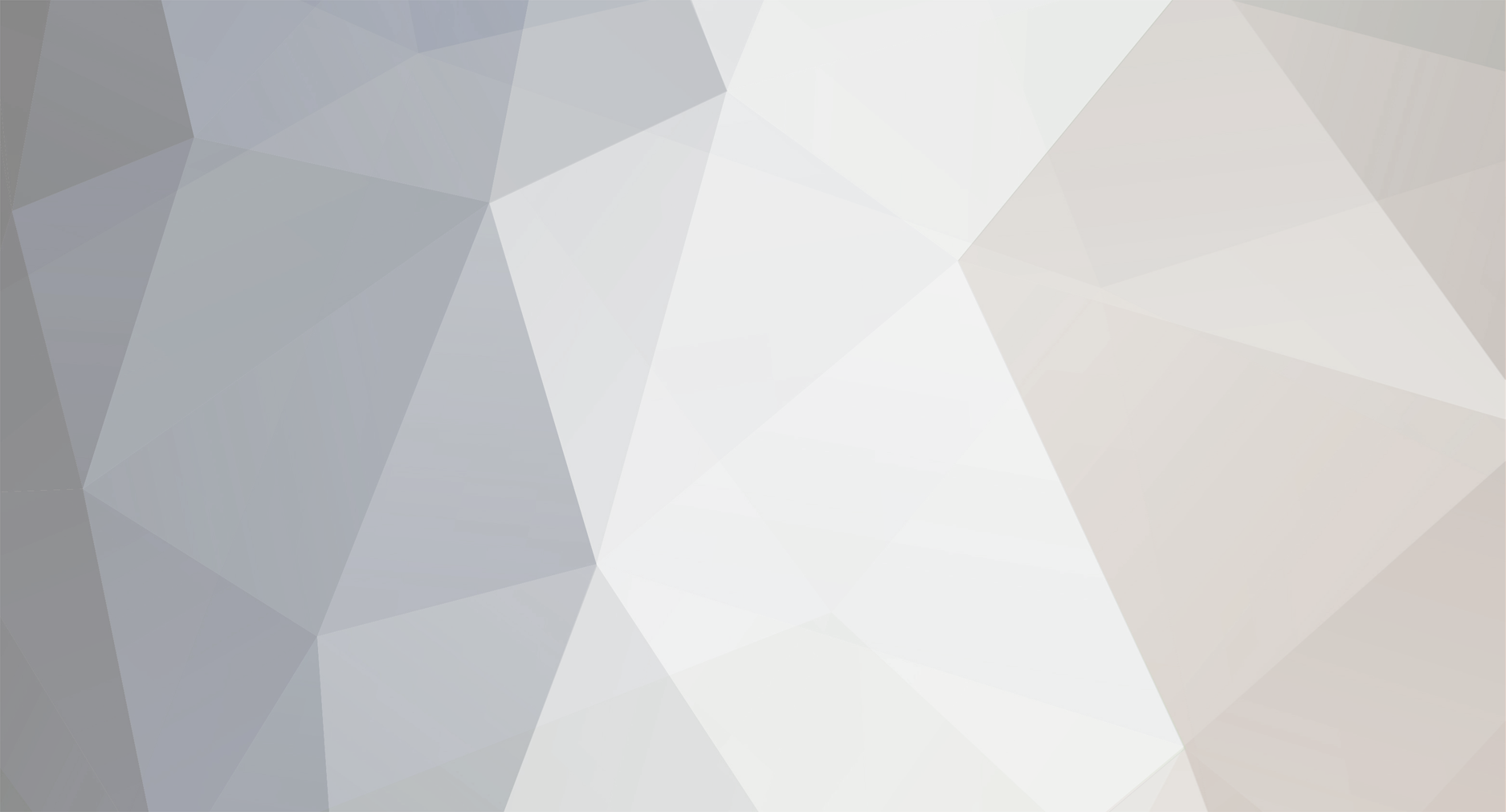
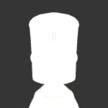
xEvilReeperx
Members-
Posts
894 -
Joined
-
Last visited
Content Type
Profiles
Forums
Developer Articles
KSP2 Release Notes
Everything posted by xEvilReeperx
-
Spawning a part
xEvilReeperx replied to FiresThatBurn's topic in KSP1 C# Plugin Development Help and Support
Those are actually MonoBehaviour methods. You just define them and then Unity will call them when appropriate -
Having some means of figuring out which science you still need did get requested a few times. I decided not to incorporate it into ScienceAlert because I was working on another mod, BiomeOverlay, that would be more useful for that. I was in the middle of coming up with a more intuitive interface for it when Stuffâ„¢ happened and my free time suffered. It's still in the pipeline though.
