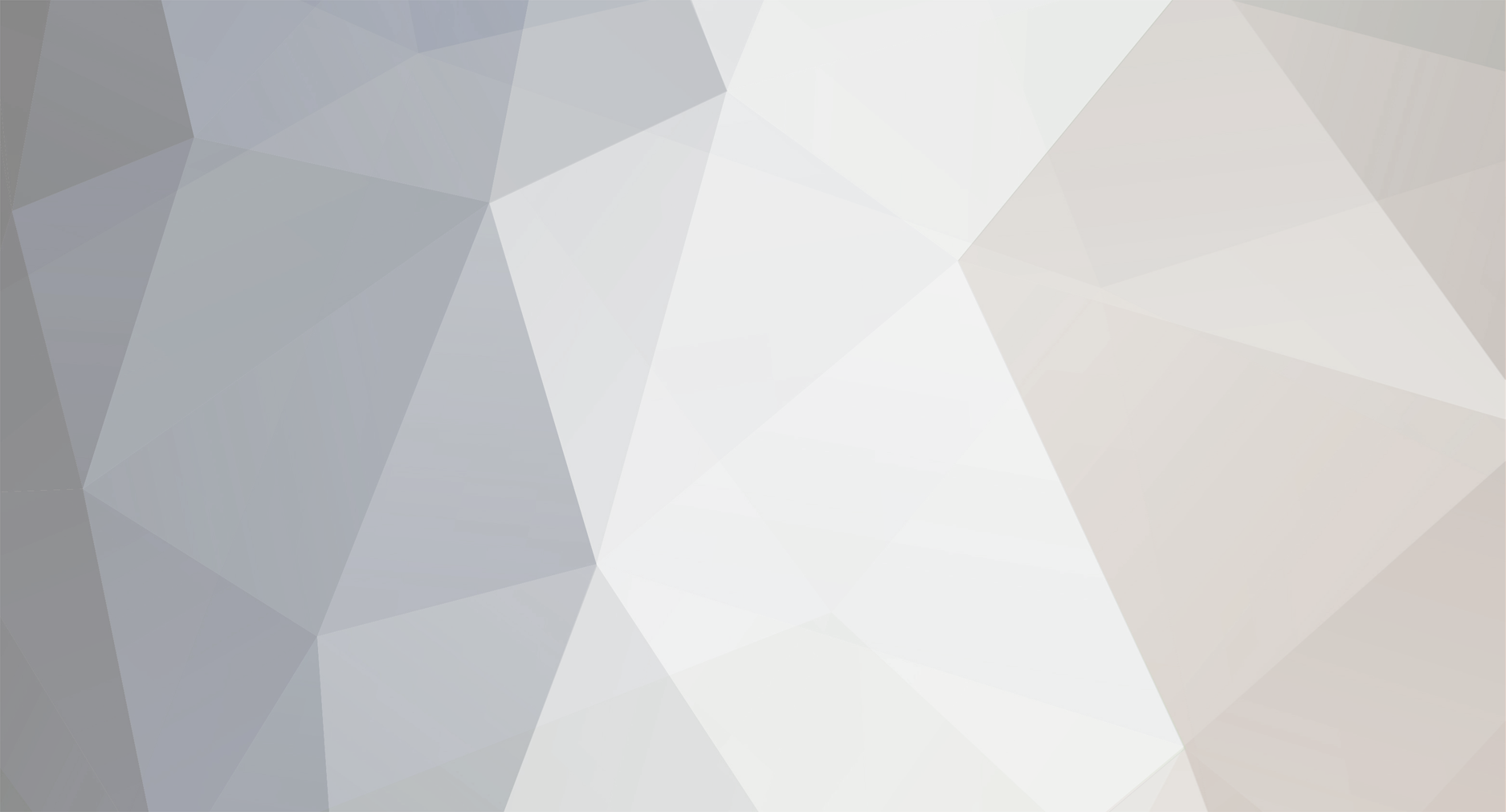
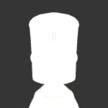
jmbon
Members-
Posts
13 -
Joined
-
Last visited
Reputation
0 NeutralProfile Information
-
About me
Curious George
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
-
Hello, I'm rather new to modding KSP and Unity in general. I am attempting to add fog and clouds to KSP and I have really no clue where to begin. I've searched Google high and low as well as the forums and all I could find is incomplete code snippets so small that they really don't help much. If someone has the time and knowledge, would you lend me a hand. Below is my code so far. I think I am on the right track with making a gameobject, assigning it's .maintexture to be my texture I choose, adding a mesh, creating vertices, triangles, etc. but I really have no idea if this is correct and if it is, where do I go now. using System; using System.IO; using System.Collections; using UnityEngine; using KSP; namespace Clouds { public class Clouds_Main : MonoBehaviour { bool debug_output = false; Texture2D white_clouds = null, storm_clouds = null; private float lastFixedUpdate = 0.0f, logInterval = 2.5f; Mesh light_cloud_mesh = new Mesh(); Mesh dark_cloud_mesh = new Mesh(); public Material light_cloud_material; public Material dark_cloud_material; GameObject dark_cloud_gameObject; GameObject light_cloud_gameObject; /* Caution: as it says here: http://docs.unity3d.com/Documentation/ScriptReference/MonoBehaviour.Awake.html, use the Awake() method instead of the constructor for initializing data because Unity uses Serialization a lot. */ /* Called after the scene is loaded. */ void Awake() { if (white_clouds == null) white_clouds = LoadPNG(@"C:\Program Files (x86)\Steam\SteamApps\common\Kerbal Space Program\GameData\Wind Mod\S_Cloud4.png"); // Load the S_Cloud4.png Icon image into memory if (storm_clouds == null) storm_clouds = LoadPNG(@"C:\Program Files (x86)\Steam\SteamApps\common\Kerbal Space Program\GameData\Wind Mod\D_Cloud1.png"); // Load the D_Cloud1.png Icon image into memory