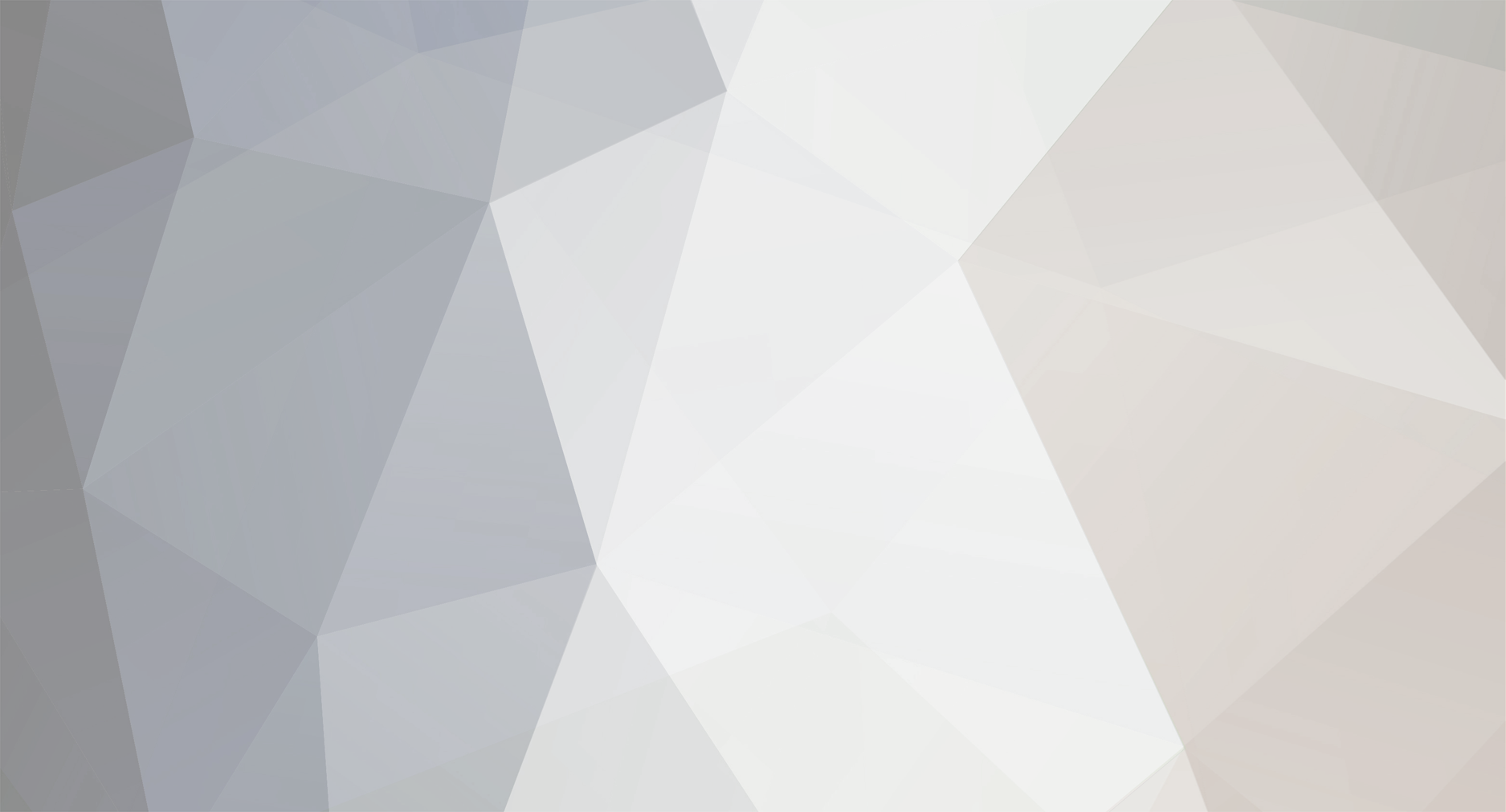
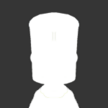
fatcargo
Members-
Posts
400 -
Joined
-
Last visited
Content Type
Profiles
Forums
Developer Articles
KSP2 Release Notes
Everything posted by fatcargo
-
PopupDialog and the DialogGUI classes
fatcargo replied to DMagic's topic in KSP1 C# Plugin Development Help and Support
Thanks ! I suspected this might be the case, which leaves DialogGUIBox() in questionable usability - if used on it's own, its functionality could have been duplicated with label with no text for example. Then again, it could be similar to DialogGUISpace(), just with added box for visibility. It is odd that it accepts any UI elements at all . -
PopupDialog and the DialogGUI classes
fatcargo replied to DMagic's topic in KSP1 C# Plugin Development Help and Support
I should consider this topic "official" discussion for DialogGUI, though i did separate posts and i'm willing to change to this topic only to keep all related problems and solutions in one spot (even though this is offically "necroposting"). So, i've got the problem with DialogGUIBox. I think this element is used for grouping other basic UI elements such as labels, buttons, sliders etc... Assuming above is correct, i can't properly set positions of UI elements inside box. When i add two labels, they appear waaay in upper left corner, far outside popupdialog window, and label text is vertically aligned. I tried placing box inside horizontal layout, tried to add content sizer as first element... nothing helps. So, does anyone have a working example of using box as container for other elements (again, if that is a proper use of box) ? Maybe a dumbed-down-hello-world style example ? -
Solved it ! My custom UISkinDef style was incomplete ! Here is what is needed to specifically customize scrollview. When i first started tinkering with styles, i ignored these fields since i already had slider-related fields, i never associated scrollbar stuff with scrollview. Thus the point of confusion. CAMSkin.verticalScrollbarUpButton = buttonStyle; CAMSkin.verticalScrollbarThumb = buttonStyle; CAMSkin.verticalScrollbarDownButton = buttonStyle; CAMSkin.verticalScrollbar = windowStyle; CAMSkin.horizontalScrollbarThumb = buttonStyle; CAMSkin.horizontalScrollbarRightButton = buttonStyle; CAMSkin.horizontalScrollbarLeftButton = buttonStyle; CAMSkin.horizontalScrollbar = windowStyle; This raises an interesting issue : when creating a new style, not all fields are initialized to values from HighLogic.UISkin (and it should, as fallback/default) , which looks odd to me.
-