-
KSPAddon - publishing values
xEvilReeperx replied to Felger's topic in KSP1 C# Plugin Development Help and Support
That's just a counting variable that refers to the current AutoGimbal instance being iterated over. You could rewrite that part as a foreach loop and it'd be equivalent. I just happen to find it slightly more readable with the ForEach is all -
Great! That narrows down the problem immensely. Something is going wrong with the biome map construction and the observer update loop gets stuck because of it. I've already ironed out two logic fails that would prevent reports from showing up if just the right conditions occurred thanks to the exception info provided by your logs.
-
KSPAddon - publishing values
xEvilReeperx replied to Felger's topic in KSP1 C# Plugin Development Help and Support
Yes, essentially. It just occurred to me there's another way you could go about this as well: Instead of the PartModule finding the MonoBehaviour, you could have the MonoBehaviour tell the PartModule which MonoBehaviour it'll be accessing. This might even be the superior solution if there's a good chance you'll be dealing with multiple manager style objects later, since you needn't modify your PartModule at all: public class AutoGimbal : PartModule { public AutoGimbalManager manager; public override void OnUpdate() { Log.Debug("target = " + manager.rotationTarget); } } [KSPAddon(KSPAddon.Startup.EveryScene, false)] public class AutoGimbalManager : MonoBehaviour { public string rotationTarget = "Mun"; void Start() { FlightGlobals.Vessels.ForEach(vessel => { vessel.FindPartModulesImplementing<AutoGimbal>().ForEach(gimbal => { gimbal.manager = this; }); }); } } I don't know why that didn't occur to me earlier -
KSPAddon - publishing values
xEvilReeperx replied to Felger's topic in KSP1 C# Plugin Development Help and Support
There are two easy ways. The first one is a static property: public class AutoGimbal : PartModule { public override void OnUpdate() { Log.Debug("target = " + AutoGimbalManager.instance.rotationTarget); } } [KSPAddon(KSPAddon.Startup.EveryScene, false)] public class AutoGimbalManager : MonoBehaviour { public string rotationTarget = "Mun"; void Start() { instance = this; } public static AutoGimbalManager instance { get; private set; } } The other way is to go looking for the right type directly. It's straightforward but you don't want to go looking through the entire scene every frame, so caching the result is important: public class AutoGimbal : PartModule { AutoGimbalManager manager; void Start() { manager = FindObjectOfType<AutoGimbalManager>(); } public override void OnUpdate() { Log.Debug("target = " + manager.rotationTarget); } } [KSPAddon(KSPAddon.Startup.EveryScene, false)] public class AutoGimbalManager : MonoBehaviour { public string rotationTarget = "Mun"; } -
Whoops, misread. Right, that's why I was explaining why you need the second half of that line. You don't need to scan the children; if the GO you hit is included in a Part's hierarchy, the Part component will always be found in the hit GO or an ancestor Yes, walking the tree upwards is exactly what Component.GetComponentInParent does. That's why I was trying to point out that you need more than just Part.FromGO, you need the hit.transform.gameObject.GetComponentInParent<Part>(); part of that line too because Part.FromGO will fail in both of your examples
-
Well, a "Part" is really just a component attached to a GameObject (the documentation you referred to really botched the description there), plus potentially some supporting components (like PartModules). The second portion of that line is because the GameObject containing the collider hit by your ray might not necessarily have a Part component attached to it if the Part it's related to has a hierarchy of colliders. Consider one of the deployable solar panels or wheels, or any user-created part that has multiple primitive Box/Sphere/etc colliders.