light_cloud_material.SetTexture(0, white_clouds); dark_cloud_material.SetTexture(1, storm_clouds); } /* Called next. */ void Start() { dark_cloud_gameObject.AddComponent<MeshRenderer>().material.mainTexture = dark_cloud_material.GetTexture(1); dark_cloud_mesh = dark_cloud_gameObject.AddComponent<MeshFilter>().mesh; dark_cloud_mesh.Clear(); dark_cloud_mesh.vertices = new Vector3[] { new Vector3(-0.5f, 0, -0.5f), new Vector3(0.5f, 0, -0.5f), new Vector3(0.5f, 0, 0.5f), new Vector3(-0.5f, 0, 0.5f) }; dark_cloud_mesh.uv = new Vector2[] { new Vector2(0, 0), new Vector2(0, 1), new Vector2(1, 1), new Vector2(1, 0) }; dark_cloud_mesh.triangles = new int[] { 0, 1, 2, 2, 3, 0 }; light_cloud_gameObject.AddComponent<MeshRenderer>().material.mainTexture = light_cloud_material.GetTexture(0); light_cloud_mesh = light_cloud_gameObject.AddComponent<MeshFilter>().mesh; light_cloud_mesh.Clear(); light_cloud_mesh.vertices = new Vector3[] { new Vector3(-0.5f, 0, -0.5f), new Vector3(0.5f, 0, -0.5f), new Vector3(0.5f, 0, 0.5f), new Vector3(-0.5f, 0, 0.5f) }; light_cloud_mesh.uv = new Vector2[] { new Vector2(0, 0), new Vector2(0, 1), new Vector2(1, 1), new Vector2(1, 0) }; light_cloud_mesh.triangles = new int[] { 0, 1, 2, 2, 3, 0 }; } /* Called every frame */ void Update() { } /* Called at a fixed time interval determined by the physics time step. */ void FixedUpdate() { if ((Time.time - lastFixedUpdate) > logInterval) { } } /* Called when the game is leaving the scene (or exiting). Perform any clean up work here. */ void OnDestroy() { } Texture2D LoadPNG(string filePath) // Converts a .png image into a BYTE array that Unity needs in order to display as a GUI image { Texture2D temp_texture = null; // Ensure the texture is always reset byte[] fileData; if (File.Exists(filePath)) { fileData = File.ReadAllBytes(filePath); temp_texture = new Texture2D(2, 2); temp_texture.LoadImage(fileData); //..this will auto-resize the texture dimensions. } else { if (debug_output) Debug.Log("[WIND MOD : Gui Draw] Icon file does not exist in location: " + filePath); } return temp_texture; } } } I would greatly appreciate any help you can give. Thanks in advance.