Thanks for the idea ! I'm not really after the class members listing, but their VALUES. So i did some digging and found a dump-values-of-all-members-inside-class over on stackoverflow. What i did was -write that function -assign a HighLogic.UISkin.scrollView to a public variable -set a convenient breakpoint -then compile/copy dll/run ksp -run debugger, run into breakpoint -in immediate window i called that function and got all values This way i can dissect how that (working) UISkinDef class is setup so i can clone it and try to modify until it looks that way i want to (or until it breaks ). Though now when i look at values my SolidColorFill() may be not correct. Here is some more code. This is what i use to create backgrounds/fills for various UI elements. public Sprite SolidColorFill(Color color) { Texture2D tex = new Texture2D(1, 1, TextureFormat.ARGB32, false); tex.SetPixel(1, 1, color); tex.Apply(); return Sprite.Create( tex, new Rect(0, 0, tex.width, tex.height), new Vector2(0.5f, 0.5f), tex.width ); } As it can be seen, it is copy from github sources, two functions mushed into one. And this is a dump from "HighLogic.UISkin.scrollView" (some editing done to make it more legible): //Type: UIStyle //Fields: System.String name = scrollview UIStyleState normal = UIStyleState UIStyleState active = UIStyleState UIStyleState highlight = UIStyleState UIStyleState disabled = UIStyleState UnityEngine.Font font = System.Int32 fontSize = 0 UnityEngine.FontStyle fontStyle = Normal System.Boolean wordWrap = False System.Boolean richText = True UnityEngine.TextAnchor alignment = UpperLeft UnityEngine.TextClipping clipping = Clip System.Single lineHeight = 13 System.Boolean stretchHeight = False System.Boolean stretchWidth = True System.Single fixedHeight = 0 System.Single fixedWidth = 0 //Type: UIStyleState //Fields: UnityEngine.Sprite background = rect_round_down_dark_veryTransparent //(UnityEngine.Sprite) UnityEngine.Color textColor = RGBA(0.000, 0.000, 0.000, 1.000) The function used to dump data is from C# Variable description function . I have removed some of the "\r" carriage returns. Note that when this is used in immediate window, it will escape \n newlines before printing, so it won't be a newline-formatted printout, rather a unbroken string. But it still beats manually exploring all members and doing copy/paste into text editor. public static string DisplayObjectInfo(System.Object o) { StringBuilder sb = new StringBuilder(); // Include the type of the object System.Type type = o.GetType(); sb.Append("Type: " + type.Name); // Include information for each Field sb.Append('\n' + "Fields:"); System.Reflection.FieldInfo[] fi = type.GetFields(); if (fi.Length > 0) { foreach (FieldInfo f in fi) { sb.Append('\n' + " " + f.ToString() + " = " + f.GetValue(o)); } } else sb.Append('\n' + " None"); // Include information for each Property sb.Append('\n'+ "Properties:"); System.Reflection.PropertyInfo[] pi = type.GetProperties(); if (pi.Length > 0) { foreach (PropertyInfo p in pi) { sb.Append('\n' + " " + p.ToString() + " = " + p.GetValue(o, null)); } } else sb.Append('\n' + " None"); return sb.ToString(); }