-
It's not a class. Here's a minimal example to get you started: using System; using UnityEngine; namespace TestPlugin { [KSPAddon(KSPAddon.Startup.Flight, false)] public class ScreenshotTest : MonoBehaviour { void Update() { if (GameSettings.TAKE_SCREENSHOT.GetKeyDown()) Debug.Log("A screenshot was just taken!"); } } } If you have your references set up correctly, that will compile and you can start to tweak it as you like
-
Is that your exact code? Assuming you added a reference to IR in your project (and it sounds like you have), you'll find MuMechToggle in MuMech, not InfernalRobotics. So, something like this: using MuMech; [...inside some object] var mmt = part.FindModulesImplementing<MuMechToggle>().First(); Or you can skip the using and specify it directly: var mmt = part.FindModulesImplementing<MuMech.MuMechToggle>().First();
-
There was a recent post that will be helpful to you. Once you have your RaycastHit info, you already have a GameObject entry into the hierarchy of whatever you hit. Let's say I want to find out if it's a part or the ground terrain (non-scaled space) that I've hit: LayerMask mask = (1 << 0) | (1 << 15); if (Physics.Raycast(ray, out hit, 10f, mask)) { if (hit.transform.gameObject.layer == 15) { // real terrain was hit } else { Part target = Part.FromGO(hit.transform.gameObject) ?? hit.transform.gameObject.GetComponentInParent<Part>(); if (target != null) Log.Write("we hit {0}", target.name); } } It would be useful to write a method that prints the components attached to the GameObject hierarchy you've hit. Mine's come in handy on many occasions
-
var just infers the type based on the right-hand side. That line is equivalent to ModuleReactionWheel rw = part.Modules.OfType<ModuleReactionWheel>().Single(); You inherit that from PartModule, along with a bunch of other stuff That's exactly what it does. part.Modules.OfType<ModuleReactionWheel>() returns an IEnumerable containing all ModuleReactionWheel instances found in part.Modules. Single() takes the first one, should there be more than one found No, that's just an alternative method. This line returns a List<DTMagnetometer> rather than IEnumerable. First() takes the first item in the list.
-
You need an actual instance of the target PartModule to work on. Say my PartModule is attached to a command pod and I want to know some info about the ModuleReactionWheel attached to it. Here are two ways to find a ModuleReactionWheel on the same part: var rw = part.Modules.OfType<ModuleReactionWheel>().Single(); rw = part.FindModulesImplementing<ModuleReactionWheel>().First(); Debug.Log(string.Format("ypr torque: {0}, {1}, {2}", rw.YawTorque, rw.PitchTorque, rw.RollTorque)); And since it's somewhat related, if I wanted to know about every ModuleReactionWheel on the vessel my part is attached to, you can easily find out with: vessel.FindPartModulesImplementing<ModuleReactionWheel>() If it's possible that your target PartModule doesn't exist on your part, use [single/First]OrDefault() instead of Single/First() and check for null before trying to work on it.
-
That's because ScenarioModules are created on a per-persistent file basis. That's how the actual Scenarios ingame are made; they're just save games that somebody has manually added a savegame-specific ScenarioModule to. The main menu isn't associated with any particular game session so it wouldn't make sense for one to be loaded there. If you did need to run code before game start, I'd probably go with a separate, non-ScenarioModule KSPAddon that persisted between scenes. Once the player has loaded up a game, you could do whatever it is you wanted: store some info you collected during the main menu into your ScenarioModule, call a function or set up some parameters, etc. Then kill it and run your logic as normal
-
You're going to smack your head on the desk in about five seconds when I bold some stuff for you So you're actually adding a ScenarioModule called TestScenarioModule to the game. Unluckily for you, the game has one and it apparently writes a test value to the persistent file and opens a popup to display it. Your ScenarioModule, TestSM, never runs so of course you never see any output
-