-
Hello, I'm rather new to modding KSP and Unity in general. I am attempting to add fog and clouds to KSP and I have really no clue where to begin. I've searched Google high and low as well as the forums and all I could find is incomplete code snippets so small that they really don't help much. If someone has the time and knowledge, would you lend me a hand. Below is my code so far. I think I am on the right track with making a gameobject, assigning it's .maintexture to be my texture I choose, adding a mesh, creating vertices, triangles, etc. but I really have no idea if this is correct and if it is, where do I go now. using System; using System.IO; using System.Collections; using UnityEngine; using KSP; namespace Clouds { public class Clouds_Main : MonoBehaviour { bool debug_output = false; Texture2D white_clouds = null, storm_clouds = null; private float lastFixedUpdate = 0.0f, logInterval = 2.5f; Mesh light_cloud_mesh = new Mesh(); Mesh dark_cloud_mesh = new Mesh(); public Material light_cloud_material; public Material dark_cloud_material; GameObject dark_cloud_gameObject; GameObject light_cloud_gameObject; /* Caution: as it says here: http://docs.unity3d.com/Documentation/ScriptReference/MonoBehaviour.Awake.html, use the Awake() method instead of the constructor for initializing data because Unity uses Serialization a lot. */ /* Called after the scene is loaded. */ void Awake() { if (white_clouds == null) white_clouds = LoadPNG(@"C:\Program Files (x86)\Steam\SteamApps\common\Kerbal Space Program\GameData\Wind Mod\S_Cloud4.png"); // Load the S_Cloud4.png Icon image into memory if (storm_clouds == null) storm_clouds = LoadPNG(@"C:\Program Files (x86)\Steam\SteamApps\common\Kerbal Space Program\GameData\Wind Mod\D_Cloud1.png"); // Load the D_Cloud1.png Icon image into memory light_cloud_material.SetTexture(0, white_clouds); dark_cloud_material.SetTexture(1, storm_clouds); } /* Called next. */ void Start() { dark_cloud_gameObject.AddComponent<MeshRenderer>().material.mainTexture = dark_cloud_material.GetTexture(1); dark_cloud_mesh = dark_cloud_gameObject.AddComponent<MeshFilter>().mesh; dark_cloud_mesh.Clear(); dark_cloud_mesh.vertices = new Vector3[] { new Vector3(-0.5f, 0, -0.5f), new Vector3(0.5f, 0, -0.5f), new Vector3(0.5f, 0, 0.5f), new Vector3(-0.5f, 0, 0.5f) }; dark_cloud_mesh.uv = new Vector2[] { new Vector2(0, 0), new Vector2(0, 1), new Vector2(1, 1), new Vector2(1, 0) }; dark_cloud_mesh.triangles = new int[] { 0, 1, 2, 2, 3, 0 }; light_cloud_gameObject.AddComponent<MeshRenderer>().material.mainTexture = light_cloud_material.GetTexture(0); light_cloud_mesh = light_cloud_gameObject.AddComponent<MeshFilter>().mesh; light_cloud_mesh.Clear(); light_cloud_mesh.vertices = new Vector3[] { new Vector3(-0.5f, 0, -0.5f), new Vector3(0.5f, 0, -0.5f), new Vector3(0.5f, 0, 0.5f), new Vector3(-0.5f, 0, 0.5f) }; light_cloud_mesh.uv = new Vector2[] { new Vector2(0, 0), new Vector2(0, 1), new Vector2(1, 1), new Vector2(1, 0) }; light_cloud_mesh.triangles = new int[] { 0, 1, 2, 2, 3, 0 }; } /* Called every frame */ void Update() { } /* Called at a fixed time interval determined by the physics time step. */ void FixedUpdate() { if ((Time.time - lastFixedUpdate) > logInterval) { } } /* Called when the game is leaving the scene (or exiting). Perform any clean up work here. */ void OnDestroy() { } Texture2D LoadPNG(string filePath) // Converts a .png image into a BYTE array that Unity needs in order to display as a GUI image { Texture2D temp_texture = null; // Ensure the texture is always reset byte[] fileData; if (File.Exists(filePath)) { fileData = File.ReadAllBytes(filePath); temp_texture = new Texture2D(2, 2); temp_texture.LoadImage(fileData); //..this will auto-resize the texture dimensions. } else { if (debug_output) Debug.Log("[WIND MOD : Gui Draw] Icon file does not exist in location: " + filePath); } return temp_texture; } } } I would greatly appreciate any help you can give. Thanks in advance.
