, have a sea level sphere that is entirely invisible and permeable and not "really" there. The Mun has one or two craters that actually allow you to go below sea level and land under the "sea", with no ill effects. Is there something in the API I can query that can tell me the difference? Given a CelestialBody or a CelestialBody's pqsController, what is the way I can tell if its sea level is a "real" one or not? This matters because I'm trying to work out what the distance to the ground or water using a homemade raycats algorithm, given the body's pqs controller, and on some planets, when the pqsController tells me the ground altitude is negative at a certain location, that means I can actually go below sea level and land down there, and on other planets, it means I cannot ever reach that ground and I'll hit the sea level sphere first. Knowing the difference matters and I don't know how to tell other than just hardcoding it per body which way (which seems inelegant).
-
[1.3] kOS Scriptable Autopilot System v1.1.3.0
Dunbaratu replied to erendrake's topic in KSP1 Mod Releases
Okay now that I can see what you're talking about the problem is understandable. -
[1.3] kOS Scriptable Autopilot System v1.1.3.0
Dunbaratu replied to erendrake's topic in KSP1 Mod Releases
This section of the post contains no images, making it almost impossible to follow what you're talking about. I think the spoiler tag is hindering the images. -
[1.3] kOS Scriptable Autopilot System v1.1.3.0
Dunbaratu replied to erendrake's topic in KSP1 Mod Releases
Actually seeing the string "((System Built-in flight control updater))" says a LOT. It says it happened while calculating the lock expression used by either LOCK STEERING or LOCK THROTTLE, which happens asynchronously in the background all the time and therefore isn't *directly* from where the current executing code happens to be. That's why it doesn't give the current line number. -
[1.3] kOS Scriptable Autopilot System v1.1.3.0
Dunbaratu replied to erendrake's topic in KSP1 Mod Releases
Can you show us where within the code the complaint occurs (what line does it highlight and say is the problem? Or does it not say?) - - - Updated - - - There might be, but I don't know of it if there is. I did make a highlight file for VIM a long while ago, but it needs to be updated once some of the newer syntax gets shaked out. But at any rate, it was for Vim, not Notepad++. -
Is there any progress on 0.25 support (the main thread first page still says 0.24.2 so I assume I can't use it with 0.25 yet)?
-
In principle that should work. It only rounds to the nearest 2 meters when its trying to solve numerically against the unloaded terrain that's very far away. When it actually has real polygons of nearby objects to collide with it gives much more accurate results. The bigger problem you might run into is the course-grain timeslicing of kOS itself. It might be hard to make that engine go faster than a limited speed because kOS won't run fast enough to keep up with your queries if you want to make an engine that goes something like 1000 rpm. but keep speed down lower and in principle it should work.
-
[1.3] kOS Scriptable Autopilot System v1.1.3.0
Dunbaratu replied to erendrake's topic in KSP1 Mod Releases
This URL had some ideas I had a long time ago, but there have always been bigger things to do taking up my limited time developing kOS that kept putting it off: https://github.com/KSP-KOS/KOS/issues/106#issuecomment-43851394 If you're curious as to how to take any raw vector and get what it's 3 coord components would have been in a different basis system, remember that you can always do this with just dot products. Build the 3 basis unit vectors in raw coords, then to get a raw coords vector's component in that basis direction, just dot-product it with that basis vector. This video describes that process in more detail starting at about 2 min, 30 seconds into the video: It's not an ideal solution but after a few fixes to how vector rotations worked, it became a possible solution, which is why that idea in the URL I posted never got implemented yet. The severity of the problem was reduced by the existence of the workaround. Basically, when comparing two problems, making things that are impossible to do *at all* become possible ends up taking higher precedence than making something that is possible but hard into something that is easy. That sort of "triage" thinking has been dominating my contributions to the code of late due to the fact that there's so few of us making code contributions, and a way more stuff to do than people to do it.