-
I am trying to make a UI for my partmodule using DialogGUI and need to fit a long list of UI elements inside window. Sorry to bug people but im paging @HebaruSan, @DMagic, @MOARdV, @malkuth and others. If anyone has some time please respond. DialogGUIScrollList (and a mandatory DialogGUIContentSizer) are used for this. When opening a window, scrollbar of right side of scroll view has white thumb and a white body. The scroll view content itself also appears to have default style (grayish box with round corners). To me it appears that scroll view has no style defined, so some of its elements either revert to hard-coded fail-safe defaults or take values from builtin style (HighLogic.UISkin). Is this a problem with my style ? Here is what i used to set my UISkinDef.scrollView. Please note that some values may by wildly off - its on purpose, for testing only. //note that i did not used static class to define/initialize variables and methods, //because i've had NREs when trying to use styles from static class on slider UI elements scrollViewStyleState.background = SolidColorFill(Color.yellow); scrollViewStyleState.textColor = Color.red; scrollViewStyle.fixedHeight = 1f; scrollViewStyle.stretchWidth = true; scrollViewStyle.stretchHeight = true; scrollViewStyle.lineHeight = 20f; scrollViewStyle.clipping = TextClipping.Clip; scrollViewStyle.alignment = TextAnchor.MiddleCenter; scrollViewStyle.richText = false; scrollViewStyle.wordWrap = false; scrollViewStyle.fontStyle = FontStyle.Normal; scrollViewStyle.fontSize = 20; scrollViewStyle.font = UISkinManager.defaultSkin.font; scrollViewStyle.disabled = scrollViewStyleState; scrollViewStyle.highlight = scrollViewStyleState; scrollViewStyle.active = scrollViewStyleState; scrollViewStyle.normal = scrollViewStyleState; scrollViewStyle.fixedWidth = 20f; //MultiOptionDialog is supplied with this new DialogGUIVerticalLayout( new DialogGUIScrollList(new Vector2(400f, 150f), new Vector2(350f, 140f), false, true, new DialogGUIVerticalLayout( new DialogGUIContentSizer(UnityEngine.UI.ContentSizeFitter.FitMode.Unconstrained, UnityEngine.UI.ContentSizeFitter.FitMode.PreferredSize, true), //UI elements such as labels, sliders, buttons etc new DialogGUILabel("label test"), new DialogGUILabel("label test"), new DialogGUILabel("label test"), new DialogGUILabel("label test"), new DialogGUILabel("label test"), new DialogGUILabel("label test"), new DialogGUILabel("label test"), new DialogGUILabel("label test"), new DialogGUILabel("label test"), new DialogGUILabel("label test") )//vertical layout - scroll )//scroll list )// vertical layout - main I don't know which of these is wrong, though i'm concerned that one-style-covers-all approach to using same styling structure for all types of UI elements may be too rough (for example what effect would text color have on scrollview ?)