-
Thanks for that. I already had the bool in the code, I just wasn't using it there. Putting it there solved that issue. Still trying to figure out how to add an image. If I figure it out, I'll post it here. Thanks again.
-
Hello, I'm relatively new to Unity still and I am having two issues that are GUI related. First, I am trying to set a .png image as the background for a GUI.button(rect, image). I just can not get the image to display as the background of the button. My second problem is that I am trying to use the same button to open another config UI for my mod so that players can enable and disable certain mod features. However, when I click the button (button appears even if the background image doesn't), my debug statement prints but the second UI doesn't draw to the screen. For the past several hours, I have searched forums, Github code (mainly Stage Recovery as it's code is commented and not a mess) and Unity 5.3 tutorials/examples/manual entries trying to figure out what I am doing wrong. Nothing has helped thus far. Hopefully someone here can spot my mistake. I greatly appreciate the help. public void OnDraw(int windowID) { draw_Icon_GUI(windowID); // GUILayout.Window(windowID, control_window_Rect, draw_Control_GUI, "Control", HighLogic.Skin.window); } void draw_Icon_GUI(int windowID) { Rect _Icon_Rect = new Rect(Screen.width - 40, 220, 40, 40); // Texture image = (Texture)Resources.Load("C:/Program Files (x86)/Steam/SteamApps/common/Kerbal Space Program/Resources/_symbol.png") as Texture; // <------- First attempt at displaying an image as the button background // GUI.skin.button.active.background = image; // <------- First attempt at displaying an image as the button background GUIContent image = new GUIContent("Click me", (Texture)Resources.Load("C:/Program Files (x86)/Steam/SteamApps/common/Kerbal Space Program/Resources/_symbol")); // <------- Third attempt at displaying an image as the button background if (image != null) { // Draw the background. // GUI.DrawTexture(wind_Icon_Rect, image); // <------- Second attempt at displaying an image as the button background } else Debug.Log("Bad texture: " + image); if (GUI.Button(wind_Icon_Rect, image)) { Debug.Log("[MOD : Gui Draw] Button was clicked."); // Prints when the button is clicked control_window_Rect = GUILayout.Window(windowID, control_window_Rect, draw_Control_GUI, "Control", HighLogic.Skin.window); // Doesn't execute ever. Worked before I started trying to make a button execute it. } } void draw_Control_GUI(int windowID) // Worked before I started trying to make a button execute it. { GUILayout.BeginVertical(); // toggle setup GUILayout.Label("Toggle", HighLogic.Skin.label); wind_toggle = GUILayout.Toggle(wind_toggle, "Toggle", HighLogic.Skin.toggle); // Slider setup hSliderValue = GUILayout.HorizontalSlider(Mathf.Round(hSliderValue), 0f, 10f); GUILayout.Label("Scaling = " + Mathf.Round(hSliderValue), HighLogic.Skin.box); // End the Groups and Area GUILayout.EndVertical(); GUI.DragWindow(); // GUI window is also having issues with not being draggable even when this section of code worked } Above is the code that handles my GUI drawing. OnGUI() is in another class but all id does is call OnDraw(int windowID). I put // <---- comments where I believe my code is having the issues. I greatly appreciate any help I can get. Thank you.