I was expecting to see some NRE spam in there. Just based on that log (although Blizzy's right, this log is missing the juicy stack traces) everything seems fine. It does narrow down the possibilities considerably however. I did notice one oddity that is probably unrelated but kind of concerning anyway: [LOG 15:21:20.409] [FLIGHT GLOBALS]: Switching To Vessel Tylpollo ---------------------- [LOG 15:21:20.410] setting new dominant body: Jool FlightGlobals.mainBody: Jool [LOG 15:21:20.411] ScienceAlert, BiomeFilter.OnDominantBodyChanged from Jool (CelestialBody) to Jool (CelestialBody) [LOG 15:21:20.412] ScienceAlert, BiomeFilter.OnDominantBodyChanged from Jool (CelestialBody) to Jool (CelestialBody) [LOG 15:21:20.413] ScienceAlert, BiomeFilter.OnDominantBodyChanged from Jool (CelestialBody) to Jool (CelestialBody) [LOG 15:21:20.414] ScienceAlert, BiomeFilter.OnDominantBodyChanged from Jool (CelestialBody) to Jool (CelestialBody) [LOG 15:21:20.500] PartHighlighter: Added highlight script to part 'radialDecoupler_4293155086' [LOG 15:21:20.535] PartHighlighter: Added highlight script to part 'radialDecoupler_4293154652' [LOG 15:21:20.567] PartHighlighter: Added highlight script to part 'radialDecoupler_4293154280' [LOG 15:21:20.601] PartHighlighter: Added highlight script to part 'radialDecoupler_4293153846' [LOG 15:21:20.663] PartHighlighter: Added highlight script to part 'radialDecoupler_4293152838' [LOG 15:21:20.678] PartHighlighter: Added highlight script to part 'radialDecoupler_4293152672' [LOG 15:21:20.754] PartHighlighter: Added highlight script to part 'liquidEngine_4293152218' [LOG 15:21:20.780] PartHighlighter: Added highlight script to part 'liquidEngine_4293151962' [LOG 15:21:20.407] Target vessel index: 66 vessel count: 71 Any chance you're running a custom version of PartHighlighter? It shouldn't be running at all in flight mode
-
I was in almost exactly the same position as you with ScienceAlert. I tried every way I could think of that didn't involve adding the PartModule to every prefab. Ultimately I failed and decided to design around the problem. In your case, I think Greys' solution is the best. Don't bother with MM; that's just an unnecessary dependency. The only gotcha is that you shouldn't forget that the vessel configuration can change, so you'll be needing some appropriate logic in OnVesselWasModified etc Edit: Also, there's the customPartData field you can use. It would be the most simple and straightforward solution, though of course there will be issues mixing your mod with any other that happens to also use the field
-
There seem to be some issues related to IDrawable and the latest update. I have users reporting incorrect toolbar positions and in some cases the GUI becomes nearly non-interactive if the toolbar is positioned such that the auto positioning code fails (typically when at the top of the screen, positioned between the altimeter and sides of the screen). Here is an example: The exact position will alternate on every right-click-open and in both cases something strange is going on because clicking buttons does nothing or (if the positioning is as the second picture) the drawable will suddenly snap to the wrong place. I want to say that the actual location checked for mouse input is lagging behind the displayed position, since you can sometimes manage to click buttons if you twitch your mouse fast enough to the right spot. 1.7.1 works fine though
-
Looking into it! Edit: This looks like an issue with the toolbar and how it now tries to avoid overlapping anything important. I'll let blizzy know. Can you guys confirm that the options window (right-click toolbar button) behaves very strangely as well? Edit again: strangely in certain locations*, like when the toolbar's auto positioning code fails and it ends up overlapping something
-
I like to keep a copy of my plugin directory with the source (so any related assets can be added to source control) so my post build event looks like this: set ksp=full_ksp_path_to_gamedata_here robocopy "$(ProjectDir)bin\$(Configuration)" "$(ProjectDir)GameData\$(ProjectName)" $(TargetFileName) robocopy "$(ProjectDir)GameData\$(ProjectName)" "%ksp%\$(ProjectName)" /E /xo REM a little hack because robocopy reports code 1 on file copy success REM build process will interpret non-zero as an error and report failed build set rce=%errorlevel% if %rce%==1 exit 0 if %rce%==2 GOTO ExtraFiles if %rce%==3 GOTO ExtraFiles if not %rce%==1 exit %rce% else exit 0 :ExtraFiles echo WARNING: Extra files in output dir exit 0 Then any time I work with any assets, I keep them in $(ProjectDir)GameData\$(ProjectName)\ and can edit them at will. Any changes will be moved into the KSP directory next time I rebuild. It's convenient, and I can delete any directory in my dev install as I like without worrying about accidentally losing something important