-
Does "PAW" refer to Part Action Window Sorter-Outer ? While i tinkered with my code, i used following flags (this was done with stock part menus) : guiActive = true guiActiveEditor = true externalToEVAOnly = false guiActiveUnfocused = true //this one ? unfocusedRange = 1 //not directly relevant, though it wouldn't surprise me if KSP freaks out on large values Though i tested this by launching two probe cores separated with docking ports and batteries, then cheating them into orbit and then decoupling them. As distance grew, beyond 1m range, menu item for other vessel vanished. Note that distance is measured from part's stack attachment node (most likely first one in part.cfg, part i work with has only one).
-
Is it possible to create a UI asset bundle and use it so KSP plugin can add new UI elements at run-time (maybe copy existing UI element and adding it into existing hierarchy) ? So far, none of the tutorials mention such a possibility. Asset bundles appear to be static constructs. In my case, i will need arbitrary number of labels and sliders in vertical scrollable list.
-
[1.10.0] AnimatedAttachment 2.1.5 (2020-07-15)
fatcargo replied to Katten's topic in KSP1 Mod Releases
Crafts in orbits can have two things in common : they can be not yet loaded (ie can be seen from KSC tracking station) and "under the hood" vessels in orbit (under fast warp, no accelerations from atmosphere or engines) have their physics turned off. To me it sounds like issue with unloaded vessels. -
[1.10.0] AnimatedAttachment 2.1.5 (2020-07-15)
fatcargo replied to Katten's topic in KSP1 Mod Releases
B9 Proc Wings have flaps/ailerons and procedural tanks/SRBs seem not to have anything animated (i tried to look for gimballed engines but found nothing). What is more important : in SPH/VAB procedural parts change shape and size while editing, does AA capture these events ? Even stock parts with variant switching may pose a challenge (oh and by the way do TweakScaled parts work OK too ?). It was showcased how various part can be attached and animated on flaps surfaces B9 PWings popped into my mind. One other test : do RCS and RCSFX parts properly update their thrust vectors when moved into new positions ? Did'nt see it in yt video... Another possible issue is with Kerbal Foundries wheels/tracks : few KSP versions back (old KF before unity wheel collider breakdown) i had trouble with KF wheels mounted on IR parts. Back then, KF wheels would in flight scene assume direction/steering on vessel load and after deployment via IR they did not work properly. This time i hope AA deployed KF wheels should not suffer from this (now they have different direction/steering setup). -
[1.10.0] AnimatedAttachment 2.1.5 (2020-07-15)
fatcargo replied to Katten's topic in KSP1 Mod Releases
Got this from post on IR Next thread. You did not just save my life .. you saved everyone ! Lately i don't have much time for playing KSP (though i am trying to make finish a mod, old and long story...) but can anyone test for compatibility with procedural mods like b9 proc wings and/or procedural parts (tanks ands SRBs) ? -
I've tried also with alternate PlayerConnectionConfigFile generated on my system (which is kind of paradox since locally generated file is actually original, but i'm splitting hairs here ) and no luck. I then renamed my KSP debug player to "Unity.exe" (and added .pdb file from same editor install location where i copied debug player), made new link to folder and it (again) worked without a hitch. When i was googling around i found some post over on unity forums that unity debugger for VS needed to find "Unity.exe" process. Ehhh i guess this works too. If both debug player and editor are run at the same time, you can tell the difference because editor will have project name in list of debug listeners, ksp debug player will have that entry empty. Finally, if above does not work you can resort to listing all processes in system that have open listen ports (two TCP and one UDP, usually the second TCP is one you should connect to). Then open VS unity debugger and choose "Input IP" and enter 127.0.0.1:PORT. Problem is (at least on my machine) that every time ksp debug player is run, PORT number changes Oh and word of caution : i just noticed that VS unity debugger sees KSP debug player as "Editor". I don't know if it matters, but i tried inserting a breakpoint and it seems to work.