-
Hello, I am in the process of creating a new mod and I find myself stuck on correctly creating two separate GUI's. The first is very simple as it has a toggle, a horizontal slider and 2 labels all inside of a window and all automatically positioned by using GUILayout.*. The second GUI is actually a compass that I want to display in the game window. I know how to do the rotations for the needle but I don't have much of a clue as to how to begin. I've read through forums, Unity how-to's etc and while they helped somewhat with the first GUI, they offered no help on the second. Here is all of my GUI code: Main Class: GUI_Code.GUI_Draw gdraw = new GUI_Code.GUI_Draw(); private void OnGUI() { gdraw.OnGUI(1); } My GUI Class using UnityEngine; namespace GUI_Code { public class GUI_Draw : MonoBehaviour { public bool toggle = true; public float hSliderValue = 0.0F; public Texture2D icon; private Rect windowRect = new Rect(10, 30, 100, 90); // Texture2D compass; // compass image // Texture2D needle; // needle image // Rect compass_rect = new Rect(10, 10, 200, 200); // create a new rectangle at position (x = 1, y = 10) with size (x = 200, y == 200) // float angle; // angle to rotate the needle // Quaternion rot; // Vector3 axis; // but an axis variable must be provided as well public void OnGUI(int windowID) { // Make a background box GUI.Box(new Rect(10, 10, 150, 90), "1st GUI Menu"); // GUI.DrawTexture(compass_rect, compass); // Vector2 compass_widget_center = new Vector2(compass_rect.x + compass_rect.width / 2,compass_rect.y + compass_rect.height / 2); // find the compass center coordinates // Matrix4x4 svMat = GUI.matrix; // save gui matrix // GUIUtility.RotateAroundPivot(angle, compass_widget_center); // prepare matrix to rotate // GUI.DrawTexture(compass_rect,needle); // draw the needle rotated by angle // GUI.matrix = svMat; // restore gui matrix // Draw all of the buttons inside of the window windowRect = GUI.Window(windowID, windowRect, WindowFunction, "My Window", HighLogic.Skin.window); } public void WindowFunction(int windowID) { // Begin the singular Horizontal Group GUILayout.BeginHorizontal(); // Toggle setup wind_toggle = GUILayout.Toggle(toggle, "Toggle", HighLogic.Skin.toggle); GUILayout.Label("Toggle", HighLogic.Skin.label); // GUILayout.BeginVertical(); // Slider setup hSliderValue = GUILayout.HorizontalSlider(1f, 0f, 10f); GUILayout.Box("Wind Scaling = " + Mathf.Round(hSliderValue), HighLogic.Skin.box); // Compass setup // GUILayout.Label("Direction"); // End the Groups and Area // GUILayout.EndVertical(); GUILayout.EndHorizontal(); GUILayout.EndArea(); // This last thing checks whether the right mouse button or middle mouse button are clicked on the window. If they are, we ignore it, otherwise we GUI.DragWindow() // Calling that allows the window to be moved by clicking it (anywhere empty on the window) with the left mouse button and dragging it to wherever you want. if (!Input.GetMouseButtonDown(1) && !Input.GetMouseButtonDown(2)) GUI.DragWindow(); } } } The first GUI is the uncommented lines in OnGUI(int window ID). It has 2 major problems that I don't know how to correct. First, the window isn't draggable even though I make it draggable in the last line of OnGUI(int window). Second, in the Debug.Log, I am getting "[Exception]: InvalidOperationException: Operation is not valid due to the current state of the object" The second GUI is the commented out lines. I am trying to make a simple 2D compass similar to: How do I even go about setting this up and what's wrong with my first GUI? I'll keep looking through forums and Github code but so far, it's been dead ends. Thank you in advance. Also, if you know how to do it, I'd like both the 1st GUI and the compass to be attached to an icon at the right side of the screen. I am assuming that would be a 3rd GUI with just a toggle that did 1st_GUI.show() and compass.show() or 1st_GUI.hide() and compass.hide() (I know the syntax is wrong but you get the idea). Is this the correct way to do this?
-
Well crap. How would I tell if my craft were over the oceans biome or highlands biome? I know it's a noob question but without being able to do a logical on either a str compare or enum result, I'm temporarily in the weeds. I really do appreciate the help. Btw, huge fan of Orbital Science. It's one of my core mods where I don't even play KSP until Orbital Science is up and running for that version.
- 4 replies
-
- biome
- biome detection
-
(and 1 more)
Tagged with:
-
Thanks for the reply. Your second way is the one I was slowly heading towards. I really appreciate it. Is there a list of official biome names in the KSP API somewhere? I'm sure I can't do something like, if (biome.name == "my_biomeKSC") { // Do stuff } I've looked through the latest Scansat source code but couldn't find a list of official c# biome names, enums, etc. Thanks again.