-
UPDATE: i managed to make desired (minimal size) installation just for plugin development. First i used this to download required packages : vs_Community.exe --layout c:\vs2017offline --passive --wait --add Microsoft.VisualStudio.Workload.ManagedDesktop --add Microsoft.Net.Component.3.5.DeveloperTools --add Microsoft.Net.Component.4.7.1.TargetingPack --add Microsoft.VisualStudio.Component.NuGet --add Microsoft.VisualStudio.Component.Roslyn.Compiler --add Microsoft.VisualStudio.Component.Roslyn.LanguageServices --add Microsoft.VisualStudio.Component.Static.Analysis.Tools --add Microsoft.VisualStudio.Component.Unity Above will load everything EXCEPT Unity3D package, i downloaded it separately. Command line will cause IDE to show so download progress will be visible. To install (similar command line) , run : PUSHD C:\vs2017offline vs_setup.exe --passive --norestart --wait --add Microsoft.VisualStudio.Workload.ManagedDesktop --add Microsoft.Net.Component.3.5.DeveloperTools --add Microsoft.Net.Component.4.7.1.TargetingPack --add Microsoft.VisualStudio.Component.NuGet --add Microsoft.VisualStudio.Component.Roslyn.Compiler --add Microsoft.VisualStudio.Component.Roslyn.LanguageServices --add Microsoft.VisualStudio.Component.Static.Analysis.Tools --add Microsoft.VisualStudio.Component.Unity POPD Note that Unity3D package can be included entirely (shortening the "--add" packages command line) to just two entries. Also, above install command line supresses reboot, it may be needed to reboot after installing.
-
Debugging update on Windows for : KSP 1.4.3 build 2152 Unity3D 2017.1.3p1 Visual Studio 2017 v15 Original Instructions are mostly OK except for : - debug version of Unity player needs to be named "Unity.exe" (see notes below) - mklink command now needs to be "mklink /J Unity_Data KSP_x64_Data" (case insensitive, see notes below) [NOTES] If debug player is not named "Unity.exe", unity addon will not recognize it and will present empty "Select Unity Instance" window, offering network debugging via "Input IP" option where it needs both IP address and PORT. For IP address its simple, if VS debugger and game are on same machine but, there will be problem with PORT since (at least in my case) debug player changes port with each game run. Debug player is looking for Data folder named as "NameOfEXE_Data". [/NOTES] Note also that because KSP 1.4.x now runs on new version of Unity3D, old debugger addons no longer work (at least in my case, your expirience may differ) for example Xamarin or older VS2015. ... and yes yes i know i necropost, but to me this is "official" thread about debugging, and this is where i first got a working debug. I felt it needed updating. Paging dr. @sarbian please as time permits to update OP. Thanks in advance !
-
It's been quite a while (almost a year) since i last did some coding in KSP. To cut the story short : i tried searching and it didn't help. I guess my case is a little specific. When i start my VS 2017 Community and look at offered project templates, there is no "Class Library (.NET Framework)" i need to make a plugin. Note that my VS 2017 install is running purely from OFFLINE installer created using vs_community.exe as so : vs_community.exe --layout c:\vs2017offline --add [one.of.many.packages] Note that i used multiple --add command line arguments and that running the above does download packages. What i'm having issue with is that i can't guess which package(s) will cause IDE to show "Class Library (.NET Framework)" template. If i load old project (which i want to avoid using as "template") i can edit project preferences and i can see "class library" option. If i choose "blank project" template, project preferences are not available. Online/download from IDE is not an option since i want to save on network usage on any subsequent reinstalls, so i want a single download of all required packages and then actual install on target machine. Note also that due to KSP being switched to Unity 2017 and me needing to have debugging facilities (Tools for Unity VS2017 package) forced me to abandon VS2015 (which did not suffer from the above problem). References: Create and offline installation folder @ MS Visual Studio Community 2017 component directory @ MS, i used this in conjuction with UI for selecting workloads and then to figure out what packages i need (so far i did not see option to create local copy of packages from UI as i can with command line). Currently, i'm trying to download whatever is missing from VS installer UI, hoping to identify missing packages...