- 4 replies
-
- biome
- biome detection
-
(and 1 more)
Tagged with:
-
Hello, I am trying to create my first addon for KSP and I am having problems detecting which biomes my active vessel is currently. I've looked through the API calls, class calls and some old code on Github but none of it has really helped. I feel like I am on the right track but hung up. Below is a snippet of my code where I am getting ready to detect the current biome my vessel is in. CelestialBody Body = new CelestialBody(); CBAttributeMapSO.MapAttribute[] biome = Body.BiomeMap.Attributes; I feel like my Body variable is the right route or maybe my FlightGlobals.activevessel. As far as I can tell, biome above just gives me a list of the current CelestialBody biomes, is that correct? In my code, I already have access to the Vessel class and the FlightGlobals class and my program is inheriting from : monobehavior. Any help or hints you can give would be greatly appreciated. Thanks.
- 4 replies
-
- biome
- biome detection
-
(and 1 more)
Tagged with:
-
Hello, I am currently working on creating my first mod for KSP and I find myself sort of hung up on UI creation in KSP. I followed the example given in but 1) I don't want to tie my UI to a specific part like the author of the above video does, I'd rather tie it to a button click in the game much like Kerbal Alarm Clock does. Once I am able to display it via in game buttonclick events, 2) I'd like a radial gauge widget to appear similar in shape to a compass. Are there any good examples of both of those you could point me to. I've looked through Github at example code but a lot of the code is a mess and almost none of it is commented. All of the tutorials like the one above are close but not quiet what I am looking for. I feel like I need to use Unity to create a UI gameobject and then import that gameobject into KSP but I am unsure if that is the right course and if it is, how to even begin doing that. Thank you in advance for your help.
-
I didn't know about the F12 functionality but it's the same as right clicking the variable, class, etc., and selecting 'Peek Definition'. See, I thought they were equivalent as well, hence me coming here after the forums, man pages, and online tutorials/examples didn't help. Anywho, when changing the B to lowercase, I got conflicting types from my program and KSPUtils.dll . I commented out my public delegate CallBack(int que, int onDraw); and it seems to have solved the current issue but now my UI window doesn't show up in KSP. using UnityEngine; //This module will create a new GUI window and button, on button press the part will explode, close the GUI, and be removed. namespace KSP_Wind_Display { public class Wind_GUI_Display : PartModule { Callback callback; private Rect window_pos = new Rect(); // New blank window private GUIStyle window_style, label_style; private bool has_init_styles = false; public override void OnStart(PartModule.StartState state) { base.OnStart(state); if (!has_init_styles) init_styles(); callback += onDraw; if (state == StartState.Flying || state == StartState.Landed || state == StartState.PreLaunch || state == StartState.Splashed) { RenderingManager.AddToPostDrawQueue(0, callback); Debug.Log("\n\n\n\nIt should have drawn the UI\n\n\n\n"); } } private void onDraw() // Start up the window { if (this.vessel == FlightGlobals.ActiveVessel) window_pos = GUILayout.Window(1, window_pos, onWindow, "Wind Direction", GUILayout.MinWidth(100)); } private void onWindow(int window_id) { GUILayout.BeginHorizontal(); GUILayout.Label("Width"); GUILayout.EndHorizontal(); GUI.DragWindow(); // Makes the window draggable } private void init_styles() { window_style = new GUIStyle(HighLogic.Skin.window); window_style.fixedWidth = 250.0f; label_style = new GUIStyle(HighLogic.Skin.label); label_style.stretchWidth = true; // stretches the label width to that of the window has_init_styles = true; } } } I suspect the problem is because 'callback' was a delegate where I casted onDraw to. Now that it's not really a delegate, I suspect that 'RenderingManager.AddToPostDrawQueue(0, callback);' isn't being called correctly now. Any thoughts? I appreciate the help.