-
[Minimum KSP version - 1.11] Kerbal Attachment System (KAS) v1.12
fatcargo replied to IgorZ's topic in KSP1 Mod Releases
Oh and one more thing ... I don't know if this was reported before : when pulling extremely heavy payloads, craft tends to go all wonky and Kraken messes it up. I made a "submarine" (to be precise a Bathysphere) that uses IW-50 winch attached to four USI floats and USI Otter impeller . Main lander can that houses a scientist and scientific instrumentation are linked below winch. Negative buoyancy is achieved using Jatwaa's MOIST!UWT mod (added intake and a fuel tank at very bottom). Problem is that UWT mod introduces CompressedLiquid resource which is VERY heavy and only a tiny amount is required. If not careful, one can easily add 100 tonnes in no time at all. Lowering the pod is not a problem, but as soon as i tried to raise it, Kraken messed up the platform and bugged the winch, freezing it at about 20m of cable length claiming it can't retract because of blockage. I started with a probe core and build from there. Build was kind of in reverse because the manned lander can is attached to winch. I did this since i needed to maintain view focus on the pod, not the platform. Anyway the pics -
[Minimum KSP version - 1.11] Kerbal Attachment System (KAS) v1.12
fatcargo replied to IgorZ's topic in KSP1 Mod Releases
I've created a new github issue for two more-less minor enhacements : 1. Adding "stop" action hroup for winches (haven;t found any in SPH/VAB) 2. Adding winch part with centered cable for vertically mass-aligned payloads -
Feature request (or separate plugin) : manually bind craft to particular contract ? If there is such feature or plugin please direct me to it. Quite often i have multiple long term DMOS missions and i guess trying to sort them is part of "mission management experience" so i also ask if this makes sense or not. Currently, i name my crafts with agency/body/instrumentation/orbit parameters and then manually match them against contract list in CapCom.
-
And a side note for future reference : i just remembered the Sequencer for IR. It is ok as it is, but it got me wondering : would it be possible to create IR-driven compound part (basically a pre-built assembly) that contains Sequencer cfg ? Such part would be pre-programmed and built either by a part creator or player. Individual IR-parts would not be accessible in-game, only the whole assembly and only with predefined Sequencer input controls. This, combined with IR parts non-collision should allow for mechanically believable assemblies. Does any of this appeal (or even makes sense) to anyone ?
-
@jrodriguez Being the one to update B9 for 1.4.2 (big thanks !), i'm asking if this plugin supports anything with more complex geometry than planar surfaces like boxes, tubes or hollow spaces enclosed with multiple colliders ? The UI B9 Pwings offers is something i've never seen in any other plugin, so at least i'm willing to learn more about it before spending time making models.
-
[1.8.x] Kerbal Foundries -- Continued - Tracks, Wheels, and Gear
fatcargo replied to Shadowmage's topic in KSP1 Mod Releases
thanks for pointing out the direction helper, now if only "turn" indicator would get added too ... Though i have to stress that doing "global" control via KF toolbar icon (stock/blizzy/etc...) would be better. Activate, look at wheels, tweak, turn off. One button to run them all A side note : is there a modeling tut for KF wheels/tracks ? Something with vanilla models in fbx that i can import and and have a look at ? -
[1.8.x] Kerbal Foundries -- Continued - Tracks, Wheels, and Gear
fatcargo replied to Shadowmage's topic in KSP1 Mod Releases
Feature request : Would it be possible to simulate direction and drive of KF wheels while still in SPH/VAB editor ? Some kind of "simulate" button that activates all wheels in editor (if not stowed, with slowed down drive rotation so player sees if direction is ok). Similar to Infernal Robotics Servo Control. That way player can see how wheels / tracks turn, so tweaking wheels does not become editor - flight - editor - flight-... testing we have now. -
Feature request : i usually setup my experiments to run at once from action groups. As per usual, window for collected ALL data pops up offering the usual options + DMOS xmit so i can list and act on all of them. So far so good. However, once this window is closed, i can only either transmit all science (which available battery storage may not allow for) or select each science part and select "review" option to send data specifically for that experiment. So in short : @DMagic , can you add option to open AGAIN this data review window that lists ALL experiments ? It could be available as part menu option on any of science parts. Aaand i just realized it could be a separate plugin.
-
Thanks for the useful info ! RE point 2 : i was thinking along the lines of having custom motion parts that do not require rewriting IR. That way alternate complex/unusual joint groups can be added.