-
Ok. I understand that. When I make the changes, VS gives me; Error 11 The best overloaded method match for 'RenderingManager.AddToPostDrawQueue(int, Callback)' has some invalid arguments Error 12 Argument 2: cannot convert from 'CallBack' to 'Callback' I believe Error 11 is being caused by Error 12 but unless there is a reserved keyword type called Callback, Error 12 doesn't make much sense to me. All I can think of is that Callback in .RenderingManager.AddToPostDrawQueue(int, Callback) is not a delegate at all. However, I can find no other reference in Unity nor C# man pages to callbacks except in delegates. Any ideas or wisdom? Thanks again.
-
Hello, I am trying to create a new GUI window in KSP but when I call my delegate callback(onDraw()), VS errors on me. I've looked at the API pages, Unity tutorials and C# pages for help but have been unable to fix the issues. I'd greatly appreciate any help. Below is my code along with the VS errors. namespace KSP_Wind_Display { // public delegate CallBack(int que, int onDraw); public class Wind_GUI_Display : PartModule { delegate void CallBack(); CallBack callback; private Rect window_pos = new Rect(); // New blank window private GUIStyle window_style, label_style; private bool has_init_styles = false; public override void OnStart(PartModule.StartState state) { base.OnStart(state); if (!has_init_styles) init_styles(); callback += onDraw; if (state != StartState.Editor) RenderingManager.AddToPostDrawQueue(0, callback()); <-------- Error 11 The best overloaded method match for 'RenderingManager.AddToPostDrawQueue(int, Callback)' has some invalid arguments <-------- Error 12 Argument 2: cannot convert from 'void' to 'Callback' } private void onDraw() // Start up the window { if (this.vessel == FlightGlobals.ActiveVessel) window_pos = GUILayout.Window(10, window_pos, onWindow, "Wind Direction", window_style); } private void onWindow(int window_id) { GUILayout.BeginHorizontal(); GUILayout.EndHorizontal(); GUI.DragWindow(); // Makes the window draggable } private void init_styles() { window_style = new GUIStyle(HighLogic.Skin.window); window_style.fixedWidth = 250.0f; label_style = new GUIStyle(HighLogic.Skin.label); label_style.stretchWidth = true; // stretches the label width to that of the window has_init_styles = true; } } } For Error 11, shouldn't that result in onDraw() being called. AddToPostDrawQueue needs an int and Callback as it's arguments and that's what I am passing it. For Error 12, I suspect the problem is where I first declare Callback to be void type of delegate. However, I don't want Callback to return anything so it has to be a void. I've worked at this problem for about 2 hours now and online forums, tutorials and man pages haven't help. Can someone here spot the issue and at least give me a hint on how to resolve it? Thanks in advance.
-
Hello, I am new to modding KSP and I am having some difficulty that maybe the community could help me with. I am trying to get the surface area of the vehicle but to do that, I either need the activevessel radius (in cases of simple rockets) or a List<Part> parts that contains the current parts attached to the vehicle that I could then use to make an educated guess as to the vessels radius (for more complex ships as 3 Rockomax Jumbo-64 Fuel Tanks are likely to be side by side, etc.). Right now, I am assuming rockets can be approximated by cylinders as getting the true shape of the vessel would be a nightmare. The problem is that when looking through the API calls, I don't see anything references to vessel radius and when I call; public List<Part> parts_ = new List<Part>(); parts_ = FlightGlobals.ActiveVessel.GetActiveParts(); Debug.Log("\n\nVessel parts are: " + craft.parts + "\n\n"); Visual Studio errors on: Error 11 The type 'UnityEngine.EventSystems.IPointerClickHandler' is defined in an assembly that is not referenced. You must add a reference to assembly 'UnityEngine.UI, Version=1.0.0.0, Culture=neutral, PublicKeyToken=null'. c:\users\joshua\documents\visual studio 2013\Projects\KSP_Wind\KSP_Wind\Class1.cs 92 27 KSP_Wind I am not using any GUI options and my includes are: using System; using System.Collections; using System.Collections.Generic; using UnityEngine; using KSP; I know that the error is coming from <Part> because when I change it to public List<String> parts_ = new List<String>(); I no longer get that error. (I know I'll get another because String is incompatible with FlightGlobals.ActiveVessel.GetActiveParts();). I am unsure how to correct this issue nor am I even sure that I am on the correct track on how to either get the vessel radius or the list of currently attached parts. I greatly appreciate any help you can give. Thanks.